Question
1. Problem: Create a Java application that implements an online store complete with shopping cart and check out procedure. To clarify the problem, I have
1. Problem: Create a Java application that implements an online store complete with shopping cart and check out procedure. To clarify the problem, I have divided it into 4 parts. Part 1: Create Store Inventory. The program begins by creating an online store inventory from a list of items stored in a text file. A try-catch block must be used to handle checked exceptions.
Part 2: Create/Add to/Remove from Shopping Cart. After creating the store inventory, the program welcomes the customer, then provides him/her with a menu of options for creating a shopping cart, adding items to or removing items from the shopping cart, or checking out. Creating a shopping cart entails creating an empty shopping cart. Adding an item to the shopping cart entails searching the store inventory first by category then by item to locate an item to add. (If the customer chooses to add an item that is already in the cart, simply increase the quantity by one.) Removing an item from the shopping cart entails displaying the current contents of the shopping cart, then prompting the customer to enter the number of the item to remove. The shopping cart must be displayed after each addition or removal. All customer input must be validated. try-catch blocks must be used to handle unchecked exceptions. The program continues to display the main menu until the customer decides to check out.
Part 3: Check Out. Once the customer selects check out, the program displays a summary of the customers order. Next, the program asks the customer how s/he wishes to pay for the order. If the customer chooses 1 to charge to a credit card on file, the program simply displays the payment summary. If the customer chooses 2 to charge to a new credit card, the program prompts the customer to enter payment information (card holder full name, followed by card type, number, and expiration date), then displays the credit payment summary. Both payment summaries must be formatted as shown in the sample runs. The customers choice of payment option must be validated. With respect to the credit card information, you need only check that the card number consists of 16 digits. try-catch blocks must be used to handle unchecked exceptions.
Part 4: Thank Customer. Finally, the program thanks the customer for visiting the online store. Implementation Requirements and Guidelines: o All items must be stored in and read from a text file. An example of recommended formatting for the text file appears at the end of this document. o Java code that accesses the text file must be enclosed in a try-catch block. Minimally, your program must handle the FileNotFoundException. o You must use a minimum of two classes to implement this program: a driver containing main and an Item class. Important Note: Each Java class must be in a separate *.java file. o The store inventory and the shopping cart must each be implemented as an ArrayList of Item objects. o An Item object must have 5 attributes: item number (int), item name (String), item category (String), item price (double), and item quantity (int; initialize to 1). o Required methods for the Item class include: o A five-argument constructor that initializes the five instance variables (specified above). o set and get methods for each of the five instance variables. o A toString method to generate nicely formatted output. o The store inventory must contain at least 15 items from 3 or more categories with 3 or more items per category. o As usual, your program output should strongly resemble the sample runs at the end of this document. Pay particular attention to the handling of invalid input.
Sample Runs:
Sample run #1 (Customer correctly creates a shopping cart, adds items to it, then checks out.): run: Creating inventory ... /************************************************************************************************/ Welcome to Barnes & Noble Online! Choose an option. 1) Create empty shopping cart 2) Add item to shopping cart 3) Remove item from shopping cart 4) Check out Your choice? 1 /************************************************************************************************/ Shopping cart created! /************************************************************************************************/ Welcome to Barnes & Noble Online! Choose an option. 1) Create empty shopping cart 2) Add item to shopping cart 3) Remove item from shopping cart 4) Check out Your choice? 2 /************************************************************************************************/ Choose a category. 1) Books 2) Home & Gifts 3) Movies & TV 4) Music Your choice? 3 600 Bones: Season 12 $19.99 601 Logan $17.99 602 The Polar Express $ 9.99 Enter the number of the item you wish to add. Your choice? 600 Your choice "600 Bones: Season 12" has been added to the shopping cart. Number: 600 Name: Bones: Season 12 Category: Movies & TV Price: $ 19.99 Quantity: 1 /************************************************************************************************/ Welcome to Barnes & Noble Online! Choose an option. 