Question
1. Define a MergeSort class. In this class, your sort() method should take a sequence of integer numbers as input andreturn a sorted array in
1. Define a MergeSort class. In this class, your sort() method should take a sequence of integer numbers as input andreturn a sorted arrayin descending order.
2. Define a QuickSort class. your sort() method should take a sequence of integer numbers as input andreturn a sorted arrayin descending order.
3. In your Main class,build a test method to test your code.
public static boolean test(int[] result, int[] ans);
The test method should take two arrays as input. Pass the sorted array from your sorting function into 'result' and pass an array that you have sorted by hand to 'ans'. Your test method should check if these two arrays are exactly the same, return true whenthey are the same and false when they not. You should generate at least 3 test cases and use these test cases to test all sorting algorithms above.
For example:
-------------------------------------------------------
int[] result =sortObject.sort( {4,2,3,1} ); int[] ans = {1,2,3,4}; test(result, ans );
-------------------------------------------------------
Would return true if the sorting algorithm is working correctly.
4. In your Main class, build a compare method.
public static boolean compare(int[] arr1, int[] arr2);
This method take two arrays as input and return true when theyare equal and return false otherwise. Two arrays are said to be equal if both of them contain same set of elements,order of elements may be different though. You can assume that in one array, one element will only appear one time. For example,
-------------------------------
Input: arr1[] = {2,4,1,3,5} arr2[] = {2,4,1,3,5} Output: True
Input: arr1[] = {1,3,5,2,4} arr2[] = {2,4,1,3,5} Output: True
Input: arr1[] = {1,7,2,8,5} arr2[] = {2,4,1,3,5} Output: False
-------------------------------
5. In yourMainclass, build afindSmallestSum method.
public static int findSmallestSum(int[] array);
This method will take an array as input and return the smallest sum of two elements in this array. You can assume that the length of input array is always >= 2. For example,
----------------------------------------
Input: array = {4,7,0,0,2,9,10} Output: 0
----------------------------------------
Step by Step Solution
There are 3 Steps involved in it
Step: 1
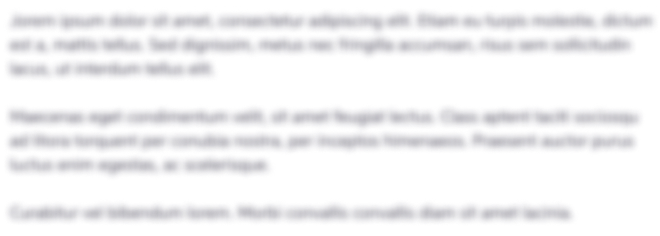
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started