Question
1. What is printed when the following statement is executed? System.out.println(17 / 5 % 3 + 17 * 5 % 3); 1 4 9 42
1. What is printed when the following statement is executed?
System.out.println(17 / 5 % 3 + 17 * 5 % 3); |
-
1
-
4
-
9
-
42
-
42.5
2. Consider the following method.
public void process(String s) { s = s.substring(2, 3) + s.substring(1, 2) + s.substring(0, 1); } |
What is printed as a result of executing the following statements (in a method in the same class)?
String s = "ABCD"; process(s); System.out.println(s); |
-
ABCD
-
CBA
-
CDBCA
-
CDBCAB
-
IndexOutOfBoundsException
3.
Suppose m is a two-dimensional array of size 4 by 4, with all its elements intialized to zeroes. What will be the values stored in m after fill(m) is called? The method fill is defined as follows:
public void fill(int[][] m) { int n = m.length; for (int i = 1; i < n - 1; i++) { for (int j = 1; j < n - 1; j++) m[i][j] = 1; } } |
-
0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0
-
1 1 0 0 1 1 0 0 0 0 0 0 0 0 0 0
-
0 0 0 0 0 1 1 0 0 1 1 0 0 0 0 0
-
1 1 1 0 1 1 1 0 1 1 1 0 0 0 0 0
-
1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1
4.
Which of the following statements will result in a syntax error?
-
String x = "123";
-
Integer x = "123";
-
Object x = "123";
-
String[] x = {"123"};
-
All of the above will compile with no errors.
-
What is printed as a result of executing the following statements?
double x = 2.5, y = 1.99; System.out.println((int)(x/y) + (int)(x*y));
-
0
-
3
-
4
-
4.0
-
5
-
-
Which of the following expressions is true if and only if NOT all three variables a, b, and c have the same value?
-
a != b && b != c
-
a != b || b != c
-
a >= b && b >= c && c >= a
-
a > b || b > c || a > c
-
!(a == b || b == c || a == c)
-
-
What is the result when the following code segment is compiled and executed?
int m = 4, n = 5; double d = Math.sqrt((m + n)/2); System.out.println(d);
-
Syntax error "sqrt(double) in java.lang.Math cannot be applied to int"
-
1.5 is displayed
-
2.0 is displayed
-
2.1213203435596424 is displayed
-
ArithmeticException
-
-
For which of the following pairs of values a and b does the expression
(a > 20 && a < b) || (a > 10 && a > b)
evaluate to true?
-
5 and 0
-
5 and 10
-
15 and 10
-
15 and 20
-
None of the above
-
-
What is printed as a result of executing the following code segment?
List
lst = new ArrayList (); for (int k = 1; k <= 6; k++) lst.add(new Integer(k)); for (int k = 0; k < 3; k++) { Integer i = lst.remove(k); lst.add(i); } for (Integer i : lst) System.out.print(i) -
123456
-
456123
-
456321
-
246135
-
IndexOutOfBoundsException
-
-
Which of the following methods are equivalent (always return the same value for the same values of input parameters)?
public boolean fun(int a, int b, int c) { if (a >= b) if (b >= c) return true; else return false; else return false; }
public boolean fun(int a, int b, int c) { if (a >= b && b >= c) return true; else return false; }
public boolean fun(int a, int b, int c) { return a >= b || b >= c; }
-
I and II only
-
I and III only
-
II and III only
-
All three are equivalent
-
All three are different
-
Consider the following class.
public class Matrix { private int[][] m; /** Initializes m to a square n by n array with all * the elements on the diagonal m[k][k] equal to 0 and * all other elements equal to 1 */ public Matrix(int n) { m = new int[n][n]; < missing code > } < other constructors and methods not shown > }
Which of the following could replace < missing code > in Matrix's constructor, so that it compiles with no errors and works as specified?
for (int r = 0; r < n; r++) for (int c = 0; c < n; c++) m[r][c] = 1; for (int k = 0; k < n; k++) m[k][k] = 0;
for (int k = 0; k < n; k++) m[k][k] = 0; for (int r = 0; r < n; r++) for (int c = 0; c < n; c++) if (r != c) m[r][c] = 1;
for (int c = 0; c < n; c++) for (int r = 0; r < n; r++) if (r == c) m[r][c] = 0; else m[r][c] = 1;
-
I only
-
II only
-
I and II only
-
II and III only
-
I, II and III
-
Which of the following statements about developing a Java program is FALSE?
-
The main purpose of testing a Java program is to make sure it doesn't generate run-time exceptions.
-
Hello.class, a Java class file obtained by compiling Hello.java under a Windows operating system can be executed on a Mac computer.
-
The size of an int variable in Java is four bytes, the same under any operating system and computer model.
-
At run time, int values are represented in the computer memory in the binary number system.
-
Division of an integer value by zero in a Java program will cause a run-time ArithmeticException.
-
Step by Step Solution
There are 3 Steps involved in it
Step: 1
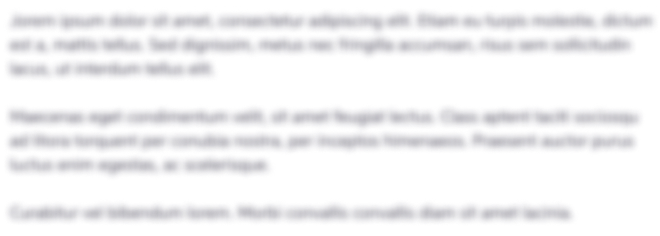
Get Instant Access with AI-Powered Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started