1) Create empty shopping cart 2) Add item to shopping cart 3) Remove item from shopping cart 4) Check out Your choice? 2 /************************************************************************************************/ Choose a category. 1) Books 2) Home & Gifts 3) Movies & TV 4) Music Your choice? 2 800 Doctor Who TARDIS Bookends Set of 2 $51.95 801 Wallace Plaid Mini Umbrella $14.95 802 WonderFlex Light, Black $18.95 Enter the number of the item you wish to add. Your choice? 801 Your choice "801 Wallace Plaid Mini Umbrella" has been added to the shopping cart. Number: 600 Name: Bones: Season 12 Category: Movies & TV Price: $ 19.99 Quantity: 1 Number: 801 Name: Wallace Plaid Mini Umbrella Category: Home & Gifts Price: $ 14.95 Quantity: 1 /************************************************************************************************/ Welcome to Barnes & Noble Online! Choose an option. 1) Create empty shopping cart 2) Add item to shopping cart 3) Remove item from shopping cart 4) Check out Your choice? 3 /************************************************************************************************/ Displaying the current contents of the shopping cart ... Number: 600 Name: Bones: Season 12 Category: Movies & TV Price: $ 19.99 Quantity: 1 Number: 801 Name: Wallace Plaid Mini Umbrella Category: Home & Gifts Price: $ 14.95 Quantity: 1 Enter the number of the item you wish to remove. Your choice? 801 Item #801 has been removed from the shopping cart. Displaying the current contents of the shopping cart ... Number: 600 Name: Bones: Season 12 Category: Movies & TV Price: $ 19.99 Quantity: 1 /************************************************************************************************/ Welcome to Barnes & Noble Online! Choose an option. 1) Create empty shopping cart 2) Add item to shopping cart 3) Remove item from shopping cart 4) Check out Your choice? 2 /************************************************************************************************/ Choose a category. 1) Books 2) Home & Gifts 3) Movies & TV 4) Music Your choice? 1 100 Doctor Who: Deep Time $ 8.47 101 Doctor Who: Heart of Stone $ 9.99 102 The Hitchhiker's Guide to the Galaxy $ 7.99 Enter the number of the item you wish to add. Your choice? 100 Your choice "100 Doctor Who: Deep Time" has been added to the shopping cart. Number: 600 Name: Bones: Season 12 Category: Movies & TV Price: $ 19.99 Quantity: 1 Number: 100 Name: Doctor Who: Deep Time Category: Books Price: $ 8.47 Quantity: 1 /************************************************************************************************/ Welcome to Barnes & Noble Online! Choose an option. 1) Create empty shopping cart 2) Add item to shopping cart 3) Remove item from shopping cart 4) Check out Your choice? 2 /************************************************************************************************/ Choose a category. 1) Books 2) Home & Gifts 3) Movies & TV 4) Music Your choice? 1 100 Doctor Who: Deep Time $ 8.47 101 Doctor Who: Heart of Stone $ 9.99 102 The Hitchhiker's Guide to the Galaxy $ 7.99 Enter the number of the item you wish to add. Your choice? 100 Your choice "100 Doctor Who: Deep Time" has been added to the shopping cart. Number: 600 Name: Bones: Season 12 Category: Movies & TV Price: $ 19.99 Quantity: 1 Number: 100 Name: Doctor Who: Deep Time Category: Books Price: $ 8.47 Quantity: 2 /************************************************************************************************/ Welcome to Barnes & Noble Online! Choose an option. 1) Create empty shopping cart 2) Add item to shopping cart 3) Remove item from shopping cart 4) Check out Your choice? 4 /************************************************************************************************/ ORDER SUMMARY: Number: 600 Name: Bones: Season 12 Category: Movies & TV Price: $ 19.99 Quantity: 1 Number: 100 Name: Doctor Who: Deep Time Category: Books Price: $ 8.47 Quantity: 2 Order Total: $ 39.15 (6.0% tax included) /************************************************************************************************/ How do you wish to pay for your order? (Enter 1 to charge to credit card on file or 2 to charge to new credit card.): 2 Please enter your payment information: Card holder name: Jane Wymark Credit card type (e.g., MasterCard): Visa Credit card number (e.g., 5201345098756420): 4321009854761189 Expiration date (e.g., 10/2016): 08/2021 /************************************************************************************************/ Credit payment summary: Customer name: Jane Wymark Payment amount: $ 39.15 Card type: Visa Card number: ************1189 Exp date: 08/2021 /************************************************************************************************/ Thanks for shopping at Barnes & Noble Online. Please come back soon! /************************************************************************************************/ BUILD SUCCESSFUL (total time: 5 minutes 29 seconds)
