Question
1 Description Imagine you have a computer system, and attached to the system bus is a secondary bus for specialized hardware. This project is about
1 Description
Imagine you have a computer system, and attached to the system bus is a secondary bus for specialized hardware. This project is about creating a simulation of the interaction between these devices on the secondary bus. The secondary bus will have connected to it a specialized RAM storage device that is used as a buffer, a specialized I/O module, and a specialized transfer device that can move data between the I/O module to buffer. The computer system will instruct the transfer device to transfer a certain amount of data, and will print the results to the screen.
2 Details
The computer system, transfer device, buffer device, and I/O modules will all be simulated with their own program. The secondary bus will be simulated with pipes and a program to manage the multiple devices. This means you will need to write a total of five (5) programs. The entire project should be launched by running the computer system program giving it two arguments: the input file name, and the number of bytes to read and write to screen. As an example in java it might look like this on the command line:
java ComputerSystem exampleinput.txt 200
The computer system program should then launch the bus program, giving it the file- name as an argument, and connecting to its standard input and output via pipes. The bus program will then launch the other programs, giving the I/O module the file name, and connect to their standard input and outputs through pipes.
The computer system will then command the transfer module to transfer the number of bytes given on the command line to the buffer. Whenever the buffer is full, the transfer device will pause its transfer and the computer system will transfer those bytes from the buffer to itself, printing them to the screen. If the number of bytes to be read have not finished, the computer system sends the command to continue the transfer.
Once done, the computer system will send the command to quit to the bus program, that will send it to its children, causing the simulation to end.
Operating Systems Concepts Page 1
CS/SE 4348.502 Project 01 Spring 2020
2.1 Simulated Hardware
2.1.1 Messages Between Programs
Messages sent along the pipes represent the electronic signals that would physically be sent. Each message will be exactly 16-bit, each bit representing the state of a physical connection (You can think of these as wires or traces on the motherboard). The first 8-bits are control bits that determine how to interpret the message. The first bit determines whether or not the interrupt (or acknowledgment in the case of the computer system) is active. The second bit determines if the message is carrying data. If the third bit is 1, then the device that receives it should quit. The rest of the first byte is a device number representing the device that is expected to receive the data or acknowledgement if it exists.
A device should ignore any messages that does not contain its id in the control byte. For the data byte, each device will have its own protocol, which will be left up to you as the designer.
2.1.2 Bus
The various hardware devices are connected through the bus. This program simulates the bus by requiring all communication to go through it. It will check for input from each device program and send a copy to all other programs. The system bus will be a 16- bit bus, but 8-bits will be reserved for control information so only a single byte can be transferred at a time. This bus is a secondary one connected to the system bus. The buss program will accept a single command line argument: the name of the input file.
The bus process is responsible for spawning the buffer, I/O module, and transfer device processes, and connecting to them via pipes.
2.1.3 Buffer
The buffer program represents a special RAM device that holds a single 128 byte buffer. This device is byte addressable, so there are 128 addresses. TO access a memory cell, the
Operating Systems Concepts Page 2
CS/SE 4348.502 Project 01 Spring 2020
device must be told the address of the byte as well as the mode (read or write). In the case of a write operation, the device must be told the value to be written.
You can view this program as a wrapper around a 128 byte array.
2.1.4 I/O Module
The I/O module simulates the systems communication with the outside world. It will be considered to be attached to a I/O device (such as a keyboard) that will have uneven bursts of input. It will read from the input file that will instruct the program when input is available, or when a delay in the input happens. Whenever a character can be read in from the I/O device, It will be read into the 8-byte internal buffer. If the value in the buffer is not retrieved then the I/O module must pause. It can only read in a new character when the contents of the buffer had been sent. The I/O module program will accept a single command line argument: the name of the input file.
2.1.5 Transfer Device
This program represents a specialized hardware device whose job it is to transfer data from the I/O module to the buffer, signalling the cpu when it is done.
