Question
1. The class Rectangle includes the void methods: calcArea and calcPerimeter. The calcArea method takes two integer parameters for length and width, calculates the area
1. The class Rectangle includes the void methods: calcArea and calcPerimeter. The calcArea method takes two integer parameters for length and width, calculates the area of a rectangle, and assigns the value to a private instance variable. The calcPerimeter method takes two integer parameters for length and width, calculates the perimeter of a rectangle, and assigns the value to a private instance variable. The Rectangle class also includes two getter methods, which return the calculated values. The Rectangle class implements the Calculatable interface, which also contains calcArea and calcPerimeter. Write the Rectangle class and the Calculatable interface.
2. Write an abstract method resetValue that takes an integer parameter and returns a double value.
3. Write an abstract method convertValue that takes an integer parameter and returns a double value.
4. Write a Calculatable interface that includes the following methods. How the methods may be used by other classes to calculate is described below. (Do not overanalyze these methods!) The volume of a cube. The volume of a cube is determined by the length, height, and width. The result is returned. The volume of a cylinder. The volume of a cylinder is determined by the height and radius of a cylinder. The result is returned.
5. Consider the following partially-completed class: public class SomeClass extends SomeOtherClass { public int methodA(double m) { //some code here } public void methodB(double n) { //some code here } } Write the corresponding abstract class containing the two methods.
6. Consider the following partially-complete class: public class SomeClass implements Comparable { private String lastName; private String firstName; public SomeClass( String lastName, String firstName ) { this.lastName = lastName; this.firstName = firstName; } public String getLastName() { return this.lastName; } public String getFirstName() { return this.firstName; } public int compareTo( SomeClass obj ) { // complete the code here } } The SomeClass implements the Comparable interface. Complete SomeClass by writing a compareTo method that takes one parameter. It should return 1 if the private instance variable lastName occurs lexicographically before the passed value, 0 if the private instance variable lastName and the parameter value are lexicographically the same, or 1 if the private instance variable lastName occurs lexicographically after the parameter value.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
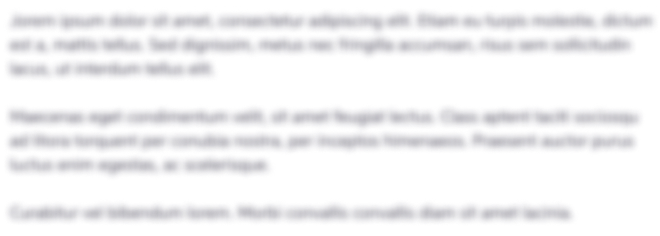
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started