Answered step by step
Verified Expert Solution
Question
1 Approved Answer
1. Modify the linked list class to include the following member functions: A member function named search that returns the position of a specific value
1. Modify the linked list class to include the following member functions:
A member function named search that returns the position of a specific value in the linked list. The first node in the list is at position 0, the second node is at position 1, and so on. If x is not found on the list, the search should return -1.
A member function for inserting a new item at a specified position. A position of 0 means that the value will become the first item on the list, a position of 1 means that the value will become the second item on the list, and so on. A position equal to or greater than the length of the list means that the value is placed at the end of the list.
Here is the likedlist.cpp
#include "LinkedList.h"
#include
using namespace std;
LinkedList::LinkedList()
{
head = NULL; // empty list
}
void LinkedList::appendNode(int data)
{
Node *tempNode = head;
Node *newNode = new Node;
newNode->data = data;
newNode->next = NULL;
if (!head) // head == NULL empty list
{
head = newNode;
}
else
{
while (tempNode->next) //tempNode->next != NULL
{
tempNode = tempNode->next;
}
tempNode->next = newNode;
}
}
void LinkedList::displayList()
{
Node *tempNode = head;
while (tempNode) // tempNode != NULL
{
cout << tempNode->data << endl;
tempNode = tempNode->next;
}
}
LinkedList::~LinkedList()
{
Node *tempNode = head;
Node *preNode = head;
while (tempNode) // tempNode != NULL
{
preNode = tempNode;
tempNode = tempNode->next;
delete preNode;
}
}
void LinkedList::deleteNode(int data)
{
Node *tempNode = head;
Node *preNode = head;
if (!head) // empty list
{
return;
}
else if (head->data == data) // delete the first value
{
head = head->next;
delete tempNode;
}
else
{
while (tempNode && tempNode->data != data)
{
preNode = tempNode;
tempNode = tempNode->next;
}
if (tempNode)
{
preNode->next = tempNode->next;
delete tempNode;
}
}
}
void LinkedList::insertNode(int data)
{
Node *newNode = new Node;
newNode->data = data;
Node *tempNode = head;
Node *preNode = head;
if (!head) // empty list
{
head = newNode;
newNode->next = NULL;
}
else if (data <= head->data) // value goes to the beginning of the list
{
newNode->next = head;
head = newNode;
}
else
{
while (tempNode && tempNode->data < data)
{
preNode = tempNode;
tempNode = tempNode->next;
}
preNode->next = newNode;
newNode->next = tempNode;
}
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
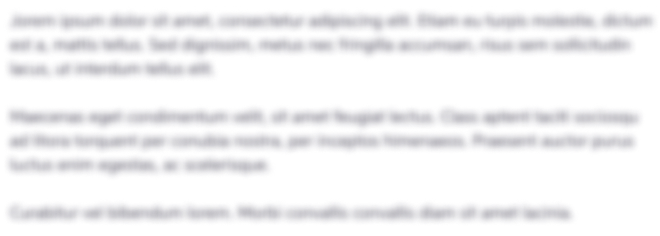
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started