Question
1 Problem Description To complete the implementation of the Mystring class, the declaration of which is provided in Mystring.h file (this file is an expansion
1 Problem Description
To complete the implementation of the Mystring class, the declaration of which is provided in Mystring.h file (this file is an expansion to the Mys- tring.h file of Lab 6 by adding more methods and operators and some global functions and operators). The Mystring class mimics the behavior of C++ string class and all methods and operators of Mystring class are basically identical to (behave the same as) the corresponding methods and operators of the C++ string class.
To find out the behavior of each method to be implemented, you are expected and encouraged to experiment with the corresponding method in C++ string class or to ask questions in the lecture. You may use any of the c-style string library functions, such as strcpy, in your implementation. However, you SHOULD NOT use C++ string!! The reason is that we assume such a class is not available and therefore we are going to implement it. Vector or any class of data structures in C++ SHALL NOT be used either.
The subsections 4.1 to 4.3 help us to understand the assignment operator and provide hints as to how to implement it (the first version where a string is assigned to another string. Note the other version is a cstring is assigned to a string). The files being referred to in the following discussion are Lab 6 files. You might review Lab 6 and think of the following as an extension.
4.1 Testing using C++ string for assignment operator
1. Comment out the line #define string Mystring in main.cpp 2. Addthefollowingbeforecout << "Lab 6 ends" << endl;statement
cout << "---Testing assignment operator--- "; { string s2; s2=s1; string s2name("s2"); check(s2,s2name); } check(s1,s1name);
We should get the following output or something similar (perhaps a larger max size() value):
This is Lab 6 checking s1 s1 contains Hello, World! s1 capacity() is 13 s1 length() is 13 s1 size() is 13 s1 max_size() is 1073741820 checking s1 s1 contains Hello, World! s1 capacity() is 13 s1 length() is 13 s1 size() is 13 s1 max_size() is 1073741820 ---Testing assignment operator--- checking s2 s2 contains Hello, World! s2 capacity() is 13 s2 length() is 13 s2 size() is 13 s2 max_size() is 1073741820 checking s1 s1 contains Hello, World! s1 capacity() is 13 s1 length() is 13 s1 size() is 13 s1 max_size() is 1073741820 Lab 6 ends Press [Enter] to close the terminal ...
4.2 Testing the use of Mystring default assignment operator provided by the compiler
1. Uncomment the line #define string Mystring in main.cpp
2. compile and run the same program
Do we get the same output as the previous step? Do we get a nasty run time error? Please try to explain why we get a different output in your report.
4.3 Implement assignment operator for Mystring class
The following provides the pseudo code or hint about how to implement the assignment operator for Mystring class as well as how to adjust the current code base. You may also study the textbook (in particular page 78).
1. Uncomment the first assignment operator prototype line in Mystring.h
2. Uncomment the code for assignment operator implementation in Mys- tring.cpp
3. If the object (left hand side of =, and "this" is the pointer to it) that invokes the assignment operator (note = is a member function conceptually) is the same as the argument object (right hand side of =, the orig argument), for example as in (where x is Mystring type) x=x, do nothing and otherwise perform the next two steps.
4. Free the space pointed to by ptr_buffer
5. Allocation space based on argument object (orig) and adjust values of the members (similar to copy constructor code)
6. The last statement is return *this
7. Compile and run the same program
We should get the following:
This is Lab 6 checking s1 s1 contains Hello, World! s1 capacity() is 14 s1 length() is 13 s1 size() is 13 s1 max_size() is 1073741820 checking s1 s1 contains Hello, World! s1 capacity() is 14 s1 length() is 13 s1 size() is 13 s1 max_size() is 1073741820 ---Testing assignment operator--- checking s2 s2 contains Hello, World! s2 capacity() is 14 s2 length() is 13 s2 size() is 13 s2 max_size() is 1073741820 checking s1 s1 contains Hello, World! s1 capacity() is 14 s1 length() is 13 s1 size() is 13 s1 max_size() is 1073741820 Lab 6 ends Press [Enter] to close the terminal ...
4.4 Implement the remaining methods or operators
Implement all the member functions, operators, as well as non- member operators, declared in Mystring.h, except for the iterator methods begin and end. The implementation goes to Mystrng.cpp. You should study the relationship between the methods and think about how some operation might be implemented by other operation(s). The idea is to reuse the code by calling other methods (an example could be homework 3 set method and constructor). Note that some methods may be implemented by calling other method(s) with a bit of additional logic. You should write the code for one method at a time, debugging and testing.