Sample run #2 (Customer makes a variety of invalid choices while shopping but finally checks out.): run: Creating inventory ... /************************************************************************************************/ Welcome to Barnes & Noble Online! Choose an option. 1) Create empty shopping cart 2) Add item to shopping cart 3) Remove item from shopping cart 4) Check out Your choice? 2 /************************************************************************************************/ Please create a shopping cart! /************************************************************************************************/ Welcome to Barnes & Noble Online! Choose an option. 1) Create empty shopping cart 2) Add item to shopping cart 3) Remove item from shopping cart 4) Check out Your choice? 1 /************************************************************************************************/ Shopping cart created! /************************************************************************************************/ Welcome to Barnes & Noble Online! Choose an option. 1) Create empty shopping cart 2) Add item to shopping cart 3) Remove item from shopping cart 4) Check out Your choice? 2 /************************************************************************************************/ Choose a category. 1) Books 2) Home & Gifts 3) Movies & TV 4) Music Your choice? 5 5 is not a valid choice! Please enter a number from 1 to 4. Enter 0 to return to the main menu. Your choice? 4 500 Acoustic Christmas $31.34 501 Hamilton (Original Broadway Cast Recording) $22.39 502 Les Miserables (Original Broadway Cast) $30.16 Enter the number of the item you wish to add. Your choice? 503 Item #503 was not found in the inventory. /************************************************************************************************/ Welcome to Barnes & Noble Online! Choose an option. 1) Create empty shopping cart 2) Add item to shopping cart 3) Remove item from shopping cart 4) Check out Your choice? 2 /************************************************************************************************/ Choose a category. 1) Books 2) Home & Gifts 3) Movies & TV 4) Music Your choice? 4 500 Acoustic Christmas $31.34 501 Hamilton (Original Broadway Cast Recording) $22.39 502 Les Miserables (Original Broadway Cast) $30.16 Enter the number of the item you wish to add. Your choice? 502 Your choice "502 Les Miserables (Original Broadway Cast)" has been added to the shopping cart. Number: 502 Name: Les Miserables (Original Broadway Cast) Category: Music Price: $ 30.16 Quantity: 1 /************************************************************************************************/ Welcome to Barnes & Noble Online! Choose an option. 1) Create empty shopping cart 2) Add item to shopping cart 3) Remove item from shopping cart 4) Check out Your choice? 2 /************************************************************************************************/ Choose a category. 1) Books 2) Home & Gifts 3) Movies & TV 4) Music Your choice? 1 100 Doctor Who: Deep Time $ 8.47 101 Doctor Who: Heart of Stone $ 9.99 102 The Hitchhiker's Guide to the Galaxy $ 7.99 Enter the number of the item you wish to add. Your choice? 101 Your choice "101 Doctor Who: Heart of Stone" has been added to the shopping cart. Number: 502 Name: Les Miserables (Original Broadway Cast) Category: Music Price: $ 30.16 Quantity: 1 Number: 101 Name: Doctor Who: Heart of Stone Category: Books Price: $ 9.99 Quantity: 1 /************************************************************************************************/ Welcome to Barnes & Noble Online! Choose an option. 1) Create empty shopping cart 2) Add item to shopping cart 3) Remove item from shopping cart 4) Check out Your choice? four For input string: "four" is not a valid format for an integer. Please re-enter your choice. Your choice? 4 /************************************************************************************************/ ORDER SUMMARY: Number: 502 Name: Les Miserables (Original Broadway Cast) Category: Music Price: $ 30.16 Quantity: 1 Number: 101 Name: Doctor Who: Heart of Stone Category: Books Price: $ 9.99 Quantity: 1 Order Total: $ 42.56 (6.0% tax included) /************************************************************************************************/ How do you wish to pay for your order? (Enter 1 to charge to credit card on file or 2 to charge to new credit card.): 3 3 is not a valid payment option. Please enter 1 to charge to card on file or 2 to charge to new card. How do you wish to pay for your order? (Enter 1 to charge to credit card on file or 2 to charge to new credit card.): 1 Payment summary: Payment amount: $ 42.56 charged to card on file /************************************************************************************************/ Thanks for shopping at Barnes & Noble Online. Please come back soon! /************************************************************************************************/ BUILD SUCCESSFUL (total time: 8 minutes 28 seconds)
Step by Step Solution
There are 3 Steps involved in it
Step: 1
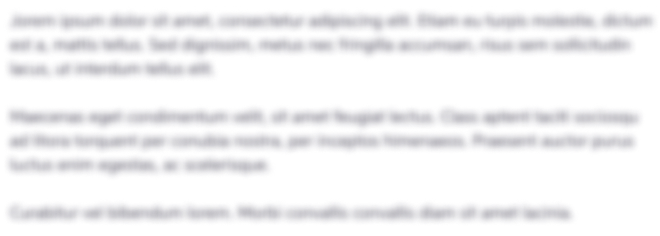
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started