Question
1 Overview You are required to write an interactive program that reads commands from the user, executes the commands, and displays results (if necessary) to
1 Overview
You are required to write an interactive program that reads commands from the user, executes the commands, and displays results (if necessary) to the user. The program must continue to read and process commands from the user until the exit command is given. If an unrecognized command is given, then the program should print the error message: Unrecognized Command, and continue to read input.
Your program is required to maintain a linked list where each node contains the following data:
1. Task: A string representing a task to be completed. Throughout this application, you may assume that each task is described by a string containing no white space characters.
2. Date: A date for which the task must be completed by. Details regarding the format for dates can be found below.
3. Complete: A Boolean eld that indicates if the task has been completed or not.
4. Completed Date: A eld that indicates the date that the task was completed. If the task is active, then this eld should remain null.
Thus, your program implements a to-do list application. The program tracks tasks to be completed, the date when the task must be completed by, and if the task has yet been completed. The commands (described below) are used to add tasks to the list, mark a task as completed, update the deadline of a task, and so on. Additionally, your program will also support saving the list of tasks to a le so that the list can be recreated for successive runs of the program. The linked list must also satisfy the following important property:
Property 1: All of the tasks contained in the list are unique. Furthermore, for all applicable input, your program should be case insensitive. For example, the command ins should be recognized also as INS or Ins. This also implies that task names are case insensitive.
2 Commands
The following is a description of the commands that your program must support:
1. Insert Task Command: The syntax for the insert task command is as follows:
ins str date
where ins is the name of the command, str is the name of the task being inserted into the list, and date represents a date where the task should be completed by, i.e. the deadline of the task. The behavior of the ins command is described by the following cases:
(a) Case I: If the task described by str is already contained in the list, but the deadline date indicated is dierent, then the program should update the date eld of the already existing node to be the new date. The rest of the list should remain unchanged. Note: If the dates are the same, then no change to the node should take place.
(b) Case II: If the task described by str is not contain in the list, then a new node should be created and added to the list. The deadline for this new task is indicated by date.
Page 1 of 4
Nota Bene: In any case, the ins command does not produce any output.
2. Complete Task Command: The syntax for the complete task command is as follows:
com str
where com is the name of the command and str is the name of a task. If the task indicated by str is contained in the list, then the application should mark that task as completed by updating the necessary elds, i.e. the completed eld and the completed date eld. The completed date eld should be set to the current day. If the task is not contained in the list, then the program should display an error message: No such task..
3. Print List Command: The syntax for the print list command is given as follows:
plt
where plt is the name of the command. If the user issues this command, then the program should print every task currently contained in the list along with the deadline, and if the task is completed or not. Each task should be displayed on a line by itself in the following format:
Name, Deadline, Completed
where Name is the name of the task, Deadline is the deadline of the task, and Completed is yes if the task is completed, or no if the task has not yet been completed.
4. Clean List Command: The syntax for this command is as follows:
cln
where cln is the name of the command. The clean command must remove from the list every completed task. The remaining tasks should not be modied.
5. Todays Tasks Command: The syntax for this command is as follows:
tdy
where tdy is the name of the command. If the user enters this command, then the program should only display the tasks with deadline matching the current date. The output format is the same as that for the print list command.
6. Save List Command: The syntax for the save list command is as follows:
sve
where sve is the name of the command. If the user enters this command, then the current list of tasks must be saved to a text le called tasks.dat. The le should contain all of the information necessary to reconstruct the task list, i.e. all of the data for each task.
7. Load List Command: The syntax for the load list command is as follows:
lod
where lod is the name of the command. If the user enters this command, then the program should build a linked list of tasks using the tasks.dat le. The behavior of this command is described by the following cases:
Page 2 of 4
(a) Case I: If the tasks.dat le does not exist or is empty, then the program should display an appropriate error message and continue processing further commands. The list maintained by the program should not be modied.
(b) Case II: If the tasks.dat le exists and contains information for a task list, then the program should construct a new linked list of tasks corresponding to the le. The current linked list of tasks should be overridden.
8. Exit Command: The syntax for the exit command is as follows:
ext
where ext is the name of the command. If the user issues this command, then the program should shut down.
3 Information Regarding Dates
Your program must use the Java Date library and necessary supporting libraries for maintaining dates. Information regarding this class can be found here:
https://docs.oracle.com/javase/7/docs/api/java/util/Date.html
The format for input and output of dates is given by the following pattern:
YYYY-MM-DD
where Y Y Y Y indicates a year using four digits, e.g. 2019, MM indicates a numeric month using two digits, e.g. February is indicated by 02 and October is indicated by 10, and DD is two digit numeric day, i.e. the fth of the month is indicated by 05. For example, the date February 15, 2019 is represented by the string:
2019-02-15
You may assume that all dates presented as input are in the correct format and are valid dates. In particular, you do not need to perform any error checking on dates given as input. However, you must ensure that all dates written as output are in the above format. No other information regarding the date need be displayed or accounted for.
4 Program Outline
The following is a general summary of the behavior of the program:
1. Read first command from the user
2. WHILE (command != end) {
3. process command
4. execute command
5. display output (if any)
6. read next command
7. }
5 Structural Requirements
Your program is required to implement at least the three following classes. The class names must be exactly as indicated in each description:
1. Driver Class: This class should handle reading the users input, parsing the users input, and constructing a linked list object. The name of this class must be TaskDriver.
Page 3 of 4
2. Task Node Class: This class should represent a node of the linked list. It must contain elds for the relevant data and appropriate getters and setters. The name of this class must be TaskNode.
3. Task List Class: This class should represent the linked list of tasks. The eld for the head pointer should be contained here; along with all of the methods needed to execute the commands described in the commands section above. The name of this class must be TaskList.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
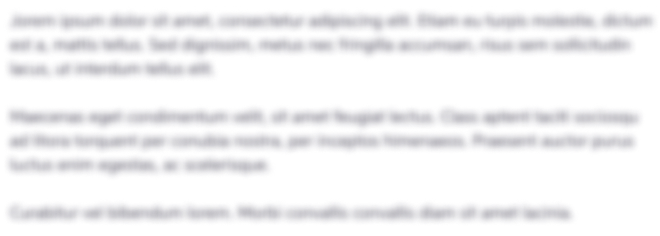
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started