Question
1) Use the LinkedStack class to support an application that tracks the status of an online auction. Bidding begins at 1 (dollars, pounds, euros, or
1) Use the LinkedStack class to support an application that tracks the status of an online auction. Bidding begins at 1 (dollars, pounds, euros, or whatever) and proceeds in increments of at least 1. If a bid arrives that is less than the current bid, it is discarded. If a bid arrives that is more than the current bid, but less than the maximum bid by the current high bidder, than the current bid for the current high bidder, is increased to match it and the new bid is discarded. If a bid arrives that is more than the maximum bid for the current high bidder, then the new bidder becomes the current high bidder, at a bid of one more than the previous high bidder's maximum. When the auction is over (the end of the input is reached), a history of the actual bids (the ones not discarded), from high bid to low bid, should be displayed. For example:
New bid Result High Bidder High Bid Maximum Bid 7 John New high bidder John 1 7 5 Hank High bid increased John 5 7 10 Jill New high bidder Jill 8 10 8 Thad No change Jill 8 10 15 Joey New high bidder Joey 11 15
The bid history for this auction would be Joey 11 Jill 8 John 5 John 1
Input/output details can be determined by you or your instructor. In any case, as input proceeds, the current status of the auction should be displayed. The final output should include the bid history as described above.
LinkedStackClass
//---------------------------------------------------------------------- // LinkedStack.java by Dale/Joyce/Weems Chapter 2 // // Implements StackInterface using a linked list to hold the elements. //----------------------------------------------------------------------- package ch02.stacks; import support.LLNode; public class LinkedStack
Step by Step Solution
There are 3 Steps involved in it
Step: 1
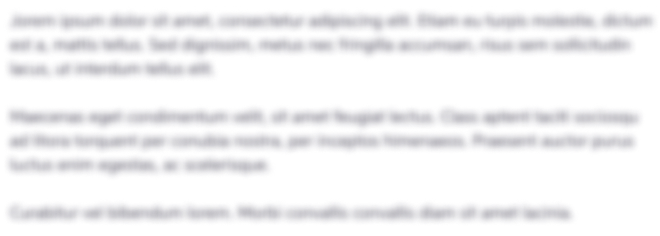
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started