Answered step by step
Verified Expert Solution
Question
1 Approved Answer
1 Introduction Images in this specification are from Carl Kingsford s lectures on KD - Trees You have now seen several ADTs in Python that
Introduction
Images in this specification are from Carl Kingsfords lectures on KDTrees
You have now seen several ADTs in Python that manage collections of ob jects, like stacks, trees and queues. A common question that we need to ask of collections is as follows: what value in this collection is most similar to item X This is called a nearest neighbour search. In this assignment, you will implement an abstract data structure called a kdtree short for kdimensional tree that efficiently supports this kind of a search.
KDTrees: A primer
Figure A KDTree where K At left, an illustration of the way the tree partitions dimensional space. At right, the nodes in the KDtree.
A kdtree is a form of a binary search tree that stores points in kdimensional space. You can use a kdtree to store a collection of points on a plane or in
higherdimensional space. In this assignment, youll be building a kdtree to store data about municipal trees and to quickly find trees based on their lo cation. As in your labs, locations will be defined as longitude and latitude coordinates on a map.
At the root of a kdtree, the xvalue in the coordinate pair is used to sepa rate nodes at the next level. All of the nodes to the right of the root have a larger or equal xvalue than the node at the root and all nodes to the left of the root have a smaller xvalue than the node at the root.
At the next level in the kdtree it is the yvalue that is used to separate nodes. In Figure the nodes to the left of the one labelled all have a yvalue less than The nodes to the right if there were any would all have a yvalue greater than or equal to
Because of the way data is organized within a kdtree, we can efficiently query whether a given point exists within it as follows. Given a coordinate pair XY we start at the root of the tree. If the root node contains XY we know the value exists in the tree. However, if the xvalue is strictly less than the xvalue of the root node, we will search for XY in the left subtree. Otherwise, we will descend into the right subtree. When we descend a level, we will again ask if the node we are exploring contains XY If not, we will compare the yvalue in our coordinate pair to the yvalue at this node. If the yvalue in the coordinate pair is strictly less than the yvalue of the node, we will search for XY in the left subtree and otherwise, we will descend into the right subtree. We will continue this process, alternating between x and y comparisons at each level, until we reach the leaves of the kdtree. We will either find the value we seek there or fail, in which case we know the value does not exist in our collection.
Your assignment will be to a KDTree class in Python that allows clients to build kdtrees and perform some lookup operations with them. We will specifi cally encode operations to assess if a value exists in the tree as well as operations to locate points that are similar, or near a given query. This search for points that are similar to a query is called a nearest neighbour search. We will describe each operation in more detail below.
Step : Tree Construction
Constructing a KDtree involves recursively partitioning the space in which our data points lie into smaller regions. These algorithm below assumes our data contains points in a dimensional space and that the root of our KDtree is anchored at a depth of When you build your own KDtree, your data will consist of longitude and latitudes that are associated with municipal trees. The first coordinate x will be the latitude, and the second y the longitude. The construction process which you will encode in the build treedata depth
method follows:
If there is only one point in the input data, we will create a single leaf or KDNode to represent this point and return it This will be the root of our tree, and were done.
Otherwise and if depth is even, we will split the data into two subsets based on the value of the xcoordinate. Call P the set of points with xcoordinates strictly less than the median value, and let P be all the remaining points.
If instead the depth is odd, we will split the list of input points into two subsets based on the value of the ycoordinate. Call P the set of points with ycoordinates strictly less than the median value, and let P be all the remaining points.
We will now recursively call build tree with input parameters P and depthie build treeP depth and we will assign the KDNode returned from this recursive call to the variable vleft.
We will also recursively build tree with input parameters P and depthie call build treeP depth and will assign the KDNode returned from this recursive call to a variable vright.
Finally, we will manufacture a KDNode to store the data point associated with the median and make vright the right child of this node and vleft the left
Step by Step Solution
There are 3 Steps involved in it
Step: 1
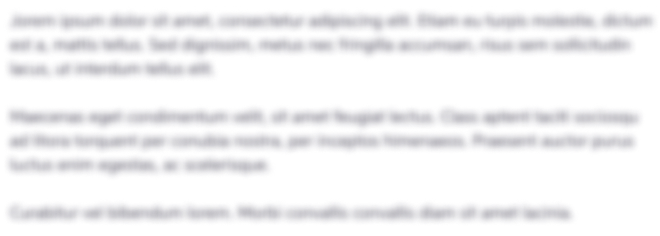
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started