Question
1. (25 pts) Implement the insert method for binary search trees. Do not insert duplicate values. 2. (15 pts) Implement the search method for binary
1. (25 pts) Implement the insert method for binary search trees. Do not insert duplicate values. 2. (15 pts) Implement the search method for binary search trees. 3. (15 pts) Implement isBST, a method for checking whether or not a tree is a binary search tree. 4. (10 pts) Implement the pre-order traversal method for binary search trees.
All of this should be implemented in the below code, in C++, without changing any of the parameters or functions as they are laid out.
#include
BST::BST(){ }
BST::~BST(){}
std::shared_ptr
std::shared_ptr
std::shared_ptr
std::shared_ptr
std::shared_ptr
std::shared_ptr
void BST::insertValue(int val){ }
std::shared_ptr
void BST::deleteValue(int val){ }
std::shared_ptr
bool BST::isBST(std::shared_ptr
bool BST::isBST(std::shared_ptr
void BST::preOrder(std::shared_ptr
void BST::inOrder(std::shared_ptr
void BST::postOrder(std::shared_ptr
The code above is meant to be grouped together with the below code:
BST.h:
// CSCI 311 - Spring 2023 // Lab 3 BST header // Author: Carter Tillquist
#ifndef BST_H #define BST_H
#include
class BST{ public: std::shared_ptr
BST(); ~BST();
std::shared_ptr
std::shared_ptr
void insertValue(int); std::shared_ptr
bool isBST(std::shared_ptr
#endif
Node.cpp:
// CSCI 311 - Spring 2023 // Node Class // Author: Carter Tillquist
#include "Node.h"
Node::Node(){ value = 0; left = nullptr; right = nullptr; }
Node::Node(int v){ value = v; left = nullptr; right = nullptr; }
Node::~Node(){}
Node.h
// CSCI 311 - Spring 2023 // Node Header // Author: Carter Tillquist
#ifndef NODE_H #define NODE_H
#include
class Node{ public: int value; Node* left; Node* right; Node* data; Node(); Node(int); ~Node(); };
#endif
BSTDriver.cpp
// CSCI 311 - Spring 2023 // Lab 3 - BST Driver // Author: Carter Tillquist
#include
void printVector(const vector
/************************************************** * Simple main to test Binary Search Tree methods * * ************************************************/ int main() { std::shared_ptr
int operation; cin >> operation;
while (operation > 0) { vector
return 0; }
/***************************************************************** * Print the values of nodes in a vector * * v - const vector
/******************************************************************************************* * Given a BST, get a value to search for from the console and apply the BST search method * * T - std::shared_ptr
/******************************************************************** * Given a BST, get a value from the console and add it to the tree * * T - std::shared_ptr
/************************************************************************************** * Given a BST, get a value from the console and remove it from the tree if it exists * * T - std::shared_ptr
Step by Step Solution
There are 3 Steps involved in it
Step: 1
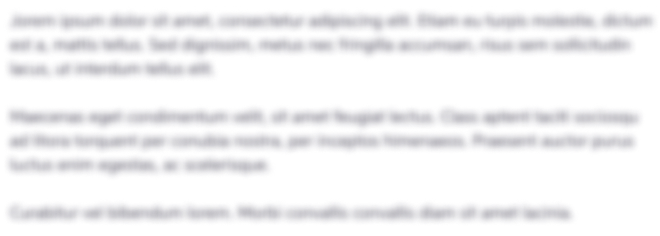
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started