Answered step by step
Verified Expert Solution
Question
00
1 Approved Answer
11:10 Search Back Assignment1.docx In Assignment 1, you will design and implement code to (1) display the player ship and the region of space in
11:10 Search Back Assignment1.docx In Assignment 1, you will design and implement code to (1) display the player ship and the region of space in front of it, (2) move the player ship, and (3) detect collisions with a single asteroid. In the game, the user will enter text commands to control the mining ship and print a picture of the player ship and the space in front of it. If the player ship collides with the asteroid, the program prints a message and terminates. The user can also terminate the program with the 'q' command at any time. As well, after cach move, the region of space that is displayed in the game will move one column to the right. When running, the game will look as follows: 1--\ IX | 1--/ + --<_l_>/ * C======\ 11:10 I Search Back Assignment1.docx The # symbols should be printed to show the border of the arca being displayed. The shape to the left is the player ship and the shape near the middle is the asteroid. The purpose of this assignment is to ensure that you understand how to use two-dimensional arrays, including defining types using two- dimensional arrays and passing two-dimensional arrays to functions. For Part A, you will develop a module that defines a display buffer. For Part B, you will begin implementing the game. For Part C. you will separate your program into multiple files. The Buffer To prepare for displaying the player ship and the region of space in front of it, a temporary area of memory, known as a buffer, should be used to represent the picture before it is displayed. The buffer is represented by a two-dimensional array of characters. Before drawing each frame, the buffer is initialized to contain all blank (* ") characters. After that, the characters representing the player ship and the asteroid are copied into the buffer. Finally, the buffer is printed on the screen. In computer science terminology, any arca of memory used for temporary storage is known as a buffer, and the technique of using a buffer to hold data temporarily is known as buffering. This technique is commonly used in computer graphics, where colour values for pixels are stored instead of characters. The advantage of buffering in this case is that it is not necessary to work out, on a character-by-character basis, exactly what should be printed. Instead, each item to be shown can simply be placed in the appropriate position in the buffer, without worrying about the order in which the characters will be printed. Displaying Things The player ship and the asteroid will each be represented using a two-dimensional array of characters. The characters in each array will be loaded from a file once when the program is started, and then they are copied to the display buffer whenever the current state of the game is displayed. The "images" for the player ship and asteroid are stored in files named player.txt and asteroid.txt. 11:11 I Search Assignment1.docx Back asteroid are stored in files named player.txt and asteroid.txt. The contents of the player.txt file are as follows: c-<_l_>/ C------ The contents of the asteroid.txt file are as follows: /--\ IXXI \--/ Beginning in Assignment 2, you will replace the XX on the asteroid with a number representing its ore value. Part A: The Buffer Type (12/40 marks] In Part A, you will write the buffer module. You will eventually put these functions in their own module, but for now put all the code in a file named BigMain.cpp. Throughout this course, make sure that you use exactly the specified file names, which are case sensitive. Here, B and M are uppercase and the remaining leters are lowercase. By the end of Part A, you will have created functions for handling the buffer with the following prototypes: void bufferPrint (const Buffer buff); void bufferClear (Buffer buff): void bufferSetcell (Buffer buff, int row, int column, char value); Perform the following steps: 1. Define BUFFER_ROW_COUNT and BUFFER_COLUMN_COUNT as integer constants with values of 20 and 60, respectively. Define BUFFER_EMPTY and BUFFER_BORDER as character constants with values of. and '' respectively. Use typedef to define a Buffer type corresponding to a 2D array of chars with dimensions BUFFER ROW_COUNT and BUFFER_COLUMN_COUNT. - Put these constants and declarations in the BigMain.cpp file outside and before the functions defined in step A2 and subsequent steps. They are global constants and a global type definition. 2. Copy in the function prototypes for bufferPrint, bufferClear, and bufferSetcel1. 1. Implement a bufferClear function that takes a Buffer as a parameter. This function should set the value of each element in the Buffer to BUFFER_EMPTY. 4. Implement a bufferPrint function that takes a Buffer as a parameter. This function should print the contents of the Buffer with a border of BUFFER_BORDER characters around it. Hint: Start by printing a line of BUFFER BORDER characters THC, tor cac lne a 11:11 I Search Back Assignment1.docx - Hint: Start by printing a line of BUFFER_BORDER characters. Then, for each line, print a BUFFER BORDER character, then all the characters in one row of the Buffer, then another BUFFER_BORDER character, and then a newline. Finally, print another line of BUFFER_BORDER characters. 3. Implement a bufferSetcell function that takes a Buffer, two ints representing a row and column, and a char representing the new value, as parameters. This function should set the element of the Buffer specified by the row and column to the character value. If the row and column lie outside the Buffer, there should be no effect. Debugging: To test the Buffer functions, create a nain function. It should first declare a variable of type Buffer named ny_buffer. Then it should call the bufferclear function with my_buffer as a parameter and then call bufferPrint, also with my_buffer as a parameter. Before continuing, test that this works as expected. Then add a call to bufferSetCel1, that says to add the letter "A' to the buffer in row 10, column 5. For example, bufferSetCell (my_buffer, "A', 10, 5):- Add another call to bufferPrint after this call to bufferSetcell. Before continuing, test that this works as expected. Then add four more calls to bufferSetcel1 to continue with three more A's and then a row of four B's undermeath to appear in rows 10 and 11 as: AAAA BB Then try specifying positions outside cach edge of the buffer and ensure that it works as expected. Part B: The Game [23 / 40 marks) In Part B, you will begin implementing the game. By the end of Part B, you will have functions with the following prototypes: int main () : void loadPlayer (); void loadAsteroid (); bool iscollision (int player_row, int player_column, int asteroid_row, int asteroid_column); Perform the following steps: 1. Define two constants to represent the size in rows and columns of the player ship "image". Create a two-dimensional array of chars to store the player ship "image". Define similar constants and create a similar array for the asteroid "image"- Note: Put these constants and declarations in the BigMain.cpp file outside and before all of the functions. They are global constants/variables. 