Answered step by step
Verified Expert Solution
Question
1 Approved Answer
1 ) In a module named dynamicArray ( . c and . h files ) write the following function: float * createArray ( int size,
In a module named dynamicArray c and h files write the following function: float createArrayint size, float initialValue; Remember the function prototype ie the function declaration that specifies the function's name, parameters and return type goes in the header h file. The function body goes in the corresponding source c file. The function should return a pointer to a dynamically allocated float array with size members, each of which is initialized to initialValue. The return value should be NULL if the array can't be allocated, or if size is Test your function by writing a program named labEc whose main function: Calls createArray to create an array of size and initialize array elements to a value of your choice. size and initialValue should be hard coded into the program. Prints the elements of the array, one per line, to stdout Frees all allocated memory Dynamically allocating an array of structs In a module named stuff c and h files implement the functions whose prototypes are given below. These functions are tested by a program named Ac For this exercise, you may use only malloc and free to allocate and deallocate memory. The program uses the following structure You must use a tag structure, not a typedef: struct stuff char name; a string int length; the length of name ; The function prototypes of the stuff module are: Purpose: Creates a dynamic array of struct stuff elements of length size Parameters: size the size of the array to be created Return: a pointer to the array or NULL if allocation fails or if size is struct stuff makeArrayint size; Purpose: Given a string input and a valid pointer to a struct stuff, the function fills the struct stuff with the provided name and sets the length to the string length Parameters: data a name string entry the struct stuff to be filled Return: None void makeStuffstruct stuff entry char data; Purpose: Given a valid pointer to a struct stuff, this function prints the stored string and the length field to stdout Parameters: entry the struct stuff to be printed Return: None void printStuffstruct stuff entry; Purpose: This function takes a pointer to an array of struct stuff elements and frees it along with all its dynamically allocated components Parameters: size the size of the passed array myArray pointer to an array of struct stuff elements Return: None void freeStuffint size, struct stuff myArray; In a file named labEc write a main function, which: Creates a dynamic array of struct stuff of size using your makeArray function. Fills the array with the strings "Hanan", "Philip", "Mohammed", and "YourName" using your makeStuff function. The strings should be hardcoded into the program. Prints the contents of the array to stdout using the printStuff function. Frees all allocated memory using the freeStuff function. In addition to the normal make rules to compile the program using separate compilation add an additional rule, named valgrindLabE to test your program with valgrind and check for memory leaks and readwrite errors.
In a module named dynamicArray c and h files write the following function:
float createArrayint size, float initialValue;
Remember the function prototype ie the function declaration that specifies the function's name, parameters and return type goes in the header h file. The function body goes in the corresponding source c file. The function should return a pointer to a dynamically allocated float array with size members, each of which is initialized to initialValue. The return value should be NULL if the array
can't be allocated, or if size is
Test your function by writing a program named labEc whose main function:
Calls createArray to create an array of size and initialize array elements to a value of your choice. size and initialValue should be hard coded into the program.
Prints the elements of the array, one per line, to stdout
Frees all allocated memory
Dynamically allocating an array of structs
In a module named stuff c and h files implement the functions whose prototypes are given below. These functions are tested by a program named Ac For this exercise, you may use only malloc and free to allocate and deallocate memory.
The program uses the following structure You must use a tag structure, not a typedef:
struct stuff
char name; a string
int length; the length of name
;
The function prototypes of the stuff module are:
Purpose: Creates a dynamic array of struct stuff elements of length size
Parameters: size the size of the array to be created
Return: a pointer to the array or NULL if allocation fails or if size is
struct stuff makeArrayint size;
Purpose: Given a string input and a valid pointer to a struct stuff, the
function fills the struct stuff with the provided name and sets the
length to the string length
Parameters:
data a name string
entry the struct stuff to be filled
Return: None
void makeStuffstruct stuff entry char data;
Purpose: Given a valid pointer to a struct stuff, this function prints the
stored string and the length field to stdout
Parameters: entry the struct stuff to be printed
Return: None
void printStuffstruct stuff entry;
Purpose: This function takes a pointer to an array of struct stuff elements
and frees it along with all its dynamically allocated components
Parameters:
size the size of the passed array
myArray pointer to an array of struct stuff elements
Return: None
void freeStuffint size, struct stuff myArray;
In a file named labEc write a main function, which:
Creates a dynamic array of struct stuff of size using your makeArray function.
Fills the array with the strings "Hanan", "Philip", "Mohammed", and "YourName" using your
makeStuff function. The strings should be hardcoded into the program.
Prints the contents of the array to stdout using the printStuff function.
Frees all allocated memory using the freeStuff function.
In addition to the normal make rules to compile the program using separate compilation add an additional rule, named valgrindLabE to test your program with valgrind and check for memory leaks and readwrite errors.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
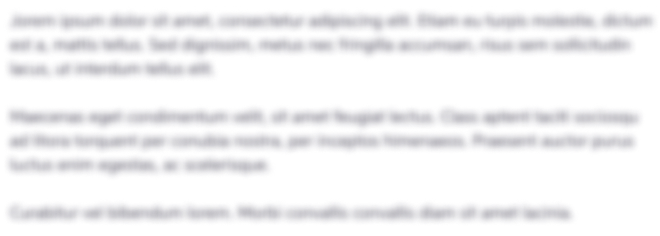
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started