Question
1- get the current time and adjust the clock to have the current time. 2-Allow smoother translation of the seconds hand. 3- Enhance the colors
1- get the current time and adjust the clock to have the current time.
2-Allow smoother translation of the seconds hand.
3- Enhance the colors of the clock in general (hands and the frame cirlce).
here is the c++ code to modify
#include
#include
void init() { glClearColor(0.0, 0.0, 0.0, 0.0); glColor3f(0.0f, 0.0f, 0.0f); glPointSize(5.0f); //gluOrtho2D(0.0f, 640.0f, 0.0f, 480.0f); glEnable(GL_POINT_SMOOTH); glEnable(GL_POLYGON_SMOOTH); glEnable(GL_LINE_SMOOTH); }
int seconds = 0; int minutes = 0;
void Timer(int value) { glutTimerFunc(1000, Timer, 0); glutPostRedisplay(); seconds+=6; if(seconds==360) { minutes+=6; seconds = 6; } printf("%s %d","Seconds = ", seconds); } double ticks = 0;
// Your first Point The hello world application void drawpoint() { glPushMatrix(); glRotated(ticks += 1, 0, 0, 1); glTranslated(0.9, 0, 0); glBegin(GL_POINTS); glVertex2d(0, 0); glEnd(); glPopMatrix(); }
void drawcircle() { // glLoadIdentity(); //Reset the drawing perspective for (int i = 0; i < 360; i++) { glPushMatrix(); glRotated(ticks += 1, 0, 0, 1); glTranslated(1, 0, 0); glColor3f(1.0, 0.0, 0.0); glBegin(GL_POINTS); glVertex2d(0, 0); glEnd(); glPopMatrix(); } /* OpenGL keeps a stack of matrices to quickly apply and remove transformations. glPushMatrix copies the top matrix and pushes it onto the stack, while glPopMatrix pops the top matrix off the stack. All transformation functions (glScaled, etc.) function on the top matrix, and the top matrix is what all rendering commands use to transform their data. By pushing and popping matrices, you can control what transformations apply to which objects, as well as apply transformations to groups of objects, and easily reverse the transformations so that they don't affect other objects. */ // Seconds hand glPushMatrix(); //Tells OpenGL to store the current state that we are in. glRotated(-seconds, 0, 0, 1); glBegin(GL_POLYGON); glVertex2d(-0.01, 0); glVertex2d(0.01, 0); glVertex2d(0.01, 0.6); glVertex2d(0, 0.9); glVertex2d(-0.01, 0.6); glEnd(); glPopMatrix(); //Then when we want to go back to our previous state, we call glPopMatrix().
// Minutes hand glPushMatrix(); glRotated(-minutes, 0, 0, 1); glColor3f(0.0, 0.0, 1.0); glBegin(GL_POLYGON); glVertex2d(-0.01, 0); glVertex2d(0.01, 0); glVertex2d(0.01, 0.6); glVertex2d(0, 0.8); glVertex2d(-0.01, 0.6); glEnd(); glPopMatrix();
// hours hand glPushMatrix(); glRotated(0, 0, 0, 1); glColor3f(1.0, 0.1, 1.0); glBegin(GL_POLYGON); glVertex2d(-0.02, 0); glVertex2d(0.02, 0); glVertex2d(0.02, -0.6); glVertex2d(0, -0.8); glVertex2d(-0.02, -0.6); glEnd(); glPopMatrix();
} void display(void) { glClear(GL_COLOR_BUFFER_BIT); glLineWidth(2.5); glColor3f(1.0, 0.0, 0.0); drawcircle(); glFlush(); } int main(int argc, char **argv) { glutInit(&argc, argv); glutInitDisplayMode(GLUT_SINGLE | GLUT_RGB); glutInitWindowPosition(200, 200); glutInitWindowSize(640, 480); glutCreateWindow("Square"); glutDisplayFunc(display); init(); Timer(0); glutMainLoop(); return 0; }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
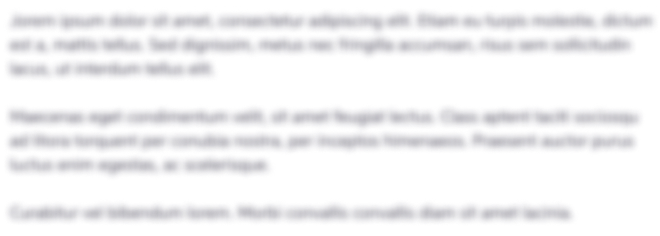
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started