2. Copy in the function prototypes shown above. 3. Implement a function, named loadPlayer, that takes no parameters. Lf N I Id.ntents of the LEluintuutualakul 11:11 I Search Back Assignment1.docx Perform the following steps: 1. Define two constants to represent the size in rows and columns of the player ship "image". Create a two-dimensional array of chars to store the player ship "image". Define similar constants and create a similar array for the asteroid "image". - Note: Put these constants and declarations in the BigMain.cpp file outside and before all of the functions. They are global constants/variables. 2. Copy in the function prototypes shown above. 3. Implement a function, named loadPlayer, that takes no parameters. This function should load the contents of the "player.txt" file into the global aray that stores the player ship "image". - Note: Immediately after you open the file, check to make sure that it opened correctly. If not, print an error message that includes the file name. For the rest of the CS 115 course, always perform this check whenever you attempt to open a file. - Hint: Use the getline function to read a line from the input file. If the name of the ifstream variable is fin: string line; getline (fin, line): Then copy the contents of the line into your array using a for loop. Do not use the stream extraction operator (>>) instead of getline because it will ignore the spaces, and we need those. - Debugging: Write a simple main function that calls the loadPlayer function. Print out the contents of the player ship "image" to ensure it was loaded correctly. You will need a double for loop. After you have confirmed that this works, comment out or delete this code. 4. Implement a function named loadAsteroid to load the asteroid "image". It should be similar to the loadPlayer function. Remember to use the asteroid constants instead of the player constants. - Debugging: Test the loadAsteroid function in a similar way to how you tested loadPlayer. s. Implement a ma in function with a local variable for the buffer. The main function should also have a pair of row and column variables to represent the position of the player ship. The player ship starts with its upper-left comer at row 10 and column 1 (rows and columns are numbered from 0). The main function should start by declaring the variables and calling the loadPlayer function. Then it should display the game state by doing the following: (1) clear the buffer, (2) use a double for loop to add the characters from the player ship image into the buffer at the position of the player ship, and (3) print the buffer. In the second of these steps, you should call setBuffercel1 (inside the double for loop) to add cach single character to the buffer. . Add another pair of row and column variables to main to represent the position of the asteroid. It starts at row 5 and column 30. Call the loadAsteroid function immediately after you call leadPlaver and cony the asteroid image into the buffer immediately after you cony the nlayer image into the 11:11 I Search Back Assignment1.docx image into the buffer at the position of the player ship, and (3) print the buffer. In the second of these steps, you should call setBufferCel1 (inside the double for loop) to add cach single character to the buffer. 4. Add another pair of row and column variables to main to represent the position of the asteroid. It starts at row 5 and column 30. Call the loadAsteroid function immediately after you call loadPlayer and copy the asteroid image into the buffer immediately after you copy the player image into the buffer. Only clear the buffer once and only display the buffer once. 1. Add a loop to the main function, called the main loop, that continues until the program terminates. The program should enter the main loop after all the variables have their initial values and both "images" have been loaded. The main loop should (1) display the game state, as described in step 5, (2) prompt the user for input by printing "Next? (without printing a endline), (3) read a line, and (4) process the first input character from the line (and do nothing with the rest of the line). If the first character is "q", the program should leave the main loop. Otherwise, if the first character is any other character, it should be ignored (do not print error messages). Hint: Use getline to read a line of user input. getline (cin, line): - Note: Make sure to handle the case where the input is an empty string. You can't look at the first character of an empty string! For the ne few pmay be helptal to tink of waching a bnd paciying townd a y wer (ateid. Imagine that you e wching the hid ying tgh bnla vievingwnd A the bird menes forward you koep moving the binoculas at the same eothat te bad stbe ationary in the binscular view. Aa the berd upproaches the wer the treppeatem iy , are. he atn , lm linate inere by me for eh me. Siilarly the viing winde has elin move thragh pace and again the olumn cordinate inc by neea he ige fer The ateds codinates are fisad. la the viewing window ompnding to the nowicalu pot from the right Intially, the viewing window ha its tap keit comer at condinate windew and the coendinstein spae e Fo i ide in viewing window up and doen we will only move ie the right. pa, the locatnthe m wewll et s. Add input commands to move the player ship. If the user enters the 'w', 's', or 'x' command, increment the column coordinate for the player ship. In addition, if the user enters a "w' command and the player ship is not in the uppermost row, the decrement the player row coordinate. Similarly, if the user enters an 'x' command and the player ship, taking into account its height, is not occupying the bottommost row, then increment the row coordinate. - Explanation: If the player ship tries to go out of bounds, the motion is disallowed, but no error message is printed. Thus, for example, if the player ship is on the top row and the user enters "w', you should still increase the column coordinate of the player. Similarly, you should still increase the column coordinate of the player if the player ship is at the bottom and the user enters 'x'. . Declare a counter variable in the main function to represent how far the viewing window has scrolled to the right in space. This variable should have an initial value of 0 and be incremented ser enters. Each 11:11 I Search Back Assignment1.docx LCCiare a counter vananie in tne main uncnon to represent how far the viewing window has scrolled to the right in space. This variable should have an initial value of 0 and be incremented for each 'w', 's', or 'x' the user enters. Each time you call bufferSetcell