Question
1. Implement a tostring() member function. This function will return a string representation of the LargeInteger. You should probably used string streams to implement this
1. Implement a tostring() member function. This function will return a string representation of the LargeInteger. You should probably used string streams to implement this function. Note that the digits of the large integer are stored in reverse of their display order, e.g. the 1's place (100 ) is in index 0 of digits, the 10's place (101 ) is in index 1, etc.
2. Implement a second constructor for the LargeInteger. This constructor will be used to construct a large integer from an array of digits. This constructor takes an int, which is the size of the array of digits, and an integer array as the second parameter. You will need to dynamically allocate the space for the digitis in your constructor (see the given constructor for an example).
3. Implement a member function named maxDigits(). This function will take a reference to a LargeInteger as its only parameter. This function should return the number of digits in the larger LargeInteger comparing the passed in object to this object.
4. Implement a member function named digitAtPlace(). This function returns the digit of this large integer object at a particular digit index. So this function takes an integer index as its input parameter, and returns an integer as its result. Again, if you ask for the digitAtPlace() index 0, this should return the 1's place 100 digit of the integer. If asked for the digit at place 1, this should return the 10's place 101 digit. If you ask for a digit place that is larger than the number of digits in the integer the member function should return 0. For example, if the integer represents 345, and you ask for the digit at place 3, then the function will return a 0. Likewise, if a negative index is given to this function, it should also return 0.
5. Implement a member function named appendDigit(). This functions takes an int digit as its only parameter. This will be a void function as it does not return any value result from calling it. The function is not 2 something a normal user of the LargeInteger type is likely to need to do to an integer, but it will be very useful for our add function. This function appends the digit to become the new most signicant digit of the LargeInteger object. For example, if the large integer object represents the value 345 and we ask it to appendDigit(7), then the new value of the object will be 7345. This function needs to manage memory to perform its task. You need to perform the following steps 1) allocate a new array of digits of numDigits+1 (e.g. enough space to hold one more digit). 2) copy the old digits to the new array of digits you created. 3) append the new passed in digit to the end of the array. Then 4) free up the old original array of digits, and make sure you class now uses the new array of allocated digits. Also, you might want to have this function ignore the append of a '0' digit (e.g. do not grow the large integer if trying to append a 0, as this does not need to be represented by the LargeInteger).
6. And finally, implement the add() member function. This function takes a reference to another LargeInteger object, and adds the digits of the other object to this object together.
Code that mucst be moddified
Main file
#include
using namespace std;
/** main * The main entry point for this program. Execution of this program * will begin with this main function. * * @param argc The command line argument count which is the number of * command line arguments provided by user when they started * the program. * @param argv The command line arguments, an array of character * arrays. * * @returns An int value indicating program exit status. Usually 0 * is returned to indicate normal exit and a non-zero value * is returned to indicate an error condition. */ int main(int argc, char** argv) { // test constructors, destructors and tostring() cout << "Testing Constructors, tostring() and destructor:" << endl; cout << "-------------------------------------------------------------" << endl; LargeInteger li1(3483); //cout << "li1 = " << li1.tostring() << endl; //assert(li1.tostring() == "3483");
//int digits2[] = {8, 4, 6, 3, 8, 4, 7, 4, 1, 2}; //LargeInteger li2(10, digits2); //cout << "li2 = " << li2.tostring() << endl; //assert(li2.tostring() == "2147483648");
LargeInteger* li3 = new LargeInteger(193839); //cout << "li3 = " << li3->tostring() << endl; //assert(li3->tostring() == "193839"); delete li3; // should invoke the class destructor // test maxDigits() member function cout << endl; cout << "Testing maxDigits() member function" << endl; cout << "-------------------------------------------------------------" << endl; int maxDigits; //maxDigits = li1.maxDigits(li2); //cout << "The maximum digits between l1 and l2 is: " << maxDigits << endl; //assert(maxDigits == 10);
//LargeInteger li4(398298312); //int digits5[] = {3, 3, 1, 4, 2, 1, 5, 1, 2, 4, 7, 6, 9, 3, 9, 5, 6}; //LargeInteger li5(17, digits5); //cout << "li4 = " << li4.tostring() << " and li5 = " << li5.tostring() << endl; //maxDigits = li5.maxDigits(li4); //cout << "The maximum digits between l5 and l4 is: " << maxDigits << endl; //assert(maxDigits == 17);
// test digitAtPlace() member function cout << endl; cout << "Testing digitAtPlace() member function" << endl; cout << "-------------------------------------------------------------" << endl; //cout << "li5[0] == " << li5.digitAtPlace(0) << endl; //assert(li5.digitAtPlace(0) == 3); //cout << "li5[16] == " << li5.digitAtPlace(16) << endl; //assert(li5.digitAtPlace(16) == 6); //cout << "li5[17] == " << li5.digitAtPlace(17) << endl; //assert(li5.digitAtPlace(17) == 0); //cout << "li5[4] == " << li5.digitAtPlace(4) << endl; //assert(li5.digitAtPlace(4) == 2); //cout << "li5[10] == " << li5.digitAtPlace(10) << endl; //assert(li5.digitAtPlace(10) == 7); //cout << "li5[25] == " << li5.digitAtPlace(25) << endl; //assert(li5.digitAtPlace(25) == 0); //cout << "li5[-1] == " << li5.digitAtPlace(-1) << endl; //assert(li5.digitAtPlace(-1) == 0); //cout << endl;
