Question
1. Name the class Test1 2. You must implement the following methods in your class. Note that the methods must follow exactly the headers given,
1. Name the class Test1
2. You must implement the following methods in your class. Note that the methods must follow exactly the headers given, and perform the duties exactly as they are described below each method. Helpful built in methods are listed, and while not required, are suggested for good reason (think: testable material).
a. public static void detectWord(String[] stringArr, String key)
i. Using command line arguments, from the main method, pass a string array to detectWord. This method will then prompt the user to search for a word in the array (using a Scanner). detectWord will then print a meaningful statement about the inclusion of that word in the string array. (Helpful built in methods: equals)
b. public static void containsNumber(String[] stringArr)
i. Again using command line arguments, from the main method, pass a string array of unknown length to containsNumber. Then, for each index in the array, print to the screen whether that string contains a numeric digit. (Helpful built in methods: isDigit, charAt)
c. public static void readWrite(Scanner scnr)
i. Using a Scanner object created in the main method, and passed to this method, prompt the user to input a string that contains at least one whitespace character (i.e., , or \t). Continue to prompt the user for correct input if they do not follow this instruction. Then, remove all whitespaces, and print the users string without spaces. (Helpful built in methods: next, nextLine, indexOf, replace)
d. public static String[] alphaOrder(String[] stringArr)
i. From the main method, pass a string array of length 2 to alphaOrder. This method will then put the two strings in alphabetical order, concatenate them with no whitespace, and print the resulting string to the screen.Your main method should prompt the user to input two strings and store them in an array to pass to alphaOrder; in this way, you can avoid having to include an error check. (Helpful built-in methods: compareTo, concat)
e. public static void nameGame(Scanner scnr)
i. Taking user input from the keyboard, replace the first letter of the inputted name with Banana-fana fo-f and print it to the screen (e.g., if Arielle is inputted: output: Banana-fana fo-frielle). (Helpful built-in methods: substring, concat).
3. Your main method may look like this (it need not be identical, but the following is provided as a guide):
public static void main(String[] args){
// Command line args methods:
System.out.println("Enter a string to search for in your input array.");
String key = scnr.next();
detectWord(args,key);
containsNumber(args);
// Methods that use keyboard inputted strings
Scanner scnr = new Scanner(System.in);
readWrite(scnr);
System.out.println("Enter two strings to put in alphabetical order:");
String[] alphaArray = new String[2];
System.out.println("Enter the first string:");
alphaArray[0] = scnr.nextLine();
System.out.println("Enter the second string:");
alphaArray[1] = scnr.nextLine();
alphaOrder(alphaArray);
nameGame(scnr);
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
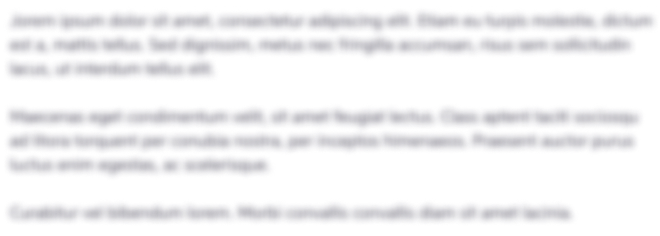
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started