to put a character into the buffer, you should subtract this counter variable from the column coordinate of the item that you want to draw. For example, if one character in the asteroid has a column coordinate of 38 and the counter is 5, use 33 as the column parameter when you call the bufferSetcel1 function to print the character. Note: The purpose of this step is to implement the "side scrolling" aspect of the game. It will cause the viewing window to move one position in space for each wisx move that the player makes. Thus, the asteroid will appear to move one position to the left in the viewing window for each wisix move that the player makes. At the same time, the spaceship moves one to the right on cach wis/x move. Thus, the spaceship will stay in the leftmost column of the viewing window. It would look the same if you moved the asteroid and kept the spaceship still, but this way will work better when there are many asteroids. n. Implement a function, named isCollision, that takes four ints as parameters (representing the row and column coordinates for the player ship and the row and columa coordinates for the asteroid) and returns a bool indicating whether or not the two collide. This function can also use the constants representing the height and width of the player ship and the height and width of the asteroid. A collision occurs if the rectangular images for the player ship and the asteroid overlap. - Note: To write this function, you can write one long IF statement or a series of IF statements. For example, if the row coordinate of the player ship plus the height of the player ship is less than the row coordinate of the asteroid, there is no collision. n. Implement an additional check in the main function. Each time the player ship moves, the isCollision function should be used to test for a collision between the player ship and the asteroid. If there is a collision (ie, if the function returns true), the main function should print "Game Over" and terminate. Part C: Separate the Program into Modules [5/ 40 marks For Part C, you will separate your program into multiple files. 1. Save a copy of your existing file named BigMain.cpp somewhere safe. 2. Place the typedef for Buffer, the BUFFER_" constants, and the function prototypes from Part A in a header file named Buffer.h. 1. Put a line that #includes the header file Buffer.h in a new file named Buffer.cpp. Place the implementations of the buffer functions in Buffer.cppafter the #include line. 4. Copy your original file from BigMain.cpp to Main.cpp. Add a line that includes the Buffer.h header file at the top of Main n Bemoe the.constants function prototynes and function implementations that vou.coniod so 11:11 I Search Back Assignment1.docx Perform the following steps: 1. Define two constants to represent the size in rows and columns of the player ship "image". Create a two-dimensional array of chars to store the player ship "image". Define similar constants and create a similar array for the asteroid "image". - Note: Put these constants and declarations in the BigMain.cpp file outside and before all of the functions. They are global constants/variables. 2. Copy in the function prototypes shown above. 3. Implement a function, named loadPlayer, that takes no parameters. This function should load the contents of the "player.txt" file into the global aray that stores the player ship "image". - Note: Immediately after you open the file, check to make sure that it opened correctly. If not, print an error message that includes the file name. For the rest of the CS 115 course, always perform this check whenever you attempt to open a file. - Hint: Use the getline function to read a line from the input file. If the name of the ifstream variable is fin: string line; getline (fin, line): Then copy the contents of the line into your array using a for loop. Do not use the stream extraction operator (>>) instead of getline because it will ignore the spaces, and we need those. - Debugging: Write a simple main function that calls the loadPlayer function. Print out the contents of the player ship "image" to ensure it was loaded correctly. You will need a double for loop. After you have confirmed that this works, comment out or delete this code. 4. Implement a function named loadAsteroid to load the asteroid "image". It should be similar to the loadPlayer function. Remember to use the asteroid constants instead of the player constants. - Debugging: Test the loadAsteroid function in a similar way to how you tested loadPlayer. s. Implement a ma in function with a local variable for the buffer. The main function should also have a pair of row and column variables to represent the position of the player ship. The player ship starts with its upper-left comer at row 10 and column 1 (rows and columns are numbered from 0). The main function should start by declaring the variables and calling the loadPlayer function. Then it should display the game state by doing the following: (1) clear the buffer, (2) use a double for loop to add the characters from the player ship image into the buffer at the position of the player ship, and (3) print the buffer. In the second of these steps, you should call setBuffercel1 (inside the double for loop) to add cach single character to the buffer. . Add another pair of row and column variables to main to represent the position of the asteroid. It starts at row 5 and column 30. Call the loadAsteroid function immediately after you call leadPlaver and cony the asteroid image into the buffer immediately after you cony the nlayer image into the 11:11 I Search Back Assignment1.docx image into the buffer at the position of the player ship, and (3) print the buffer. In the second of these steps, you should call setBufferCel1 (inside the double for loop) to add cach single character to the buffer. 4. Add another pair of row and column variables to main to represent the position of the asteroid. It starts at row 5 and column 30. Call the loadAsteroid function immediately after you call loadPlayer and copy the asteroid image into the buffer immediately after you copy the player image into the buffer. Only clear the buffer once and only display the buffer once. 1. Add a loop to the main function, called the main loop, that continues until the program terminates. The program should enter the main loop after all the variables have their initial values and both "images" have been loaded. The main loop should (1) display the game state, as described in step 5, (2) prompt the user for input by printing "Next? (without printing a endline), (3) read a line, and (4) process the first input character from the line (and do nothing with the rest of the line). If the first character is "q", the program should leave the main loop. Otherwise, if the first character is any other character, it should be ignored (do not print error messages). Hint: Use getline to read a line of user input. getline (cin, line): - Note: Make sure to handle