Question
Create an inheritance hierarchy that a bank might use to represent customers bank accounts. All customers can deposit (credit) into their accounts and withdraw (debit)
Create an inheritance hierarchy that a bank might use to represent customers’ bank accounts. All customers can deposit (“credit”) into their accounts and withdraw (“debit”) from their accounts. More specific types of accounts also exist. Savings accounts, for instance, earn interest on the money they hold. Checking accounts, on the other hand, can charge a fee per transaction. Create an inheritance hierarchy containing base class Account and derived classes SavingsAccount and CheckingAccount that inherit from Account.
Deliverables
•A driver program (driver.cpp)
•An implementation of Account class (account.h, account.cpp)
•An implementation of SavingsAccount (savingsaccount.h, savingsaccount.cpp)
•An implementation of CheckingAccount (checkingaccount.h, checkingaccount.cpp)
1 Account class
Implement the base class.
1.1 Data Members
balance (double)
1.2 Member Functions
Constructors
Defines default and paramaterized constructors. Confirm balance not below zero. If so, set to zero and , output an error message.
Destructor
Defines destructor. Clean up any allocated memory.
Accessors
double getBalance()
Mutators
void debit(amount) Withdraws from the account if the amount does not exceed the balance. If so, nothing is debited and an error message is printed. This method may change when you implement the derived class.
void credit(amount) Deposits into the account.
2 SavingsAccount class
Implement the derived class.
2.1 Data Members
interestRate (double)
2.2 Member Functions
Constructors
Defines default and parameterized constructor. Confirm interestRate not below zero. If so, set to zero and output an error message.
Destructor
Defines destructor. Clean up any allocated memory.
Accessors
double calcInterest() Returns the current interest of the account (balance multiplied by interest rate).
3 CheckingAccount class
Implement the derived class.
3.1 Data Members
feeForTransaction (double)
3.2 Member Functions
Constructors
Defines default and parameterized constructor. Confirm feeForTransaction not below zero. If so, set to zero and output an error message.
Destructor
Defines destructor. Clean up any allocated memory.
Mutators
void debit(amount) Invokes the base class debit member function and substracts the fee if successful debit (Hint: redefine parent method to return a value you can check).
void credit(amount) Invokes the base class credit member function and subtracts the fee.
4 The Driver Program
In your driver, create a vector of six Account pointers (alternating Checking then Saving, etc.) For each account, allow the user to deposit and withdraw from the account using the appropriate member functions. As you process each account, determine its type. If it is a SavingsAccount, calculate and add interest after the deposit. After processing each account, print the updated balance by invoking the base-class member function getBalance.
4.1 Example output:
Account 1 balance: $70.00
Enter an amount to withdraw from Account 1: 80.00
Debit amount exceeded account balance.
Enter an amount to deposit into Account 1: 35.00
$1.00 transaction fee charged.
Updated Account 1 balance: $104.00
Account 2 balance: $80.00
Enter an amount to withdraw from Account 2: 10.00
Enter an amount to deposit into Account 2: 35.00
Adding $5.00 interest to Account 2 (a SavingsAccount)
Updated Account 2 balance: $110.00
(repeat for Accounts 3 through 6)
Step by Step Solution
3.45 Rating (152 Votes )
There are 3 Steps involved in it
Step: 1
copyable code maincpp include file include header file include standard input using namespace std include Account1h include SaveAccounth include Check...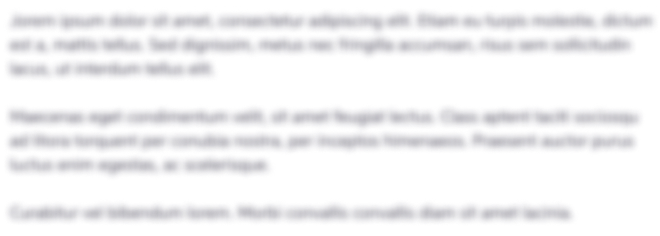
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Document Format ( 2 attachments)
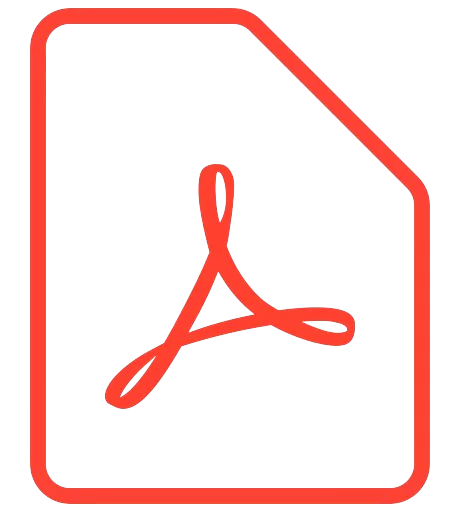
6093e6b0e5c63_24283.pdf
180 KBs PDF File
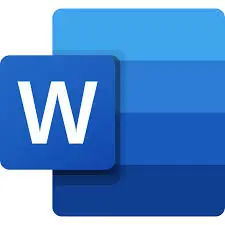
6093e6b0e5c63_24283.docx
120 KBs Word File
Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started