Question
1. Implement the methods specified for the unbounded stack (LinkedStack) Add an inspector method inspect(int loc). The method will return the element found at the
1. Implement the methods specified for the unbounded stack (LinkedStack) Add an inspector method inspect(int loc). The method will return the element found at the location loc. Return null if the location is invalid. Implement the method popSome(int count). The method will remove the top count items from the stack. The method should throw a StackUnderFlow Exception as needed. Note that you may have some room for decisions in this method, but possible less choices than with the Array implementation. Document your approach in the comments. Without adding any new instance variables, implement a size() method. The method will return an integer value indicating the number of elements in the stack. You will need to traverse your stack nodes using a while loop similar to the example in class. ** These methods will not be part of the StackInterface or the BoundedStackInterface. You dont need to define them in the interface declarations. 2. Test your code in the LinkedStackTester to show the results of using the new methods
LinkedStack
package ch03;
import support.LLNode;
public class LinkedStack
protected LLNode
public LinkedStack() {
this.top = null;
}
@Override
public void pop() throws StackUnderflowException {
// TODO Auto-generated method stub
if (isEmpty())
throw new StackUnderflowException("Stack is empty");
else {
top = top.getLink();
}
}
@Override
public T top() throws StackUnderflowException {
// TODO Auto-generated method stub
if (isEmpty())
throw new StackUnderflowException("Stack is empty");
else {
return top.getInfo();
}
}
@Override
public boolean isEmpty() {
// TODO Auto-generated method stub
return (top == null);
}
@Override
public void push(T element) {
// TODO Auto-generated method stub
LLNode
newNode.setLink(top);
top = newNode;
}
}
LLNode
package support;
public class LLNode
private LLNode
private T info;
public LLNode
return link;
}
public void setLink(LLNode
this.link = link;
}
public T getInfo() {
return info;
}
public void setInfo(T info) {
this.info = info;
}
public LLNode(T info) {
this.info = info;
this.link = null;
}
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
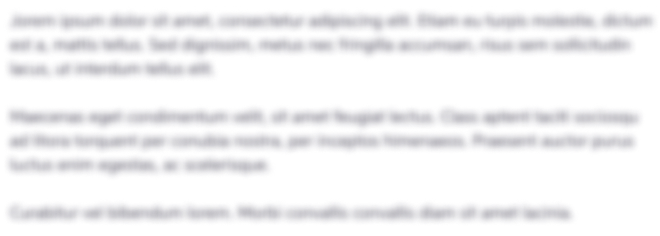
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started