the case where the input is an empty string. You can't look at the first character of an empty string! For the ne few pmay be helptal to tink of waching a bnd paciying townd a y wer (ateid. Imagine that you e wching the hid ying tgh bnla vievingwnd A the bird menes forward you koep moving the binoculas at the same eothat te bad stbe ationary in the binscular view. Aa the berd upproaches the wer the treppeatem iy , are. he atn , lm linate inere by me for eh me. Siilarly the viing winde has elin move thragh pace and again the olumn cordinate inc by neea he ige fer The ateds codinates are fisad. la the viewing window ompnding to the nowicalu pot from the right Intially, the viewing window ha its tap keit comer at condinate windew and the coendinstein spae e Fo i ide in viewing window up and doen we will only move ie the right. pa, the locatnthe m wewll et s. Add input commands to move the player ship. If the user enters the 'w', 's', or 'x' command, increment the column coordinate for the player ship. In addition, if the user enters a "w' command and the player ship is not in the uppermost row, the decrement the player row coordinate. Similarly, if the user enters an 'x' command and the player ship, taking into account its height, is not occupying the bottommost row, then increment the row coordinate. - Explanation: If the player ship tries to go out of bounds, the motion is disallowed, but no error message is printed. Thus, for example, if the player ship is on the top row and the user enters "w', you should still increase the column coordinate of the player. Similarly, you should still increase the column coordinate of the player if the player ship is at the bottom and the user enters 'x'. . Declare a counter variable in the main function to represent how far the viewing window has scrolled to the right in space. This variable should have an initial value of 0 and be incremented ser enters. Each 11:11 I Search Back Assignment1.docx LCCiare a counter vananie in tne main uncnon to represent how far the viewing window has scrolled to the right in space. This variable should have an initial value of 0 and be incremented for each 'w', 's', or 'x' the user enters. Each time you call bufferSetcell to put a character into the buffer, you should subtract this counter variable from the column coordinate of the item that you want to draw. For example, if one character in the asteroid has a column coordinate of 38 and the counter is 5, use 33 as the column parameter when you call the bufferSetcel1 function to print the character. Note: The purpose of this step is to implement the "side scrolling" aspect of the game. It will cause the viewing window to move one position in space for each wisx move that the player makes. Thus, the asteroid will appear to move one position to the left in the viewing window for each wisix move that the player makes. At the same time, the spaceship moves one to the right on cach wis/x move. Thus, the spaceship will stay in the leftmost column of the viewing window. It would look the same if you moved the asteroid and kept the spaceship still, but this way will work better when there are many asteroids. n. Implement a function, named isCollision, that takes four ints as parameters (representing the row and column coordinates for the player ship and the row and columa coordinates for the asteroid) and returns a bool indicating whether or not the two collide. This function can also use the constants representing the height and width of the player ship and the height and width of the asteroid. A collision occurs if the rectangular images for the player ship and the asteroid overlap. - Note: To write this function, you can write one long IF statement or a series of IF statements. For example, if the row coordinate of the player ship plus the height of the player ship is less than the row coordinate of the asteroid, there is no collision. n. Implement an additional check in the main function. Each time the player ship moves, the isCollision function should be used to test for a collision between the player ship and the asteroid. If there is a collision (ie, if the function returns true), the main function should print "Game Over" and terminate. Part C: Separate the Program into Modules [5/ 40 marks For Part C, you will separate your program into multiple files. 1. Save a copy of your existing file named BigMain.cpp somewhere safe. 2. Place the typedef for Buffer, the BUFFER_" constants, and the function prototypes from Part A in a header file named Buffer.h. 1. Put a line that #includes the header file Buffer.h in a new file named Buffer.cpp. Place the implementations of the buffer functions in Buffer.cppafter the #include line. 4. Copy your original file from BigMain.cpp to Main.cpp. Add a line that includes the Buffer.h header file at the top of Main n Bemoe the.constants function prototynes and function implementations that vou.coniod so 11:11 I Search Back Assignment1.docx returns true), the main lunction should print "Game Over" and terminate. Part C: Separate the Program into Modules (5/ 40 marks For Part C, you will separate your program into multiple files. 1. Save a copy of your existing file named BigMain.cpp somewhere safe. 2. Place the typedef for Buffer, the BUFFER_" constants, and the function prototypes from Part A in a header file named Buffer.h. 1. Put a line that #includes the header file Buffer.h in a new file named Buffer.cpp. Place the implementations of the buffer functions in Buffer.cpp after the #include line. 4. Copy your original file from BigMain.cpp to Main.cpp. Add a line that #includes the Buffer.h header file at the top of Main.cpp. Remove the constants, function prototypes, and function implementations that you copied to other files from Main.cpp. After removing them, Main.cpp should contain the main, loadPlayer, loadAsteroid, and iscollision functions (and possibly helper functions of your own if you added any). s. Upload your source and header files and all your data files to hercules.cs.uregina.ca and compile them together as explained in the lab. Try compiling only BigMain.cpPp. Then try compiling Buffer.cppand Main.cpp together. Formatting |-5 marks if not done] 1. Neatly indent and format your program. Use a consistent indentation scheme and make sure to put spaces around your arithmetic operators, eg, x- x + 3:. Submission - Submit a complete copy of your source code (only your source code!). You should have the following files with exactly these names: 1. BigMain.cPp 2. Buffer.h 3. Buffer.cpPP 4. Main.cpp Note: A Visual Studio .sln file does NOT contain the source code, it is just a text file. You do not need to submit it. Make sure you submit the .cpp files and .h files. - If possible, combine all your files into a single.zip file before handing them in - Do NOT submit a compiled version or sample output - Do NOT submit intermediate files, such as: Debug folder Release folder ipch folder *.ncb,.sdf, or.db files *.o files (created