Question
1. double sum = 0; double d; for (d = 0; d < 10; d = d + 0.1 ) { sum = sum +
1.
double sum = 0;
double d;
for (d = 0; d < 10; d = d + 0.1 )
{
sum = sum + d;
}
a. The program has a syntax error because the semicolons in the for loop are in the wrong place
b. The program has a syntax error because the control variable in the for loop cannot be double.
c. The program compiles but does not stop because d would always be less than 10.
d. .The program compiles and runs fine.
2.
What is y after the following for loop statement is executed?
int y = 0;
for (int i=0; i < 10; i ++)
{
y ++;
}
a. 9
b. 10
c. 11
d. 12
3.
Array arr is declared and initialized in the following line of code:
int [ ] arr = {10, 7, 3, 5, 9};
What is arr[3] - arr[1]?
a. -7
b. 7
c. -2
d. 2
4.
Which of the following is a valid array declaration and initialization?
a. char [ ] charArray;
charArray = new char[26];
b. int [ ] words;
words = new words[10];
c. char [ ] charArray;
charArray = 15;
d. double [3] nums;
5.
Consider the following code fragment:
int [ ] list;
list = new int[10];
for (int i = 0; i <= 10; i = i + 1)
{
list[i] = 0;
}
Which of the following statements is true?
a. The loop body will execute 9 times.
b. The program has a syntax error because the control variable, i, in the for loop is not defined in the body of the loop.
c. The loop body will execute 10 times, filling up the array with zeros.
d. The code has a runtime error indicating that the array is out of bound.
6.
Assume the declaration of the method xMethod is as follows. This means that xMethod is sent one object that is an array of double values.
public static void xMethod(double [ ] a);
Which of the following could be used to call xMethod?
a. xMethod(5.7);
b. xMethod(this one is correct);
c. double [ ] z = {8.4, 9.3, 10.7};
xMethod( z[2] );
d. double [ ] z;
z = new double[6];
xMethod( z );
7.
Analyze the following code:
public class Test
{
public static void main(String[] args)
{
int [ ] x = {0, 1, 2, 3, 4, 5};
xMethod(x, 5);
}
public static void xMethod(int [ ] x, int len)
{
for (int i = 0; i < len; i = i + 1)
{
System.out.print( " " + x[i] );
}
}
}
a. The program displays 0 1 2 3 4.
b. The program displays 0 1 2 3 4 and then raises a runtime exception.
c. The program displays 0 1 2 3 4 5.
d. The program displays 0 1 2 3 4 5 and then raises a runtime exception.
8.
Given the following statements:
int [ ] list;
list = new int[10];
a. The array variable list contains an object that refers to an array of 10 int values |
b. The array variable list contains an object that refers to an array of 9 int values
d. The above has a compiler error.
9. Given the following statements, length is a property of all arrays. By writing the name of an array followed by a dot, followed by the word length, it will return the total number of locations in the array.
int [ ] list = new int[10]; int n = list.length;
What is the value of n? a. 10 b. 9 c. The value depends on how many integers are stored in the array list d. 0
10. True or false? When a variable is sent to a method and the variable has a primitive data type, then the method can change the value of that variable. |
Step by Step Solution
There are 3 Steps involved in it
Step: 1
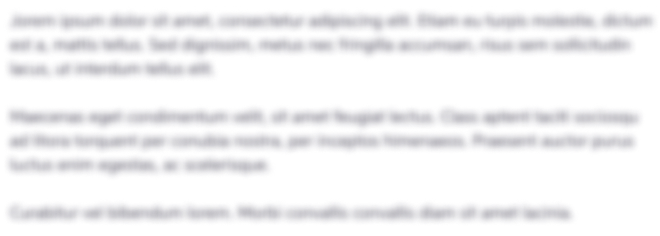
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started