Question
1) Complete the function rotate(c, n), which takes as inputs a single character c and a non-negative integer n between 0 and 25, and that
1) Complete the function rotate(c, n), which takes as inputs a single character c and a non-negative integer n between 0 and 25, and that returns a single character that is based on c. If c is a letter of the alphabet, the function should “rotate” it by n characters forward in the alphabet, wrapping around as needed. For example:
>>> rotate('a', 1)
result: 'b'
>>> rotate('B', 3)
result: 'E'
2) Write a function encipher(s, n) that takes as inputs an arbitrary string s and a non-negative integer n between 0 and 25, and that uses recursion to create and return a new string in which the letters in s have been “rotated” by n characters forward in the alphabet, wrapping around as needed. Upper-case letters should be rotated to upper-case letters, lower-case letters should be rotated to lower-case letters, and non-alphabetic characters should be left unchanged.
For example:
>>> encipher('hello', 1)
result: 'ifmmp'
>>> encipher('hello', 2)
result: 'jgnnq'
>>> encipher('ABCXYZ', 3)
result: 'DEFABC'
>>> encipher('xyzabc', 4)
result: 'bcdefg'
>>> encipher('#caesar!', 2)
result: '#ecguct!'
3) In our starter code for ps3pr3, we’ve given you a fully implemented function called letter_score(c) that takes a single-character string c and returns a numeric score that is based on the frequency with which that character appears in English-language text documents. For example:
>>> letter_score('t')
result: 0.0737
>>> letter_score('m')
result: 0.0205
Note that the score for 't' is higher than the score for 'm', because 't' occurs more frequently in English-language text documents than 'm' does.
You should not modify letter_score. Rather, you will use it as a helper function in your next function.
4) Write a function called string_score(s) that takes an arbitrary string s and that uses either recursion OR a list comprehension to compute and return the sum of the letter-score values of the characters in s. Your function must call the provided letter_score function as a helper function to determine the score of a given letter.
Here’s one example of how it should work:
>>> string_score('then')
result: 0.2853
Note that this call returns 0.2853, which is the sum of the letter scores of 't', 'h', 'e' and 'n'.
Here are two other examples:
>>> string_score('caesar')
result: 0.3497
>>> string_score('brutus')
result: 0.2274
5) Write a function decipher(s) that takes as input an arbitrary string s that has already been enciphered by having its characters “rotated” by some amount (possibly 0). The function should return, to the best of its ability, the original English string, which will be some rotation (possibly 0) of the input string s. For example:
>>> decipher('Bzdrzq bhogdq? H oqdedq Bzdrzq rzkzc.')
result: 'Caesar cipher? I prefer Caesar salad.'
Note that decipher does not take a number specifying the amount of rotation! Rather, it should determine the rotation (out of all possible rotations) that produces the most plausible English string.
Here is the approach that you should take:
a) Start by using a list comprehension to create a list of all possible decipherings, and assign that list to a variable. You should end up with a line of code that looks like this:
options = [_____________ for n in ____________]
You need to consider all possible shifts – from 0 to 25 – that could be used to create a deciphering, and thus the second blank above should be replaced with an expression that produces the necessary sequence of integers.
And since deciphering involves rotating a string, you’ve already written the function needed to create each of the possible decipherings! You should make the appropriate call to it in the first blank above.
b) Next, use the “list-of-lists” technique that we discussed in lecture to assign a numeric score to each of the possible decipherings in options. The best_word function from lecture is a good example of this technique.
Call your string_score function to provide the score of each option. Because the scores that string_score provides are based on how frequently the individual characters appear in English documents, strings that are more likely to be English phrases should end up with higher scores!
c) After you have scored all of the options, you should choose the option with the highest score and return it. Here again, the best_word function from lecture is a good model for what you need to do.
Once you think you have a working decipher, try it on the following string:
'kwvozibctibqwva izm qv wzlmz nwz iv mfkmttmvb rwj'
Step by Step Solution
There are 3 Steps involved in it
Step: 1
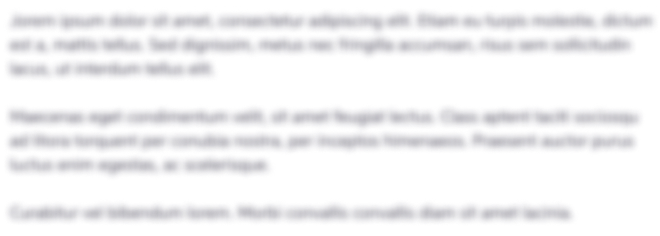
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started