by g++) 11:10 Search Back Assignment1.docx In Assignment 1, you will design and implement code to (1) display the player ship and the region of space in front of it, (2) move the player ship, and (3) detect collisions with a single asteroid. In the game, the user will enter text commands to control the mining ship and print a picture of the player ship and the space in front of it. If the player ship collides with the asteroid, the program prints a message and terminates. The user can also terminate the program with the 'q' command at any time. As well, after cach move, the region of space that is displayed in the game will move one column to the right. When running, the game will look as follows: 1--\ IX | 1--/ + --<_l_>/ * C======\ 11:10 I Search Back Assignment1.docx The # symbols should be printed to show the border of the arca being displayed. The shape to the left is the player ship and the shape near the middle is the asteroid. The purpose of this assignment is to ensure that you understand how to use two-dimensional arrays, including defining types using two- dimensional arrays and passing two-dimensional arrays to functions. For Part A, you will develop a module that defines a display buffer. For Part B, you will begin implementing the game. For Part C. you will separate your program into multiple files. The Buffer To prepare for displaying the player ship and the region of space in front of it, a temporary area of memory, known as a buffer, should be used to represent the picture before it is displayed. The buffer is represented by a two-dimensional array of characters. Before drawing each frame, the buffer is initialized to contain all blank (* ") characters. After that, the characters representing the player ship and the asteroid are copied into the buffer. Finally, the buffer is printed on the screen. In computer science terminology, any arca of memory used for temporary storage is known as a buffer, and the technique of using a buffer to hold data temporarily is known as buffering. This technique is commonly used in computer graphics, where colour values for pixels are stored instead of characters. The advantage of buffering in this case is that it is not necessary to work out, on a character-by-character basis, exactly what should be printed. Instead, each item to be shown can simply be placed in the appropriate position in the buffer, without worrying about the order in which the characters will be printed. Displaying Things The player ship and the asteroid will each be represented using a two-dimensional array of characters. The characters in each array will be loaded from a file once when the program is started, and then they are copied to the display buffer whenever the current state of the game is displayed. The "images" for the player ship and asteroid are stored in files named player.txt and asteroid.txt. 11:11 I Search Assignment1.docx Back asteroid are stored in files named player.txt and asteroid.txt. The contents of the player.txt file are as follows: c-<_l_>/ C------ The contents of the asteroid.txt file are as follows: /--\ IXXI \--/ Beginning in Assignment 2, you will replace the XX on the asteroid with a number representing its ore value. Part A: The Buffer Type (12/40 marks] In Part A, you will write the buffer module. You will eventually put these functions in their own module, but for now put all the code in a file named BigMain.cpp. Throughout this course, make sure that you use exactly the specified file names, which are case sensitive. Here, B and M are uppercase and the remaining leters are lowercase. By the end of Part A, you will have created functions for handling the buffer with the following prototypes: void bufferPrint (const Buffer buff); void bufferClear (Buffer buff): void bufferSetcell (Buffer buff, int row, int column, char value); Perform the following steps: 1. Define BUFFER_ROW_COUNT and BUFFER_COLUMN_COUNT as integer constants with values of 20 and 60, respectively. Define BUFFER_EMPTY and BUFFER_BORDER as character constants with values of. and '' respectively. Use typedef to define a Buffer type corresponding to a 2D array of chars with dimensions BUFFER ROW_COUNT and BUFFER_COLUMN_COUNT. - Put these constants and declarations in the BigMain.cpp file outside and before the functions defined in step A2 and subsequent steps. They are global constants and a global type definition. 2. Copy in the function prototypes for bufferPrint, bufferClear, and bufferSetcel1. 1. Implement a bufferClear function that takes a Buffer as a parameter. This function should set the value of each element in the Buffer to BUFFER_EMPTY. 4. Implement a bufferPrint function that takes a Buffer as a parameter. This function should print the contents of the Buffer with a border of BUFFER_BORDER characters around it. Hint: Start by printing a line of BUFFER BORDER characters THC, tor cac lne a 11:11 I Search Back Assignment1.docx - Hint: Start by printing a line of BUFFER_BORDER characters. Then, for each line, print a BUFFER BORDER character, then all the characters in one row of the Buffer, then another BUFFER_BORDER character, and then a newline. Finally, print another line of BUFFER_BORDER characters. 3. Implement a bufferSetcell function that takes a Buffer, two ints representing a row and column, and a char representing the new value, as parameters. This function should set the element of the Buffer specified by the row and column to the character value. If the row and column lie outside the Buffer, there should be no effect. Debugging: To test the Buffer functions, create a nain function. It should first declare a variable of type Buffer named ny_buffer. Then it should call the bufferclear function with my_buffer as a parameter and then call bufferPrint, also with my_buffer as a parameter. Before continuing, test that this works as expected. Then add a call to bufferSetCel1, that says to add the letter "A' to the buffer in row 10, column 5. For example, bufferSetCell (my_buffer, "A', 10, 5):- Add another call to bufferPrint after this call to bufferSetcell. Before continuing, test that this works as expected. Then add four more calls to bufferSetcel1 to continue with three more A's and then a row of four B's undermeath to appear in rows 10 and 11 as: AAAA BB Then try specifying positions outside cach edge of the buffer and ensure that it works as expected. Part B: The Game [23 / 40 marks) In Part B, you will begin implementing the game. By the end of Part B, you will have functions with the following prototypes: int main () : void loadPlayer (); void loadAsteroid (); bool iscollision (int player_row, int player_column, int asteroid_row, int asteroid_column); Perform the following steps: 1. Define two constants to represent the size in rows and columns of the player ship "image". Create a two-dimensional array of chars to store the player ship "image". Define similar constants and create a similar array for the asteroid "image"- Note: Put these constants and declarations in the BigMain.cpp file outside and before all of the functions. They are global constants/variables. 