Question
1. Study the following pseudo-code to make sure you understand the basic algorithm for efficiently calculating the number of asserted bits in a given input.
1. Study the following pseudo-code to make sure you understand the basic algorithm for efficiently calculating the number of asserted bits in a given input. // inputs: go, in (WIDTH bits) // outputs: out (clog2(WIDTH+1) bits), done // reset values (add any others that you might need) out = 0; done = 0; while(1) { // wait for go to start circuit while (go == 0); done = 0; count = 0; // store input in register n_r = in; // main algorithm while(n_r != 0) { n_r = n_r & (n_r 1); count ++; } // assign output and assert done output = count; done = 1; } 1-process FSMD 2. Using the provided file asserted_bit_count.sv, fill in the asserted_bit_count_fsmd_1p module with a design that uses the 1-process FSMD model. Done should remain asserted until the application is started again, which is represented by go being asserted while done is asserted. Done should be cleared on the cycle after go is asserted. Use the provided testbench to test your module. Note that the testbench only tests a single module. Therefore, make sure the following line: asserted_bit_count_fsmd_1p #(.WIDTH(WIDTH)) DUT (.*); is uncommented, and that all other modules are commented out. For the other parts of the lab, you will specify a different module. The testbench applies 10002 tests, in addition to testing the correct timing of the done signal. Your design works when there are 10002 successful tests and no other errors report. Note that the done timing checks are not included in the error count and will be reported as separate assertion errors. IMPORTANT: The constructs used to verify the timing of the done signal do not work in the free version of Modelsim. They will compile just fine, but the simulator will simply ignore them. This is dangerous because if you simulate using the free version, you might think your design is working perfectly, only to find out that the timing of done fails when tested in the full version of Modelsim. Make sure to use Modelsim on the class servers to avoid this problem. 2-process FSMD 3. Repeat the same process as the previous step, but using a 2-process FSMD in the asserted_bit_count_fsmd_2p module. Use the provided testbench to test your module. Note that the testbench only tests a single module. Therefore, make sure the following line: asserted_bit_count_fsmd_2p #(.WIDTH(WIDTH)) DUT (.*); is uncommented, and that all other modules are commented out. Datapath 4. In this step, you will create your own datapath to implement the algorithm. Create your own datapath module in a new file (datapath.sv). Implement it however you like (behaviorally, structurally, with any number of resources, etc.). Draw a schematic of the datapath and include it in the report described below. FSM 5. Create a 2-process finite-state machine in a new file (fsm.sv) that works with your custom datapath to implement the algorithm. FSM+D 6. Connect your FSM and datapath together in the asserted_bit_count_fsm_plus_d module of asserted_bit_count.sv. Use the provided testbench to test your module. Note that the testbench only tests a single module. Therefore, make sure the following line: asserted_bit_count_fsm_plus_d #(.WIDTH(WIDTH)) DUT (.*); is uncommented, and that all other modules are commented out. HERE IS the asserted_bit_count.sv // TODO: Put your one 1-process FSMD here. module asserted_bit_count_fsmd_1p #(parameter int WIDTH) ( input logic clk, input logic rst, input logic go, input logic [WIDTH-1:0] in, output logic [$clog2(WIDTH+1)-1:0] out, output logic done ); endmodule // TODO: Put your one 2-process FSMD here. module asserted_bit_count_fsmd_2p #(parameter int WIDTH) ( input logic clk, input logic rst, input logic go, input logic [WIDTH-1:0] in, output logic [$clog2(WIDTH+1)-1:0] out, output logic done ); endmodule // TODO: Put your FSM+D here. Add your datapath and FSM modules in other files. module asserted_bit_count_fsm_plus_d #(parameter int WIDTH) ( input logic clk, input logic rst, input logic go, input logic [WIDTH-1:0] in, output logic [$clog2(WIDTH+1)-1:0] out, output logic done ); endmodule // Top-level for synthesis. Change the comments to synthesize each module. module asserted_bit_count #(parameter int WIDTH=32) ( input logic clk, input logic rst, input logic go, input logic [WIDTH-1:0] in, output logic [$clog2(WIDTH+1)-1:0] out, output logic done ); asserted_bit_count_fsmd_1p #(.WIDTH(WIDTH)) top (.*); //asserted_bit_count_fsmd_2p #(.WIDTH(WIDTH)) top (.*); //asserted_bit_count_fsm_plus_d #(.WIDTH(WIDTH)) top (.*); endmodule
Step by Step Solution
There are 3 Steps involved in it
Step: 1
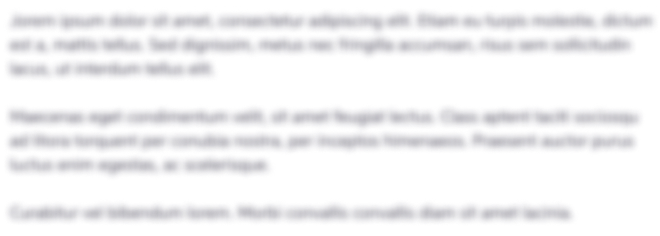
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started