Question
1. Compile Time Bugs Find and fix the five compile time bugs in the code at the end of this section. Compile time bugs show
1. Compile Time Bugs Find and fix the five compile time bugs in the code at the end of this section. Compile time bugs show as errors when you compile, but the Visual Studio IDE also gives you visual clues in the form of red squiggly underlines, as shown here . You will actually see more than five errors, but they are all caused by just five bugs (four in just one line). Remember, start with the first error in your code and work down.
Here is the code. // Week 1 Assignment-1 // Description: Compile time errors with loops and branching //----------------------------------
//**begin #include files************ #include
using namespace std; //----------------------------------
//**begin global constants********** const int arraySize = 5; //--end of global constants--------- //----------------------------------
//**begin main program************** int main() { // seed random number generator srand(time(NULL)); // create a flag to end the while loop bool endLoop = false; // create an int array int array[arraySize]; // initialize the int array for (i = 0; i
2. Run-Time Errors in Loops and Branching Loops and branching are hot spots for simple but difficult-to-spot bugs. This code is filled with some very annoying and common bugs. The code will compile fine, but it wont work right and may even crash. There are two bugs that are crash bugs. There is one logic error that causes the program to quit before it should and one typo that causes incorrect behavior. This last one will be the hardest to find because it only happens when you win, and it is an error that is notoriously hard to spot until youve been caught by it enough to expect it. This is a simple slot machine. Here is how it is supposed to work. 1. When you hit the return key, the wheel spins (you wont actually see any spinning, just the results of the spin). 2. You automatically bet $1 on each spin. 3. If you get three of the same symbols, you win according to the following table. a. Lemonsyou keep your bet. b. Cherriesyou win $5. c. Orangesyou win $10. d. Bellsyou win $15. e. Jackpotyou win $1,000 and the game is over. 4. When your pot is gone (pot == 0), you lose and the game is over. Here is the code. // Week 3 Assignment- 2 // Description: Run-time errors in loops and branching //----------------------------------
//**begin #include files************ #include
using namespace std; //----------------------------------
//**begin main program************** int main() { // seed random number generator srand(time(NULL)); // create enum for symbols enum symbol { Lemon, Cherry, Orange, Bell, Jackpot }; // create a struct for slot machine wheel struct Wheel { array
bool gameOn = true; bool winner = false; int thePot = 100; int bet = 1; vector
3. Create a Program From Pseudocode This exercise will be to use pseudocode (in the form of comments) to write a program that simulates a one-sided snowball fight. You select the row and column you want the snowball to hit; if the target is at that location, you get a point; otherwise, you get the distance (but not the direction) of the target from the snowball hit point. The distance is calculated by taking the absolute value of the difference between the x position of the target and the snowball hit and the y position and the snowball hit. Report the larger of the two if they are different. After each throw of the snowball, the target moves using the following algorithm. Generate a random number (02). The target doesnt move if the random number is 0. If the random number is 1, the target changes its x position. If the random number is 2, it changes its y position. Randomly choose whether to increment by one or decrement by one. If the target is on the boundary of the grid, move away from the boundary by one. The grid size should be 5. The number of turns should be 10. Here is the pseudocode. // Week 3 Assignment-3 // Description: Snowball fight - version 1 //----------------------------------
//**begin #include files************ #include
using namespace std; //----------------------------------
//**begin global constants********** // define a struct of the target // -- xPos is the x position (the column) // -- yPos is the y position (the row) // -- xHit is x position of the hit(the column) // -- yHit is y position of the hit(the row) // -- distance between target and snowball hit // -- hits is how many times the target has been hit // const grid size (i.e. 5x5 grid constant is 5) // const number of turns //--end of global constants--------- //----------------------------------
//**begin main program************** int main() { // initialization // seed the random number generator // create the target struct // set target at random location // set hits to zero // loop for the specified number of turns // get x and y position for the snowball from player // compare to the target's position // report hit, or distance of miss (see instructions for details) // target moves (see instruction for details) // end of loop // report score (number of hits vs turns) // Wait for user input to close program when debugging. cin.get(); return 0; } //--end of main program------------- //----------------------------------
Step by Step Solution
There are 3 Steps involved in it
Step: 1
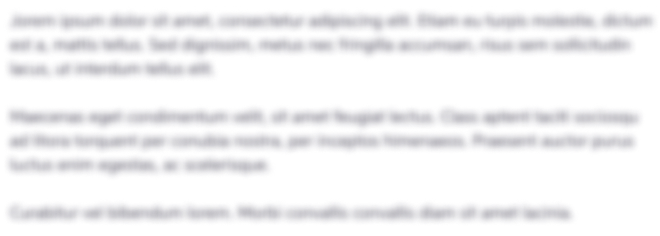
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started