2. Copy in the function prototypes shown above. 3. Implement a function, named loadPlayer, that takes no parameters. Lf N I Id.ntents of the LEluintuutualakul 11:11 I Search Back Assignment1.docx Perform the following steps: 1. Define two constants to represent the size in rows and columns of the player ship "image". Create a two-dimensional array of chars to store the player ship "image". Define similar constants and create a similar array for the asteroid "image". - Note: Put these constants and declarations in the BigMain.cpp file outside and before all of the functions. They are global constants/variables. 2. Copy in the function prototypes shown above. 3. Implement a function, named loadPlayer, that takes no parameters. This function should load the contents of the "player.txt" file into the global aray that stores the player ship "image". - Note: Immediately after you open the file, check to make sure that it opened correctly. If not, print an error message that includes the file name. For the rest of the CS 115 course, always perform this check whenever you attempt to open a file. - Hint: Use the getline function to read a line from the input file. If the name of the ifstream variable is fin: string line; getline (fin, line): Then copy the contents of the line into your array using a for loop. Do not use the stream extraction operator (>>) instead of getline because it will ignore the spaces, and we need those. - Debugging: Write a simple main function that calls the loadPlayer function. Print out the contents of the player ship "image" to ensure it was loaded correctly. You will need a double for loop. After you have confirmed that this works, comment out or delete this code. 4. Implement a function named loadAsteroid to load the asteroid "image". It should be similar to the loadPlayer function. Remember to use the asteroid constants instead of the player constants. - Debugging: Test the loadAsteroid function in a similar way to how you tested loadPlayer. s. Implement a ma in function with a local variable for the buffer. The main function should also have a pair of row and column variables to represent the position of the player ship. The player ship starts with its upper-left comer at row 10 and column 1 (rows and columns are numbered from 0). The main function should start by declaring the variables and calling the loadPlayer function. Then it should display the game state by doing the following: (1) clear the buffer, (2) use a double for loop to add the characters from the player ship image into the buffer at the position of the player ship, and (3) print the buffer. In the second of these steps, you should call setBuffercel1 (inside the double for loop) to add cach single character to the buffer. . Add another pair of row and column variables to main to represent the position of the asteroid. It starts at row 5 and column 30. Call the loadAsteroid function immediately after you call leadPlaver and cony the asteroid image into the buffer immediately after you cony the nlayer image into the 11:11 I Search Back Assignment1.docx image into the buffer at the position of the player ship, and (3) print the buffer. In the second of these steps, you should call setBufferCel1 (inside the double for loop) to add cach single character to the buffer. 4. Add another pair of row and column variables to main to represent the position of the asteroid. It starts at row 5 and column 30. Call the loadAsteroid function immediately after you call loadPlayer and copy the asteroid image into the buffer immediately after you copy the player image into the buffer. Only clear the buffer once and only display the buffer once. 1. Add a loop to the main function, called the main loop, that continues until the program terminates. The program should enter the main loop after all the variables have their initial values and both "images" have been loaded. The main loop should (1) display the game state, as described in step 5, (2) prompt the user for input by printing "Next? (without printing a endline), (3) read a line, and (4) process the first input character from the line (and do nothing with the rest of the line). If the first character is "q", the program should leave the main loop. Otherwise, if the first character is any other character, it should be ignored (do not print error messages). Hint: Use getline to read a line of user input. getline (cin, line): - Note: Make sure to handle the case where the input is an empty string. You can't look at the first character of an empty string! For the ne few pmay be helptal to tink of waching a bnd paciying townd a y wer (ateid. Imagine that you e wching the hid ying tgh bnla vievingwnd A the bird menes forward you koep moving the binoculas at the same eothat te bad stbe ationary in the binscular view. Aa the berd upproaches the wer the treppeatem iy , are. he atn , lm linate inere by me for eh me. Siilarly the viing winde has elin move thragh pace and again the olumn cordinate inc by neea he ige fer The ateds codinates are fisad. la the viewing window ompnding to the nowicalu pot from the right Intially, the viewing window ha its tap keit comer at condinate windew and the coendinstein spae e Fo i ide in viewing window up and doen we will only move ie the right. pa, the locatnthe m wewll et s. Add input commands to move the player ship. If the user enters the 'w', 's', or 'x' command, increment the column coordinate for the player ship. In addition, if the user enters a "w' command and the player ship is not in the uppermost row, the decrement the player row coordinate. Similarly, if the user enters an 'x' command and the player ship, taking into account its height, is not occupying the bottommost row, then increment the row coordinate. - Explanation: If the player ship tries to go out of bounds, the motion is disallowed, but no error message is printed. Thus, for example, if the player ship is on the top row and the user enters "w', you should still increase the column coordinate of the player. Similarly, you should still increase the column coordinate of the player if the player ship is at the bottom and the user enters 'x'. . Declare a counter variable in the main function to represent how far the viewing window has scrolled to the right in space. This variable should have an initial value of 0 and be incremented ser enters. Each 11:11 I Search Back Assignment1.docx LCCiare a counter vananie in tne main uncnon to represent how far the viewing window has scrolled to the right in space. This variable should have an initial value of 0 and be incremented for each 'w', 's', or 'x' the user enters. Each time you call bufferSetcell to put a character into the buffer, you should subtract this counter variable from the column coordinate of the item that you want to draw. For example, if one character in the asteroid has a column coordinate