Question
1. code needs to be written c++ 2. Recode the roster example we studied in class such that it prints the list of classes
2. Recode the "roster"example we studied in class such that it prints the list of classes each student is enrolled in. Here is an example printout
cs1.txt:
Mary Price
Stephanie Watson
Rachel Rivera
Raymond Powell
Catherine Ward
Doris Howard
Cynthia Jenkins
Betty Torres
Juan Cox
Kathleen Anderson
Mark Russell
Gerald Edwards
Mikhail Nesterenko
Charles Martin
Linda Johnson
David Collins
Elizabeth Jones
Jonathan Baker
Maria Kelly
Martin Taylor
Dennis White
Brian Sanchez
cs2.txt.:
Joyce Rodriguez
Sharon Bennett
John Jenkins
Gerald Edwards
Rachel Peterson
Marilyn Phillips
Catherine Morgan
Lori Walker
Julie Perry
Peter Young
Peter Hall
Mark Russell
Linda Johnson
Dennis White
cs3.txt:
Mikhail Nesterenko
Catherine Powell
Anne Clark
Tina Ward
Gerald Edwards
Andrew Turner
Peter Hall
Jason Reed
Kathleen Anderson
Alan Wood
Elizabeth Green
Louis Harris
Cynthia Jenkins
Peter Young
Lori Walker
cs4.txt
Peter Hall
Gerald Edwards
Mark Russell
Anne Clark
Judy Miller
Nicholas Peterson
Elizabeth Green
Brian Sanchez
Juan Cox
Craig Jackson
Louis Gray
Maria Kelly
drop out.txt
Mikhail Nesterenko
Catherine Powell
Anne Clark
Sharon Bennett
Jason Reed
Raymond Powell
Catherine Ward
Alan Wood
Dennis White
- roster.cpp vector and list algorithms Mikhail Nesterenko 3/11/2014
// vector and list algorithms
// Mikhail Nesterenko
// 3/11/2014
#include
#include
#include
#include
#include
#include
using std::ifstream;
using std::string; using std::getline;
using std::list; using std::vector;
using std::cout; using std::endl;
using std::move;
// reading a list from a fileName
void readRoster(list& roster, string fileName);
// printing a list out
void printRoster(const list& roster);
int main(int argc, char* argv[]){
if (argc =>
cout
}
// vector of courses of students
vector > courseStudents;
for(int i=1; i
list roster;
readRoster(roster, argv[i]);
cout
printRoster(roster);
courseStudents.push_back(move(roster));
}
// reading in dropouts
list dropouts;
readRoster(dropouts, argv[argc-1]);
cout
// master list of students
list allStudents;
for(auto& lst : courseStudents)
allStudents.splice(allStudents.end(), lst);
cout
printRoster(allStudents);
// sorting master list
allStudents.sort();
cout
printRoster(allStudents);
// eliminating duplicates
allStudents.unique();
cout
printRoster(allStudents);
// removing individual dropouts
for (const auto& str : dropouts)
allStudents.remove(str);
cout
printRoster(allStudents);
}
// reading in a file of names into a list of strings
void readRoster(list& roster, string fileName){
ifstream course(fileName);
string first, last;
while(course >> first >> last)
roster.push_back(move(first + ' ' + last));
course.close();
}
// printing a list out
void printRoster(const list& roster){
for(const auto& str : roster)
cout
}
- rosterObject.cpp vector and list algorithms with objects in containers Mikhail Nesterenko 8/11/2018
#include
#include
#include
#include
#include
#include
using std::ifstream;
using std::string; using std::getline;
using std::list; using std::vector;
using std::cout; using std::endl;
using std::move;
class Student{
public:
Student(string firstName, string lastName):
firstName_(firstName), lastName_(lastName) {}
// move constructor, not really needed, generated automatically
Student(Student && org):
firstName_(move(org.firstName_)),
lastName_(move(org.lastName_))
{}
// force generation of default copy constructor
Student(const Student & org) = default;
string print() const {return firstName_ + ' ' + lastName_;}
// needed for unique() and for remove()
friend bool operator== (Student left, Student right){
return left.lastName_ == right.lastName_ &&
left.firstName_ == right.firstName_;
}
// needed for sort()
friend bool operator
return left.lastName_
(left.lastName_ == right.lastName_ &&
left.firstName_
}
private:
string firstName_;
string lastName_;
};
// reading a list from a fileName
void readRoster(list& roster, string fileName);
// printing a list out
void printRoster(const list& roster);
int main(int argc, char* argv[]){
if (argc =>
// vector of courses of students
vector > courseStudents;
for(int i=1; i
list roster;
readRoster(roster, argv[i]);
cout
printRoster(roster);
courseStudents.push_back(move(roster));
}
// reading in dropouts
list dropouts;
readRoster(dropouts, argv[argc-1]);
cout
list allStudents; // master list of students
for(auto& lst : courseStudents)
allStudents.splice(allStudents.end(),lst);
cout
printRoster(allStudents);
allStudents.sort(); // sorting master list
cout
allStudents.unique(); // eliminating duplicates
cout
for (const auto& str : dropouts) // removing individual dropouts
allStudents.remove(str);
cout
}
void readRoster(list& roster, string fileName){
ifstream course(fileName);
string first, last;
while(course >> first >> last)
roster.push_back(Student(first, last));
course.close();
}
// printing a list out
void printRoster(const list& roster){
for(const auto& student : roster)
cout
}
- Here is the output for the above example roster files.
