Question
1 Concentration Game 100 points For this assignment you will implement a modied version of Concentration Game. Check out the Wikipedia page on the Concentration
1 Concentration Game 100 points For this assignment you will implement a modied version of Concentration Game. Check out the Wikipedia page on the Concentration Game here: https://en.wikipedia.org/wiki/Concentration_(game) 1 Note that in this assignment (both here and in the code) I used words deck and board interchangeably, for basically the same thing. Beforereadingthedescriptionbelow,watchthevideoImadeonhowyourgameshouldplay: https://youtu.be/M5hiCkZ7308 I also provide you with a le A3-example-runs.txt that contains the example runs (those I typed while making the video). Watching the videp will make following the below description much easier. Note that the video does not have a sound as it is not needed. All the requirements implied by the video and example runs in A3-example-runs.txt and the provided code in a3_xxxxxx.py and the requirements stated in this assignment should be considered mandatory requirements for the assignment. You may assume that the player will follow your instructions and enter the correct date type but not necessarily the correct data values for that type. For example, if you ask the player for an even integer between 2 and 52 you may assume that indeed they will enter an integer (rather than a real number say), but your program should test if the player entered an integer in the required range and if it is indeed even number. Your program should prompt the player to repeat the entry until correct input is obtained. You should also fully test your program for example, what does your program do if the player does something silly, like enteres a location that is already discovered, or enters two same locations ... Watch the video to see how your program should behave in these cases. Also, think of the design of your program. For example, your program should have a function that displays the current board (I provided that fully), but it should also have a function that displays the board with two new positions revealed (I only provided a header and docstrings for that function). These functions should be called from your game playing function (called play_game). Designing your program by decomposing it into smaller subproblems (to be implemented as functions) makes programming easer, less prone to errors and makes your code more readable. You will be graded on these aspects of your program too. The game is usually played with cards lied down on a table. Your game will not use graphics libraries to display the board, but instead it will print the board with the tools that we have seen in class so far, i.e. printing on Python console. A typical card has a suit and a rank on one side. Lets call that side of a card, a face side, and the other case a dull side. Instead of suit and a rank each of our cards will have a single character on its face side and that character will be an upper case letter of english alphabet or any special character (e.g those you can nd on the upper row of your keyboard). A typical card deck has the same drawing on the dull side of the cards. Instead of a drawing our cards will have a character star, *, on their dull side. From now on we can assume we start o with such a deck with some number of cards. For the deck to be suitable for playing Concentration game, each character that appears in the deck needs to appear on an even number of cards. Furthermore, the deck cannot have cards with * on their face sides, as we could not distinguish a face side from an a dull side of such cards. Therefore before playing we need to clean up the deck. In particular, we need to remove all the cards that have a * on their faces. In addition, for each character that appears on an odd number of cards, we need to remove exactly one card with that character from the deck. After such a clean-up, the resulting deck can be used for playing Concentration game. We call such a deck, the one where each character appears on even number of cards and where no card has * on its face side, a playable deck. Finally we call a deck rigorous if it is playable and each character of the deck appears exactly two times. Your game must use a (one dimensional) list as the board, as implied by the provided code in a3_xxxxxx.py The game starts o by asking the player (1) if they wanted a (rigorous) deck, and thus board, automatically generated (2) or if they want to start with the deck given in a le. If option (1) is chosen, you need to rst prompt the player for the size of the deck. You should only accept even integers, between 2 and 52 for Option 1. As part of this assignment, I provided you with a fully coded function, called create_board, that returns a rigorous deck of the given size. Thus option 1 only plays a game with rigorous decks. If option (2) is chosen, the deck will be read from a le. The player will enter a name of that le when prompted. I provide you with a couple of practice les. (TAs will test your assignment with these and some other les.) To use them, you need to put those les in the same directory/folder as your program a3_xxxxxx.py. As part of this assignment, I provided you with a fully coded function, called read_raw_board, that reads the le and returns a list containing the deck of cards specied in the le. This deck however is not necessarily playable. Thus you rst need to make it playable. For that you will code a function called clean_up_board. I provide a header and docstrings for that function. That function should return a playable deck and that is the deck you will play the game with in Option 2. But before playing the game with it, for the fun of it, you would like to know if the resulting deck is not only playable but also rigorous. For that you will need to complete the function called is_rigorous for which I provide you with the header and docstrings. Finally you will notice that a3_xxxxxx.py has a function called play_game(board). That is where you should put your game paying code. The function play_game takes a playable deck as input parameter (i.e. a deck created by option 1 or option 2). Once the player completes the game, you should also print the number of guesses it took to solve it and how far it is from the optimal (although impossible without luck) len(board)/2 guesses. Outside of those functions, i.e. in the main you will do initial communication with the player, and whatever else is specied in the a3_xxxxxx.py.