of 38 and the counter is 5, use 33 as the column parameter when you call the bufferSetcel1 function to print the character. Note: The purpose of this step is to implement the "side scrolling" aspect of the game. It will cause the viewing window to move one position in space for each wisx move that the player makes. Thus, the asteroid will appear to move one position to the left in the viewing window for each wisix move that the player makes. At the same time, the spaceship moves one to the right on cach wis/x move. Thus, the spaceship will stay in the leftmost column of the viewing window. It would look the same if you moved the asteroid and kept the spaceship still, but this way will work better when there are many asteroids. n. Implement a function, named isCollision, that takes four ints as parameters (representing the row and column coordinates for the player ship and the row and columa coordinates for the asteroid) and returns a bool indicating whether or not the two collide. This function can also use the constants representing the height and width of the player ship and the height and width of the asteroid. A collision occurs if the rectangular images for the player ship and the asteroid overlap. - Note: To write this function, you can write one long IF statement or a series of IF statements. For example, if the row coordinate of the player ship plus the height of the player ship is less than the row coordinate of the asteroid, there is no collision. n. Implement an additional check in the main function. Each time the player ship moves, the isCollision function should be used to test for a collision between the player ship and the asteroid. If there is a collision (ie, if the function returns true), the main function should print "Game Over" and terminate. Part C: Separate the Program into Modules [5/ 40 marks For Part C, you will separate your program into multiple files. 1. Save a copy of your existing file named BigMain.cpp somewhere safe. 2. Place the typedef for Buffer, the BUFFER_" constants, and the function prototypes from Part A in a header file named Buffer.h. 1. Put a line that #includes the header file Buffer.h in a new file named Buffer.cpp. Place the implementations of the buffer functions in Buffer.cppafter the #include line. 4. Copy your original file from BigMain.cpp to Main.cpp. Add a line that includes the Buffer.h header file at the top of Main n Bemoe the.constants function prototynes and function implementations that vou.coniod so 11:11 I Search Back Assignment1.docx Perform the following steps: 1. Define two constants to represent the size in rows and columns of the player ship "image". Create a two-dimensional array of chars to store the player ship "image". Define similar constants and create a similar array for the asteroid "image". - Note: Put these constants and declarations in the BigMain.cpp file outside and before all of the functions. They are global constants/variables. 2. Copy in the function prototypes shown above. 3. Implement a function, named loadPlayer, that takes no parameters. This function should load the contents of the "player.txt" file into the global aray that stores the player ship "image". - Note: Immediately after you open the file, check to make sure that it opened correctly. If not, print an error message that includes the file name. For the rest of the CS 115 course, always perform this check whenever you attempt to open a file. - Hint: Use the getline function to read a line from the input file. If the name of the ifstream variable is fin: string line; getline (fin, line): Then copy the contents of the line into your array using a for loop. Do not use the stream extraction operator (>>) instead of getline because it will ignore the spaces, and we need those. - Debugging: Write a simple main function that calls the loadPlayer function. Print out the contents of the player ship "image" to ensure it was loaded correctly. You will need a double for loop. After you have confirmed that this works, comment out or delete this code. 4. Implement a function named loadAsteroid to load the asteroid "image". It should be similar to the loadPlayer function. Remember to use the asteroid constants instead of the player constants. - Debugging: Test the loadAsteroid function in a similar way to how you tested loadPlayer. s. Implement a ma in function with a local variable for the buffer. The main function should also have a pair of row and column variables to represent the position of the player ship. The player ship starts with its upper-left comer at row 10 and column 1 (rows and columns are numbered from 0). The main function should start by declaring the variables and calling the loadPlayer function. Then it should display the game state by doing the following: (1) clear the buffer, (2) use a double for loop to add the characters from the player ship image into the buffer at the position of the player ship, and (3) print the buffer. In the second of these steps, you should call setBuffercel1 (inside the double for loop) to add cach single character to the buffer. . Add another pair of row and column variables to main to represent the position of the asteroid. It starts at row 5 and column 30. Call the loadAsteroid function immediately after you call leadPlaver and cony the asteroid image into the buffer immediately after you cony the nlayer image into the 11:11 I Search Back Assignment1.docx image into the buffer at the position of the player ship, and (3) print the buffer. In the second of these steps, you should call setBufferCel1 (inside the double for loop) to add cach single character to the buffer. 4. Add another pair of row and column variables to main to represent the position of the asteroid. It starts at row 5 and column 30. Call the loadAsteroid function immediately after you call loadPlayer and copy the asteroid image into the buffer immediately after you copy the player image into the buffer. Only clear the buffer once and only display the buffer once. 1. Add a loop to the main function, called the main loop, that continues until the program terminates. The program should enter the main loop after all the variables have their initial values and both "images" have been loaded. The main loop should (1) display the game state, as described in step 5, (2) prompt the user for input by printing "Next? (without printing a endline), (3) read a line, and (4) process the first input character from the line (and do nothing with the rest of the line). If the first character is "q", the program should leave the main loop. Otherwise, if the first character is any other character, it should be ignored (do not print error messages). Hint: Use getline to read a line of user input. getline (cin, line): - Note: Make sure to handle the case where the input is an empty string. You can't look at the first character of an empty string! For the ne few pmay be helptal to tink of waching a bnd paciying townd a y wer (ateid. Imagine that you e wching