Question
1. Create a new project. Then, create the void method with the following header: public static void displayInstructions() Once this is created, complete the method
1.
Create a new project. Then, create the void method with the following header:
public static void displayInstructions()
Once this is created, complete the method body so that it outputs the instructions for Blackjack to the player. The Instructions for blackjack are:
First, the dealer and player each start with two cards drawn. The goal of the game is to get as close to 21 card points as possible. Having a card total of 21 points results in a win (unless the dealer also has a total of 21 points, in which case, it is a tie). If anyone goes over 21 points, they automatically lose. If both the dealer and player get over 21 card points, it is a tie. Otherwise, whoever is closest to 21 is deemed winner.
Copy and paste only your method into the textbox provided below.
next question
2.
Look at the following program before continuing.
import java.util.Scanner; public class Calculator{ public static void main(String[] args){ Scanner scan = new Scanner(System.in); displayMenu(); System.out.print("Enter your choice: "); int choice = scan.nextInt(); if(choice == 1){ System.out.print("Enter two numbers to add: "); int num1 = scan.nextInt(); int num2 = scan.nextInt(); System.out.println("Sum = " + add(num1, num2)); } // Complete the code... } public static void displayMenu(){ System.out.println("1. Add"); System.out.println("2. Subtract"); System.out.println("3. Subtract with doubles"); System.out.println("4. Multiple"); System.out.println("5. Divide"); } public static int add(int a, int b){ int sum = a + b; return sum; } public static int subtract(int a, int b){ return a - b; } public static double subtract(double a, double b){ // Complete the code here. Pay attention to data types! } // Create a multiplication method that returns a double // Create a division method that returns a double }
Step 1:
After reading through and understanding the program given above, create a new project. Copy and paste the code above into your new project.
There will be some errors, since the subtract(double a, double b) method is not yet complete. Write some code in that method so that it returns the answer. Copy and paste your subtract(double a, double b) method in the textbox below.
Step 2:
Look at the main() method now, and notice how it is already set up for you. Continue on with the program by adding the required if-statements and input/output for options 2 and 3 (subtraction with ints, and subtraction with doubles).
Make sure to properly call your methods for both types of subtractions.
Once complete, copy and paste your whole main() method in the textbox below.
Step 3:
Let's fix the displayMenu() method!
First, edit the method to do the following:
We want to also allow the user to quit. Include a "Quit" option in the print statements!
Have it get the user input from within the method itself and return an int based on the user's choice. (Make sure to also edit the method header and how it is called back in the main() method!) What is the method's return type now? [ Select ] ["int", "double", "String"]
Include input validation for the user's choice before returning the value. For example, if the user enters something that is not an option (such as a 10), tell them to choose again. Keep asking them to enter a number until the input is valid. Only return an integer that is a valid option. What will you use to create this input validation? [ Select ] ["if-statement", "loop"]
Back in the main() method, add a loop now as well! The user should be able to keep choosing options until they decide to quit. Only stop iterating once they've chosen the option to quit.
Display a goodbye message at the end of the program.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
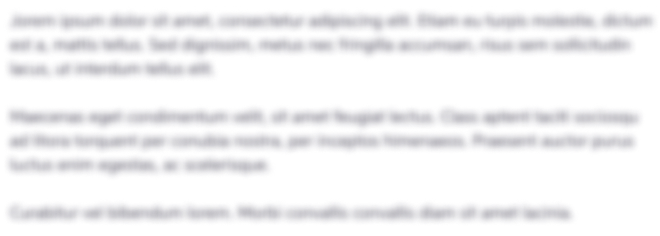
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started