Question
1. For this assignment you will need the following files which are given: a. The file dynamic_array_driver.cpp contains the declaration and implementation for the dynamic
1. For this assignment you will need the following files which are given:
a. The file dynamic_array_driver.cpp contains the declaration and implementation for the dynamic array class tlist.
b. The file ourData.txt contains input data for the program.
c. The file dynamic_array_output.txt contains the output that the main program you write will produce when you add it to the file dynamic_array_driver.cpp.
2. Comment every item in the private and private areas of the class declaration. Be specific in your choice of words. Consider the following as appropriate comments for some of the items in the class declaration:
a. Destructor
b. Copy construction
c. Delete an item from the dynamic array
d. Overloaded assignment operator as a member function with chaining..
e. The dynamic array
4. Write a function header for each function in the class declaration (see file dynamic_array_driver.cpp). Each function header should be placed above the function implementation; the code that is provided is enough information for you to write correct function headers. Remember, function headers should include the function name, pre- condition, post-condition, and function description.
5. Write a main program to produce the output, exactly, as in the file dynamic_array_output.txt. Use the file ourData.txt for input to your program. The body of your main program (which you will add to the file dynamic_array_driver.cpp) should have no more than 20 lines of code; this includes blank lines. The key to doing a good job on this assignment, is to read and understand the code that is provided. Hint: any output and/or messages should be done inside the functions of the class. Your main program should only use cout when invoking (calling) the overloaded insertion operator (<<).
6.) The output of your program must match the data in the file: dynamic_array_output.txt
------------------------dynamic_array_driver.cpp-----------------------------
#include
#include
#include
using namespace std;
class tlist
{
public:
tlist();//default
~tlist();
tlist(const tlist &);
bool IsEmpty();
bool IsFull();
int Search(const string &);
void Insert(const string &);
void Remove(const string &);
void Triple_size();
tlist & operator=(const tlist &);
friend ostream & operator<<(ostream &, const tlist &);
private:
string *DB;
int count;
int capacity;
};
//***************************Sample Header Format**********************
// Function Name: Default Constructor
// Pre-Condition: The count, capacity and the dynamic array DB have not been
initialized.
// Post-Condition:The count, capacity and the dynamic array DB have been
initialized.
// Description:The default constructor initializes that state of the class.
//
//********************************************************************
tlist::tlist()
{
string s;
ifstream input;
input.open("ourData.txt");
if (input.fail())
{
cout << "problem with input data file ";
exit(1);
}
cout << " Default Constructor Invoked "
<< "*************************** ";
count = 0;
capacity = 1;
DB = new string[capacity];
IsEmpty();
while (!input.eof())
{
input >> s;
Insert(s);
}
input.close();
}
tlist::~tlist()
{
cout << " Destructor Invoked "
<< "******************* ";
delete[] DB;
}
tlist::tlist(const tlist & org)
{
cout << " Copy Constructor Invoked "
<< "************************* ";
count = org.count;
capacity = org.capacity;
DB = new string[capacity];
for (int i = 0; i < count; i++)
{
DB[i] = org.DB[i];
}
}
bool tlist::IsEmpty()
{
cout << " IsEmpty Invoked "
<< "**************** ";
return count == 0;
}
bool tlist::IsFull()
{
cout << " IsFull Invoked "
<< "*************** ";
return count == capacity;
}
int tlist::Search(const string & key)
{
for (int i = 0; i < count; i++)
{
if (DB[i] == key)
{
return i;
}
}
return -1;
}
void tlist::Insert(const string & key)
{
if (IsFull())
{
Triple_size();
}
DB[count++] = key;
}
void tlist::Remove(const string & key)
{
int i = Search(key);