//cout << "li4[0] == " << li4.digitAtPlace(0) << endl; //assert(li4.digitAtPlace(0) == 2); //cout << "li4[8] == " << li4.digitAtPlace(8) << endl; //assert(li4.digitAtPlace(8) == 3); //cout << "li4[9] == " << li4.digitAtPlace(9) << endl; //assert(li4.digitAtPlace(9) == 0); //cout << "li4[2] == " << li4.digitAtPlace(2) << endl; //assert(li4.digitAtPlace(2) == 3); //cout << "li4[33] == " << li4.digitAtPlace(33) << endl; //assert(li4.digitAtPlace(33) == 0); //cout << "li4[-5] == " << li4.digitAtPlace(-5) << endl; //assert(li4.digitAtPlace(-5) == 0);
// test append digit cout << endl; cout << "Testing appending digits appendDigit() member function" << endl; cout << "-------------------------------------------------------------" << endl; //li5.appendDigit(1); //cout << "li5 after appendDigit(1) = " << li5.tostring() << endl; //assert(li5.tostring() == "165939674215124133"); //li5.appendDigit(0); //cout << "li5 after appendDigit(0) = " << li5.tostring() << endl; //assert(li5.tostring() == "165939674215124133");
//li4.appendDigit(7); //cout << "li4 after appendDigit(7) = " << li4.tostring() << endl; //assert(li4.tostring() == "7398298312"); //li4.appendDigit(0); //cout << "li4 after appendDigit(0) = " << li4.tostring() << endl; //assert(li4.tostring() == "7398298312"); // test addition operator cout << endl; cout << "Testing LargeInteger addition add() member function" << endl; cout << "-------------------------------------------------------------" << endl; //LargeInteger li6 = li4.add(li5); //cout << "Addition of li4 + li5 = " << li6.tostring() << endl; //assert(li6.tostring() == "165939681613422445"); //LargeInteger li7 = li5.add(li4); //cout << "Addition of li5 + li4 = " << li7.tostring() << endl; //assert(li7.tostring() == "165939681613422445");
//assert(li6.tostring() == li7.tostring());
// test carry //LargeInteger li8(999999999); //int digits9[] = {9, 9, 9, 9, 9, 9, 9, 9, 9}; //LargeInteger li9(9, digits9);
//cout << setw(12) << right << li8.tostring() << endl; //cout << "+" << setw(11) << right << li9.tostring() << endl; //cout << "------------" << endl; //LargeInteger li10 = li8.add(li9); //cout << setw(12) << right << li10.tostring() << endl; cout << endl; // return 0 to indicate successful completion return 0; }
LargeInteger.cpp code below
#include "LargeInteger.hpp"
using namespace std;
// integer to create unique id for new LargeInteger instances // please set and use this in the same way in the constructor you // create for this assignment static int nextLargeIntegerId = 1;
/** LargeInteger constructor * Constructor for LargeInteger class that takes a simple built-in * integer to be used as the initial value of the large integer. * * @param value A regular (32-bit) integer that we will use as the * initial value of this LargeInteger data type. */ LargeInteger::LargeInteger(int value) { // set this instance id id = nextLargeIntegerId++; // first determine number of digits, so we know what size of array // to construct dynamically // https://stackoverflow.com/questions/1489830/efficient-way-to-determine-number-of-digits-in-an-integer numDigits = (int) log10((double) value) + 1;
// allocate an array of the right size digits = new int[numDigits];
// iterate through the digits in value, putting them into our // array of digits. int digit; for (int digitIndex = 0; digitIndex < numDigits; digitIndex++) { // least significant digit digit = value % 10; digits[digitIndex] = digit;
// integer division to chop of least significant digit value = value / 10; } }
/** LargeInteger destructor * Destructor for the LargeInteger class. Make sure we are good * managers of memory by freeing up our digits when this object * goes out of scope. */ LargeInteger::~LargeInteger() { // We display a log message to track destructor invocation. In // a real application we might send this to a log file, and parameterize // whether to log these type of events or not. cout << "
LargeInteger.hpp below this
#ifndef _LARGEINTEGER_H_ #define _LARGEINTEGER_H_
#include
using namespace std;
/** LargeInteger class. * * @param id Private member integer variable, this is not strictly needed * for this class, but we assign a unique id to each instance of * LargeInteger that is created, so that you can more easily see how * the destructor works and is being called. You should set the * id and increment it in any constructors you create for this class. * @param numDigits Private member integer variable, contains the number * of digits currently in the LargeInteger, or equivalently, the size * of they digits array of integers. * @param digits A dynamically allocated array of integers. This array * holds the digits of the large integer this object represents. The * digits in the array are orderd such that the 1's place (10^0) is in * index 0 of the array, the 10's place (10^1) is in the index 1, and so * on. */ class LargeInteger { private: int id; int numDigits; // number of digits in LargeInt / size of alloc array int* digits; // the digits of the LargeInt we are representing
public: LargeInteger(int value); ~LargeInteger(); };
#endif
Code must follow this
1. All indentation must be correct according to class style guidelines (2 spaces, no tabs, all code blocks correctly indented by level).
2. All functions (and member functions of classes) must have a function header documentation that gives a short description, documents all input parameters using @param tags, and documents the return value if the function returns a value, using @returns tag. You do not have to document class setter/getter methods, but all other class methods should probably be documented
Step by Step Solution
There are 3 Steps involved in it
Step: 1
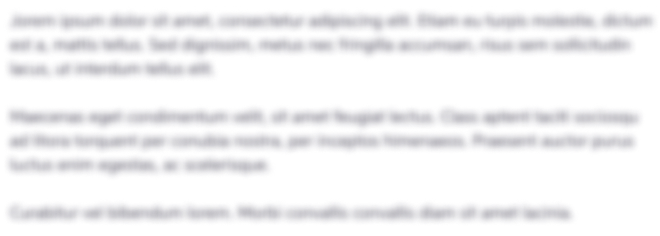
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started