Question
A particular talents competition has 5 judges, each of whom award a score between 0 and 10 to each performer. Fractional scores, such as 8.3
A particular talents competition has 5 judges, each of whom award a score between 0 and 10 to each performer.
Fractional scores, such as 8.3 are allowed. A performer’s final score is determined by dropping the highest and lowest score received, then averaging the 3 remaining scores. Write a program that uses this method to calculate a contestant’s score. It should include the following functions: void getJudgeData() should ask the user for a judge’s score, store it in a reference parameter variable, and validate it. This function should be called by main once for each of the 5 judges. void calcScore() should calculate and display the average of the 3 scores that remain after dropping the highest and lowest scores the performer received. This function should be called just once by main, and should be passed the 5 scores.
The last two functions, described below, should be called by calcScore, which uses the returnedinformation todetermine which of the scores to drop.
• int findLowest()should find and return the lowest of the 5 scores passed to it.
• int findHighest()should find and return the highest of the 5 scores passed to it.
Input Validation: Do not accept judge scores lower than 0 or higher than 10.
You may use more functions, but you must use at least the four listed above. Here are the prototypes:
// Function prototypes
void getJudgeData(double &);
void calcScore(double, double, double, double, double);
double findLowest(double, double, double, double, double);
double findHighest(double, double, double, double, double);
- Compile your work frequently to avoid too many errors at one time.
- Verify the parameter lists in the prototype, the call, and the header for each function.
- You can call a function from inside another function.
Program requirements and/or constraints:
• No global variables are allowed!
• program must work for any valid input and not just the values shown in the sample output.
• Don't use material beyond the current topic
Sample run: User input is to the right of the colon or parenthesis:
Enter score between 0 and 10: 8
Enter score between 0 and 10: 7.5
Enter score between 0 and 10: 10
Enter score between 0 and 10: 8
Enter score between 0 and 10: 5
This contestant's talent score is: 7.83
Do you want to enter another set of scores? (y/n)y
Enter score between 0 and 10: 8
Enter score between 0 and 10: 8
Enter score between 0 and 10: 1
Enter score between 0 and 10: 8
Enter score between 0 and 10: 10
This contestant's talent score is: 8.00
Do you want to enter another set of scores? (y/n)y
Enter score between 0 and 10: 8.5
Enter score between 0 and 10: 7.6
Enter score between 0 and 10: 5.5
Enter score between 0 and 10: 9.4
Enter score between 0 and 10: 6
This contestant's talent score is: 7.37
Do you want to enter another set of scores? (y/n)y
Enter score between 0 and 10: 8
Enter score between 0 and 10: 11
Score must be in the range 0 - 10. Please re-enter score: 10
Enter score between 0 and 10: 15
Score must be in the range 0 - 10. Please re-enter score: 15
Score must be in the range 0 - 10. Please re-enter score: 7
Enter score between 0 and 10: 8
Enter score between 0 and 10: 9This contestant's talent score is: 8.33
Do you want to enter another set of scores? (y/n)n
Step by Step Solution
There are 3 Steps involved in it
Step: 1
include include using namespace std void getJudgeDatadouble ...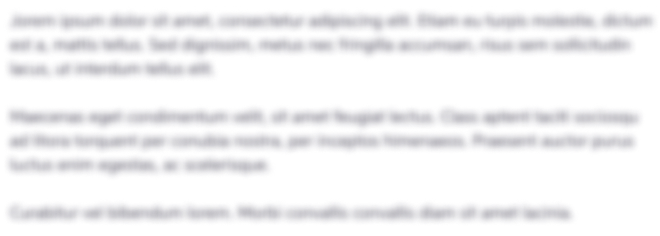
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Document Format ( 2 attachments)
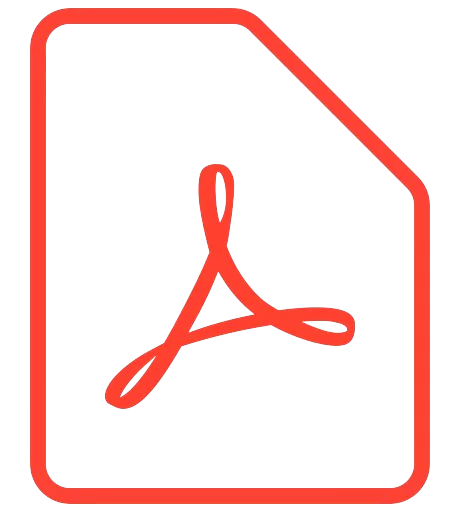
609474c272067_24864.pdf
180 KBs PDF File
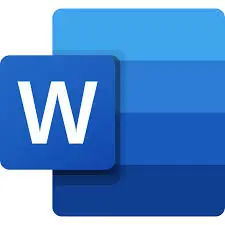
609474c272067_24864.docx
120 KBs Word File
Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started