the hid ying tgh bnla vievingwnd A the bird menes forward you koep moving the binoculas at the same eothat te bad stbe ationary in the binscular view. Aa the berd upproaches the wer the treppeatem iy , are. he atn , lm linate inere by me for eh me. Siilarly the viing winde has elin move thragh pace and again the olumn cordinate inc by neea he ige fer The ateds codinates are fisad. la the viewing window ompnding to the nowicalu pot from the right Intially, the viewing window ha its tap keit comer at condinate windew and the coendinstein spae e Fo i ide in viewing window up and doen we will only move ie the right. pa, the locatnthe m wewll et s. Add input commands to move the player ship. If the user enters the 'w', 's', or 'x' command, increment the column coordinate for the player ship. In addition, if the user enters a "w' command and the player ship is not in the uppermost row, the decrement the player row coordinate. Similarly, if the user enters an 'x' command and the player ship, taking into account its height, is not occupying the bottommost row, then increment the row coordinate. - Explanation: If the player ship tries to go out of bounds, the motion is disallowed, but no error message is printed. Thus, for example, if the player ship is on the top row and the user enters "w', you should still increase the column coordinate of the player. Similarly, you should still increase the column coordinate of the player if the player ship is at the bottom and the user enters 'x'. . Declare a counter variable in the main function to represent how far the viewing window has scrolled to the right in space. This variable should have an initial value of 0 and be incremented ser enters. Each 11:11 I Search Back Assignment1.docx LCCiare a counter vananie in tne main uncnon to represent how far the viewing window has scrolled to the right in space. This variable should have an initial value of 0 and be incremented for each 'w', 's', or 'x' the user enters. Each time you call bufferSetcell to put a character into the buffer, you should subtract this counter variable from the column coordinate of the item that you want to draw. For example, if one character in the asteroid has a column coordinate of 38 and the counter is 5, use 33 as the column parameter when you call the bufferSetcel1 function to print the character. Note: The purpose of this step is to implement the "side scrolling" aspect of the game. It will cause the viewing window to move one position in space for each wisx move that the player makes. Thus, the asteroid will appear to move one position to the left in the viewing window for each wisix move that the player makes. At the same time, the spaceship moves one to the right on cach wis/x move. Thus, the spaceship will stay in the leftmost column of the viewing window. It would look the same if you moved the asteroid and kept the spaceship still, but this way will work better when there are many asteroids. n. Implement a function, named isCollision, that takes four ints as parameters (representing the row and column coordinates for the player ship and the row and columa coordinates for the asteroid) and returns a bool indicating whether or not the two collide. This function can also use the constants representing the height and width of the player ship and the height and width of the asteroid. A collision occurs if the rectangular images for the player ship and the asteroid overlap. - Note: To write this function, you can write one long IF statement or a series of IF statements. For example, if the row coordinate of the player ship plus the height of the player ship is less than the row coordinate of the asteroid, there is no collision. n. Implement an additional check in the main function. Each time the player ship moves, the isCollision function should be used to test for a collision between the player ship and the asteroid. If there is a collision (ie, if the function returns true), the main function should print "Game Over" and terminate. Part C: Separate the Program into Modules [5/ 40 marks For Part C, you will separate your program into multiple files. 1. Save a copy of your existing file named BigMain.cpp somewhere safe. 2. Place the typedef for Buffer, the BUFFER_" constants, and the function prototypes from Part A in a header file named Buffer.h. 1. Put a line that #includes the header file Buffer.h in a new file named Buffer.cpp. Place the implementations of the buffer functions in Buffer.cppafter the #include line. 4. Copy your original file from BigMain.cpp to Main.cpp. Add a line that includes the Buffer.h header file at the top of Main n Bemoe the.constants function prototynes and function implementations that vou.coniod so 11:11 I Search Back Assignment1.docx returns true), the main lunction should print "Game Over" and terminate. Part C: Separate the Program into Modules (5/ 40 marks For Part C, you will separate your program into multiple files. 1. Save a copy of your existing file named BigMain.cpp somewhere safe. 2. Place the typedef for Buffer, the BUFFER_" constants, and the function prototypes from Part A in a header file named Buffer.h. 1. Put a line that #includes the header file Buffer.h in a new file named Buffer.cpp. Place the implementations of the buffer functions in Buffer.cpp after the #include line. 4. Copy your original file from BigMain.cpp to Main.cpp. Add a line that #includes the Buffer.h header file at the top of Main.cpp. Remove the constants, function prototypes, and function implementations that you copied to other files from Main.cpp. After removing them, Main.cpp should contain the main, loadPlayer, loadAsteroid, and iscollision functions (and possibly helper functions of your own if you added any). s. Upload your source and header files and all your data files to hercules.cs.uregina.ca and compile them together as explained in the lab. Try compiling only BigMain.cpPp. Then try compiling Buffer.cppand Main.cpp together. Formatting |-5 marks if not done] 1. Neatly indent and format your program. Use a consistent indentation scheme and make sure to put spaces around your arithmetic operators, eg, x- x + 3:. Submission - Submit a complete copy of your source code (only your source code!). You should have the following files with exactly these names: 1. BigMain.cPp 2. Buffer.h 3. Buffer.cpPP 4. Main.cpp Note: A Visual Studio .sln file does NOT contain the source code, it is just a text file. You do not need to submit it. Make sure you submit the .cpp files and .h files. - If possible, combine all your files into a single.zip file before handing them in - Do NOT submit a compiled version or sample output - Do NOT submit intermediate files, such as: Debug folder Release folder ipch folder *.ncb,.sdf, or.db files *.o files (created by g++)











Step by Step Solution
There are 3 Steps involved in it
Step: 1
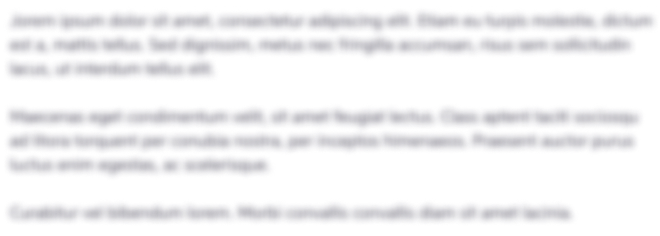
Get Instant Access with AI-Powered Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started