import random def shuffle_deck(deck): '''(list of str)->None Shuffles the given list of strings representing the playing deck ''' # YOUR CODE GOES HERE random.shuffle(deck) print(deck)
def create_board(size): '''int->list of str Precondition: size is even positive integer between 2 and 52 Returns a rigorous deck (i.e. board) of a given size. ''' board = [None]*size
letter='A' for i in range(len(board)//2): board[i]=letter board[i+len(board)//2 ]=board[i] letter=chr(ord(letter)+1) return board
def print_board(a): '''(list of str)->None Prints the current board in a nicely formated way ''' for i in range(len(a)): print('{0:4}'.format(a[i]), end=' ') print() for i in range(len(a)): print('{0:4}'.format(str(i+1)), end=' ')
def wait_for_player(): '''()->None Pauses the program/game until the player presses enter ''' input(" Press enter to continue. ") print()
def print_revealed(discovered, p1, p2, original_board): '''(list of str, int, int, list of str)->None Prints the current board with the two new positions (p1 & p2) revealed from the original board Preconditions: p1 & p2 must be integers ranging from 1 to the length of the board ''' # YOUR CODE GOES HERE
############################################################################# # FUNCTIONS FOR OPTION 1 (with the board being read from a given file) # #############################################################################
def read_raw_board(file): '''str->list of str Returns a list of strings represeniting a deck of cards that was stored in a file. The deck may not necessarifly be playable ''' raw_board = open(file).read().splitlines() for i in range(len(raw_board)): raw_board[i]=raw_board[i].strip() return raw_board
def clean_up_board(l): '''list of str->list of str
The functions takes as input a list of strings representing a deck of cards. It returns a new list containing the same cards as l except that one of each cards that appears odd number of times in l is removed and all the cards with a * on their face sides are removed ''' print(" Removing one of each cards that appears odd number of times and removing all stars ... ") playable_board=[]
# YOUR CODE GOES HERE return playable_board
def is_rigorous(l): '''list of str->True or None Returns True if every element in the list appears exactlly 2 times or the list is empty. Otherwise, it returns False.
Precondition: Every element in the list appears even number of times '''
# YOUR CODE GOES HERE
####################################################################3
def play_game(board): '''(list of str)->None Plays a concentration game using the given board Precondition: board a list representing a playable deck '''
print("Ready to play ... ")
# this is the funciton that plays the game # YOUR CODE GOES HERE
#main # YOUR CODE TO GET A CHOICE 1 or CHOCE 2 from a player GOES HERE
# YOUR CODE FOR OPTION 1 GOES HERE # In option 1 somewhere you need to and MUST have a call like this: # board=create_board(size)
# YOUR CODE FOR OPTION 2 GOES HERE # In option 2 somewhere you need to and MUST have the following 4 lines of code one after another # #print("You chose to load a deck of cards from a file") #file=input("Enter the name of the file: ") #file=file.strip() #board=read_raw_board(file) #board=clean_up_board(board)
Step by Step Solution
There are 3 Steps involved in it
Step: 1
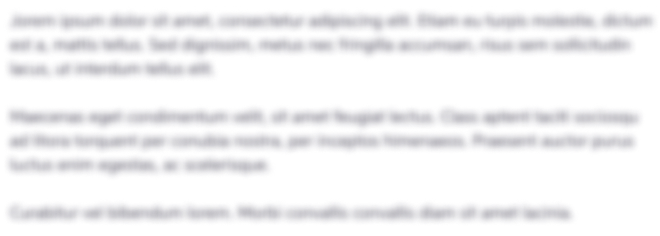
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started