Mystring.h
#ifndef MYSTRING_H #define MYSTRING_H #include #include #include using namespace std; class Mystring { public: // types with scope in this class typedef unsigned int size_type; typedef char* iterator; typedef const char* const_iterator; static const long int npos = 1073741824; // Default constructor, code given to you in lab6 Mystring.cpp Mystring(); // Other constructors, code given to you in lab6 Mystring.cpp Mystring(const char *); // Copy constructor, done in lab6 Mystring(const Mystring& orig); // Destructor, code given to you in lab6 Mystring.cpp ~Mystring(); // Iterators We will not implement them for hw4 iterator begin(); iterator end(); //=== Memory Related === // Change buffer size to n void reserve(size_type n); size_type size() const; // code given to you in lab6 Mystring.cpp size_type length() const; //code given to you in lab6 Mystring.cpp size_type capacity() const; //code given to you in lab6 Mystring.cpp size_type max_size() const; //code given to you in lab6 Mystring.cpp bool empty() const; //=== Overloading operators === // Assignment operator // the next two lines are commented out, so to use default. Uncomment to implement both later //Mystring& operator=(const Mystring&); //Mystring& operator=(const char *); // Array notation char operator[](size_type pos) const; // minor difference we use char and not const char& char& operator[](size_type pos); // Append Mystring& operator+=(const Mystring& str); Mystring& operator+=(const char * str); //=== Methods that modify the string void clear(); void push_back(char c); // substring (2) Mystring& append(const Mystring& str, size_type subpos, size_type sublen); // buffer (4) Mystring& append(const char * str, size_type n); // string (1) Mystring& insert(size_type pos, const Mystring& str); // c-string (3) Mystring& insert(size_type pos, const char * str); // string (1) Mystring& replace(size_type start, size_type span, const Mystring& str); // c-string (3) Mystring& replace(size_type start, size_type span, const char * str); //=== Conversion to c-string const char * c_str() const; // code given to you in lab6 Mystring.cpp //=== functionalities similar to homework 1, quizzes, exams // buffer (3) version of the overloaded method size_type find_first_of (const char* s, size_type pos, size_type n) const; // c-string (2) size_type find_last_not_of (const char* s, size_type pos = npos) const; private: // pointer to the memory location where string is stored as a c-style // string char * ptr_buffer; // the size of the memory in terms of bytes or characters size_type buf_size; // number of characters currently in the memory not including the // terminating null character size_type len; }; //=== Overloading global operators for Mystring // Overload operator == for Mystring bool operator==(const Mystring&, const Mystring&); bool operator==(const char *, const Mystring&); bool operator==(const Mystring&, const char *); // Overload operator != for Mystring bool operator!=(const Mystring&, const Mystring&); bool operator!=(const char *, const Mystring&); bool operator!=(const Mystring&, const char *); // Overload operator + for string as concatenation Mystring operator+(const Mystring&, const Mystring&); // Output ostream& operator<<(ostream& out, const Mystring& str); // code given to you in lab6 Mystring.cpp // typedef Mystring::iterator iterator; #endif /* MYSTRING_H */
Mystring.cpp:
#include "Mystring.h" // default constructor Mystring::Mystring() { ptr_buffer = new char[1]; *ptr_buffer = '\0'; buf_size = 1; len = 0; } // constructor from c-style string or "abc" Mystring::Mystring(const char * s) { len = strlen(s); buf_size = len + 1; ptr_buffer = new char[buf_size]; strcpy(ptr_buffer, s); } // copy constructor to be implemented Mystring::Mystring(const Mystring& orig) { len = orig.length(); buf_size = len + 1; ptr_buffer = new char[buf_size]; strcpy(ptr_buffer, orig.ptr_buffer); } // one of the over loaded assignment operator if you have time /*Mystring& Mystring::operator=(const Mystring& orig) { }*/ // some simple methods implemented for you Mystring::size_type Mystring::size() const { return len; } Mystring::size_type Mystring::length() const { return len; } Mystring::size_type Mystring::capacity() const { return buf_size; } Mystring::size_type Mystring::max_size() const { return (int)pow(2,30) -4 ; } // Destructor Mystring::~Mystring() { delete [] ptr_buffer; } // Provided for the lab so we may cout mystring ostream& operator<<(ostream& out, const Mystring& str) { out << str.c_str(); return out; } // implemented for the lab to support the implementation of << const char * Mystring::c_str() const { return ptr_buffer; }
Main.cpp
#include #include #include #include "Mystring.h" // comment out to use string class, now use Mystring class #define string Mystring using namespace std; /* * Check function from the previous lab. */ void check (const string s, const string name) { cout << "checking " << name << endl; cout << name << " contains " << s << endl; cout << name << " capacity() is " << s.capacity() << endl; cout << name << " length() is " << s.length() << endl; cout << name << " size() is " << s.size() << endl; cout << name << " max_size() is " << s.max_size() << endl << endl; } /* * Check-obj function is similar to check, except we will check the object * instead of the copy of the object built by the copy constructor. */ void check_obj (const string& s, const string name) { cout << "checking " << name << " the object" << endl; cout << name << " contains " << s << endl; cout << name << " capacity() is " << s.capacity() << endl; cout << name << " length() is " << s.length() << endl; cout << name << " size() is " << s.size() << endl; cout << name << " max_size() is " << s.max_size() << endl << endl; } int main() { cout << "This is Lab 6 "; string s1("Hello, World!"); string s1name ("s1"); check (s1, s1name); check (s1, s1name); cout << "Lab 6 ends "; cout << " --- Testing reserve --- "; check_obj(s1, s1name); s1.reserve(15); check_obj (s1, s1name); cout << "---Testing assignment operator = --- "; s1 = "Hi!"; check_obj(s1, s1name); string s2,s3("abcdefghijklmnopqrstuvwxyz01234567890ABCDEFGHIJKLMNOPQRSTUVWXYZ"); s2 = s1 = s3; check_obj(s1, s1name); check_obj(s2, "s2"); check_obj(s3, "s3"); string s4; check_obj(s4, "s4"); s3 = s4; check_obj(s3, "s3"); cout << "---Testing append --- "; s3.append("CSCE2014", 4); check_obj(s3, "s3"); return 0; }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
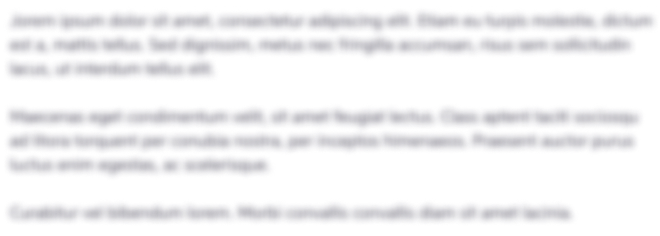
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started