Question
1. instructions, and my files named Account.h and Account.cpp. i dont know what to do next need some help!! a. Youll note that the Account
1. instructions, and my files named Account.h and Account.cpp. i dont know what to do next need some help!!
a. Youll note that the Account class has a static unsigned int named accountTally. This tally is used inside each constructor for the Account class to set the accountNumber variable for an account; the accountTally is incremented inside each constructor so that each new account gets the next account number.
b. The Account classs second constructor, which accepts two std::string arguments by constant reference, uses these arguments to set the first and last names for the account holder.
c. The Account classs display() method displays the details of the account. Specifically, it displays the first name, last name, account number and balance.
d. Note that balance is an unsigned long long. As you may recall, floating-point types contain mere approximations to real numbers; as a result, when reliable precision is required, we may want to use integer types instead. Here, we can think of balance as representing the total number of cents in an account. In order to display the value as dollars and cents, we can use integer division by 100 to get the dollar amount, and take the balance modulo 100 to get the number of cents that wed place after the decimal point. For example, an account with 10 dollars and 75 cents would have a balance of 1075. In order to represent this value in dollars and cents, we could calculate 1075 / 100 to obtain the dollar value, and 1075 % 100 to obtain the remaining cents, so that we could display the total value as $10.75.
e. The deposit() method checks to make sure the argument is greater than zero. If so, the incoming value is added to the balance.
f. All getters and setters of Account exhibit their expected behavior (the setFirstName() method sets the first name, etc.).
2. Write the header and implementation files for the following three classes: Checking, PremiumChecking, and Savings. Each of these classes should be derived from the Account class using public inheritance. a. Checking class i. A checking account allows for withdrawals to be made. ii. In addition to a default constructor and a constructor that sets the first and last names, Checking should have a withdraw() method. 1. The withdraw() method takes a parameter of type unsigned long long, representing the amount that should be withdrawn. 2. If the incoming amount is positive and less than or equal to the balance, it should be deducted from the balance. If it is greater than the balance, the overdrawnCount variable should be incremented and the incoming amount should not be deducted from the balance (i.e., the attempted withdrawal fails). If overdrawnCount is less than 3, a fee of $10.00 should be deducted from the balance. Otherwise, a fee of $30.00 should be deducted from the balance. If a fee is charged, the user should be informed of this fact. iii. The Checking class should have a display() method, which overrides the display() method of Account. It should first call the display() method of the Account class and then display the number of times the Checking account has been overdrawn. The Account classs display() method can be called from within any of its children by writing the fully qualified name of the method: Account::display(). b. PremiumChecking class i. A premium checking account requires a monthly fee, but allows the account holder to bounce the occasional check (up to a point). Accordingly, this class should have a fee data member of type unsigned long long, initialized to represent $5.75. ii. In addition to a default constructor and a constructor that sets the first and last names, PremiumChecking will have a chargeMonthlyFee() method that simply deducts the monthly fee from the balance. It should also have getter and setter methods for the monthly fee. iii. The PremiumChecking class should have a withdraw() method. The withdraw() method of this class should check to make sure the incoming argument is positive. 1. If so, the incoming argument should also be checked to see whether it is less than the balance. In that case, the amount can simply be deducted from the balance. If the incoming argument is greater than the balance, then the transaction should still be permitted as long as it doesnt result in a balance below negative $200. If the new balance would be below negative $200, then the transaction should not proceed and the user should be informed of this fact. iv. The PremiumChecking class should have a display() method, which overrides the display() method of Account. It should first call the display() method of the Account class and then display the monthly fee.
c. Savings class i. A savings account has an interest rate. Accordingly, this class should have an interestRate data member of type double, initialized to 0.0. ii. In addition to a default constructor and a constructor that sets the first name, last name, and interest rate, the Savings class should have getter and setter methods for the interest rate. iii. The Savings class should have a display() method, which overrides the display() method of Account. It should first call the display() method of the Account class and then display the interest rate.
3. Finally, write a driver file that meets the following requirements: a. Present the user with a menu that allows for creating a user-specified number of accounts of any type, or for displaying the details of all accounts created thus far. b. If the user chooses to create accounts, prompt the user for the number of accounts to create. The driver should then create this number of accounts, populating their data members with randomly chosen values. i. Hint: to randomly create names, you could create two separate vectors with first and last names of your choice, then use a random-number-generator to choose the names. ii. These accounts should be created on the heap, and each of their addresses stored in a pointer-to-Account. For example, for with a checking account, we could say: Account* temp = new Checking(); iii. As each account is created, the corresponding pointer-to-Account should be pushed into a std::vector and its details displayed for the user. 1. Note: at this point you are working with pointers to type Account, so the Account pointer will not know about the Savings classs setInterestRate() method. You must therefore use a dynamic cast to convert the pointer-to-Account into a pointer-to-Savings: Savings* sPtr = dynamic_cast
//Account.h
#pragma once #include
//Account.cpp #include
Sample Output: MENU: 1. Create Checking accounts 2. Create Premium accounts 3. Create Savings accounts 4. Display all accounts 5. Quit Selection: 1 Choice 1 How many checking accounts would you like to create? 4 Account details: First name: Juliet Last name: Wu Account number: 1 Balance: $57074.24 Overdrawn count:0
Account details: First name: George Last name: Goldsworthy Account number: 2 Balance: $48512.51 Overdrawn count:0
Account details: First name: Bob Last name: Philbrick Account number: 3 Balance: $83609.6 Overdrawn count:0
Account details: First name: Isabelle Last name: Kagan Account number: 4 Balance: $84490.73 Overdrawn count:0
Step by Step Solution
There are 3 Steps involved in it
Step: 1
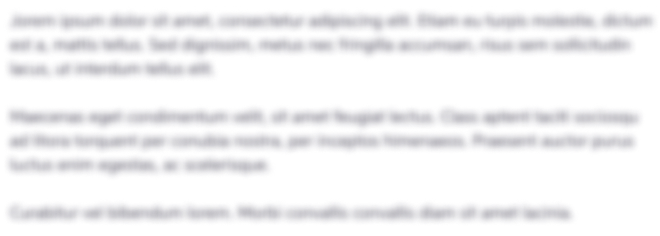
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started