The CPU(Computer System Processes in this case) will send a command to initiate the transfer, telling the transfer device how many bytes to transfer. It will only transfer 128 bytes at a time, but will remember how many bytes are left to transfer, allowing the cpu to continue the transfer with a single message.
2.1.6 Computer System
This program represents the rest of the system. This includes the cpu, main memory, and the terminal output I/O device. This program will be a simulation, so you only need to program the behavior of these systems with respect to the secondary bus. As command line arguments, this program is expected to be provided the name of the input file and the number of bytes it wants to echo. It will request the transfer device to transfer that many bytes from the I/O module to the buffer. The computer system will then read the buffer and print to screen its contents.
The computer system will be the program that is launched to run your simulation. It is responsible for spawning the bus and connecting to it via pipes.
2.2 Input File
The input file is a series of lines representing delays and inputs. Each line will begin with either a t,d, or n.
t. If the line begins with a t, then all characters on that line, not including the newline, are input to the I/O module.
n. If the line begins with an n, then it represents a newline as input to the I/O module. Anything else on the line is ignored
Operating Systems Concepts Page 3
CS/SE 4348.502 Project 01 Spring 2020
d. If the line begins with a d, then it must be followed by a space and then an integer. The I/O module will have to wait that many milliseconds before it can read the next line.
2.2.1 Example
tHello World n d 100 tGood Bye
n
This input file represents an input of the characters Hello World followed by a newline character, and then after 100 milliseconds the sequence of characters Good Bye will be inputted, followed by a newline character.
2.3 Output
The Computer System, will print the contents, up to a specified number of bytes, of the input to the screen.
2.3.1 Example
With the input file example given in the Input File section, if the number of bytes to transfer are 20 or more then the output should look like:
Hello World Good Bye
If the number of bytes are less than 20, say 15, then the output will cut the input short.
Hello World Goo
Newlines should be represented by a single byte. This byte will need to be interpreted by the computer system program when printing to account for different operating systems
public class Multiprocess { public static void main(String[] args) { try{ while(System.in.available() == 0); System.out.println("Available"); int x,z=0; char y; while((x = System.in.read()) != -1){ y = (char)x; System.out.print(y); while(System.in.available() == 0)z++; System.out.println(">>" + z); z=0; } } catch(Exception e) { e.printStackTrace(); } } }
import java.lang.*; import java.util.Scanner; public class MainMemory { public static void main(String[] args) { String mode; int memLoc = 0; Scanner s = new Scanner(System.in); mode = s.next(); while(!mode.equals("halt")) { if(mode.equals("read")) System.out.println(memLoc); else if(mode.equals("write")) memLoc = s.nextInt(); mode = s.next(); } } }
import java.lang.*; import java.io.*; import java.util.Scanner; public class CPU { public static void main(String[] args) { try { Process mem = Runtime.getRuntime().exec("java MainMemory"); InputStream inStream = mem.getInputStream(); OutputStream outStream = mem.getOutputStream(); Scanner fromMem = new Scanner(inStream); PrintStream toMem = new PrintStream(outStream); for(int i=10; i>=0; i--) { toMem.println("write"); toMem.println(i); System.out.print("Set to "); toMem.println("read"); toMem.flush(); System.out.println(fromMem.nextInt()); } toMem.println("halt"); toMem.flush(); mem.waitFor(); } catch(IOException ex) { System.out.println("Unable to run MainMemory"); } catch(InterruptedException ex) { System.out.println("Unexpected Termination"); } } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
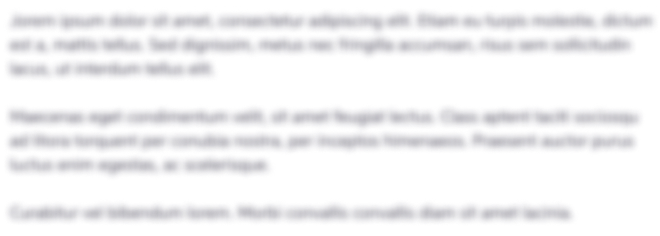
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started