Question
1. Insertion Sort (sorts an array) public void insertionSort(Comparable[] array, int lowindex, int highindex, boolean reversed); Modify the code of the insertion sort we discussed
1. Insertion Sort (sorts an array) public void insertionSort(Comparable[] array, int lowindex, int highindex, boolean reversed); Modify the code of the insertion sort we discussed in class (posted on github) so that: It sorts all elements in the array with indices in the range from lowindex to highindex (inclusive). You should not touch any of the data elements outside the range lowindex to highindex. Your code should work for different values of lowindex and highindex, not just 0 and n-1. If reversed is false, the list should be sorted in ascending order. If the reversed flag is true, the list should be sorted in descending order. To sort in descending order, you need to make changes to the algorithm itself. You may not just sort the list in ascending order and then reverse it - no credit for such solutions. Can sort the array of any Comparable objects, not just integers.
Code Needing to update:
public static void insertionSort(int[] arr) { | |
int curr; | |
int j; | |
for (int i = 1; i < arr.length; i++) { | |
curr = arr[i]; | |
j = i - 1; | |
while (j >= 0 && arr[j] > curr) { | |
arr[j + 1] = arr[j]; // shift elems to the right | |
j--; | |
} | |
arr[j + 1] = curr; | |
} | |
} | |
I cannot use any built in methods.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
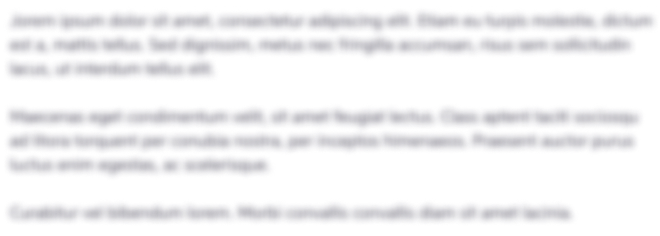
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started