if (i == -1)
{
if (IsEmpty())
{
cout << "DB is empty ";
}
else
{
cout << key << " not in DB ";
}
}
else
{
for (int j = i; j < count - 1; j++)
{
DB[j] = DB[j + 1];
}
cout << key << " found and deleted ";
count--;
}
}
void tlist::Triple_size()
{
cout << " Triple_size Invoked "
<< "*************************** ";
capacity *= 3;
string *temp = new string[capacity];
for (int i = 0; i < count; i++)
{
temp[i] = DB[i];
}
delete[] DB;
DB = temp;
}
tlist & tlist::operator=(const tlist & org)
{
cout << " overloaded assignment operator (=) Invoked "
<< "*************************** ";
if (this != &org)
{
delete[](*this).DB;
(*this).count = org.count;
(*this).capacity = org.count;
(*this).DB = new string[(*this).capacity];
for (int i = 0; i < (*this).count; i++)
{
(*this).DB[i] = org.DB[i];
}
}
return *this;
}
ostream & operator<<(ostream & cout, const tlist & org)
{
for (int i = 0; i < org.count; i++)
{
cout << org.DB[i] << endl;
}
return cout;
}
int main()
{
//YOUR ADD YOUR CODE FOR MAIN HERE
return 0;
}
---------------------ourData.txt---------------------
cloud
azure
microsoft
sql
database
firefox
netscape
myworld
algorithm
object
class
seacrest
multiplicity
associativity
amazon
------------------dynamic_array_output.txt-------------------
Default Constructor Invoked
***************************
IsEmpty Invoked
****************
IsFull Invoked
***************
IsFull Invoked
***************
Triple_size Invoked
***************************
IsFull Invoked
***************
IsFull Invoked
***************
Triple_size Invoked
***************************
IsFull Invoked
***************
IsFull Invoked
***************
IsFull Invoked
***************
IsFull Invoked
***************
IsFull Invoked
***************
IsFull Invoked
***************
Triple_size Invoked
***************************
IsFull Invoked
***************
IsFull Invoked
***************
IsFull Invoked
***************
IsFull Invoked
***************
IsFull Invoked
***************
IsFull Invoked
***************
cloud
azure
microsoft
sql
database
firefox
netscape
myworld
algorithm
object
class
seacrest
multiplicity
associativity
amazon
Copy Constructor Invoked
*************************
cloud
azure
microsoft
sql
database
firefox
netscape
myworld
algorithm
object
class
seacrest
multiplicity
associativity
amazon
google found and deleted
cloud
azure
microsoft
sql
database
firefox
netscape
myworld
algorithm
object
class
seacrest
multiplicity
associativity
amazon
amazon found and deleted
cloud
azure
microsoft
sql
database
firefox
netscape
myworld
algorithm
object
class
seacrest
multiplicity
associativity
firefox found and deleted
cloud
azure
microsoft
sql
database
netscape
myworld
algorithm
object
class
seacrest
multiplicity
associativity
IsEmpty Invoked
****************
xyz not in DB
cloud
azure
microsoft
sql
database
netscape
myworld
algorithm
object
class
seacrest
multiplicity
associativity
Default Constructor Invoked
***************************
IsEmpty Invoked
****************
IsFull Invoked
***************
IsFull Invoked
***************
Triple_size Invoked
***************************
IsFull Invoked
***************
IsFull Invoked
***************
Triple_size Invoked
***************************
IsFull Invoked
***************
IsFull Invoked
***************
IsFull Invoked
***************
IsFull Invoked
***************
IsFull Invoked
***************
IsFull Invoked
***************
Triple_size Invoked
***************************
IsFull Invoked
***************
IsFull Invoked
***************
IsFull Invoked
***************
IsFull Invoked
***************
IsFull Invoked
***************
IsFull Invoked
***************
overloaded assignment operator (=) Invoked
***************************
cloud
azure
microsoft
sql
database
firefox
netscape
myworld
algorithm
object
class
seacrest
multiplicity
associativity
amazon
Destructor Invoked
*******************
Destructor Invoked
*******************
Destructor Invoked
*******************
Step by Step Solution
There are 3 Steps involved in it
Step: 1
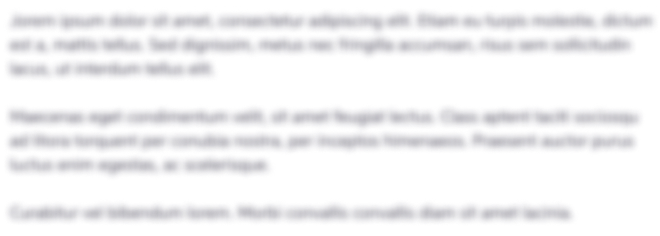
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started