All students
last name, first name: courses enrolled
Mary Price:cs1
Stephanie Watson:cs1
Rachel Rivera:cs1
Raymond Powell:cs1
Catherine Ward:cs1
Doris Howard:cs1
Cynthia Jenkins:cs1 cs3
Betty Torres:cs1
Juan Cox:cs1 cs4
Kathleen Anderson:cs1 cs3
Mark Russell:cs1 cs2 cs4
Gerald Edwards:cs1 cs2 cs3 cs4
Mikhail Nesterenko:cs1 cs3
Charles Martin:cs1
Linda Johnson:cs1 cs2
David Collins:cs1
Elizabeth Jones:cs1
Jonathan Baker:cs1
Maria Kelly:cs1 cs4
Martin Taylor:cs1
Dennis White:cs1 cs2
Brian Sanchez:cs1 cs4
Joyce Rodriguez:cs2
Sharon Bennett:cs2
John Jenkins:cs2
Rachel Peterson:cs2
Marilyn Phillips:cs2
Catherine Morgan:cs2
Lori Walker:cs2 cs3
Julie Perry:cs2
Peter Young:cs2 cs3
Peter Hall:cs2 cs3 cs4
Catherine Powell:cs3
Anne Clark:cs3 cs4
Tina Ward:cs3
Andrew Turner:cs3
Jason Reed:cs3
Alan Wood:cs3
Elizabeth Green:cs3 cs4
Louis Harris:cs3
Judy Miller:cs4
Nicholas Peterson:cs4
Craig Jackson:cs4
Louis Gray:cs4
All students, dropouts removed and sorted
last name, first name: courses enrolled
Andrew Turner:cs3
Betty Torres:cs1
Brian Sanchez:cs1 cs4
Catherine Morgan:cs2
Charles Martin:cs1
Craig Jackson:cs4
Cynthia Jenkins:cs1 cs3
David Collins:cs1
Doris Howard:cs1
Elizabeth Green:cs3 cs4
Elizabeth Jones:cs1
Gerald Edwards:cs1 cs2 cs3 cs4
John Jenkins:cs2
Jonathan Baker:cs1
Joyce Rodriguez:cs2
Juan Cox:cs1 cs4
Judy Miller:cs4
Julie Perry:cs2
Kathleen Anderson:cs1 cs3
Linda Johnson:cs1 cs2
Lori Walker:cs2 cs3
Louis Gray:cs4
Louis Harris:cs3
Maria Kelly:cs1 cs4
Marilyn Phillips:cs2
Mark Russell:cs1 cs2 cs4
Martin Taylor:cs1
Mary Price:cs1
Nicholas Peterson:cs4
Peter Hall:cs2 cs3 cs4
Peter Young:cs2 cs3
Rachel Peterson:cs2
Rachel Rivera:cs1
Stephanie Watson:cs1
Tina Ward:cs3
- he project should be done using associative containers and class Student to hold the name of the student. Specifically, you need to use a map keyed by the class Student while value could be a list of classes the student is enrolled in. The map should be the ordered map. That is, the data structure to be used in as follows: map,>>
- 2part
- Recode the roster example so that it prints all the students currently enrolled in at least one course. That is, enrolled in a course and are not in dropout list. The name of each enrolled student has to be printed exactly once. You have to use this data structure: set. Refer to this set example for guidance. Here is an example printout:
// set/multiset example
// Mikhail Nesterenko
// 3/16/2014
#include
#include
#include
#include
using std::cout; using std::endl;
using std::cin;
using std::set; using std::multiset;
int main () {
set mySet;
multiset myMset;
srand(time(nullptr));
// mySet.push_back('a'); // -->
for(int i=0; i
// selecting random characters
char newChar = 'a' + rand()%5;
mySet.emplace(newChar);
myMset.insert(newChar);
}
// print content
cout
for(const auto& e: mySet)
cout
cout
cout
for(const auto& e: myMset)
cout
cout
cout
cout
for(const auto& e: myMset)
cout
cout
// myMset.erase(myMset.begin(), myMset.end());
myMset.clear();
cout
for(const auto& e: myMset)
cout
cout
cout
char toFind; cin >> toFind;
auto found = mySet.find(toFind);
if (found != mySet.end())
cout
else
cout
}
Currently Enrolled Students
Kathleen Anderson
Gerald Edwards
Mary Price
...
- Milestone:For the first assignment, print a master list of students (without classes they are enrolled in).
Step by Step Solution
There are 3 Steps involved in it
Step: 1
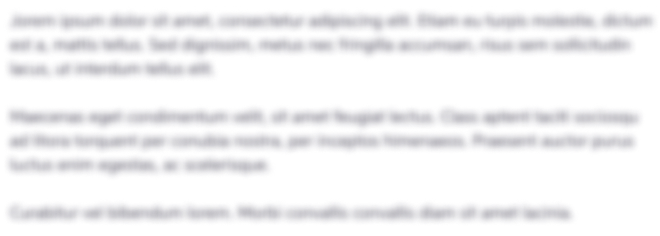
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started