Question
1.18 Lab 1: Linked List Methods (in Java) The goal of this lab is to get familiar with implementing methods of a LinkedList. This is
1.18 Lab 1: Linked List Methods (in Java)
The goal of this lab is to get familiar with implementing methods of a LinkedList. This is an individual assignment; you cannot share code with other students.
This lab uses some methods that we implemented in the class and Lab0 such as append(int data), int length(), and int get(int index).
In this lab, you need to implement two more methods in the LinkedList class. The first method public void insertAfter(int givenData, int newData) inserts a Node with newData after the Node with givenData in the linked list.
The second method public void remove(int current) removes the Node that contains current from the LinkedList. You need to make sure your program handles the special case of deleting the head of the list.
The template contains the required code to test your implementation. So go ahead and find the comment //Your code here. You need to add codes for the specified methods only.
Sample Input/Output 11 23 31 -1 37 41 should output the following:
The list contains: [11, 23, 31] The length of the list is 3 Inserting a new node after the first node. Now the list contains:[11, 37, 23, 31] Inserting new data after the third node. Now the list contains:[11, 37, 23, 41, 31] The length of the list is 5 Removing the second element: Now the list contains:[11, 23, 41, 31] Removing the head Now the list contains:[23, 41, 31]
code:
public class LinkedListTemplate { Node head;
// inserts data to the end of the list only using the head pointer public void append(int data){ Node newNode = new Node(data); if(head == null){ head = newNode;
} else { Node currentNode = head; while(currentNode.next != null){ currentNode = currentNode.next; } currentNode.next = newNode; } } // inserts data to the beginning of the list public void prepend(int data){ if(head == null){ Node newNode = new Node(data); head = newNode; return; } Node newNode = new Node(data); newNode.next = head; head = newNode; } // print the linked list elements public void print() { Node currentNode = head; System.out.printf("["); while (currentNode.next != null) { System.out.printf("%d, ", currentNode.data); currentNode = currentNode.next; } System.out.printf("%d]%n", currentNode.data); } // counts the length of the list public int length(){ int length = 0; Node currentNode = head; while(currentNode != null){ length++; currentNode = currentNode.next; } return length; } // get an item from the list specified by the index public int get(int index){ int len = length(); if(index > len){ return -1; } Node currentNode = head; int currentIndex = 0; while(currentNode != null){ if(index == currentIndex){ return currentNode.data; } else{ currentNode = currentNode.next; currentIndex++; } } return -1; } // insert a new data after the given one public void insertAfter(int givenData, int newData){ // Your code here } // Removes the Node with the given data public void remove(int current) { // Your code here
} }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
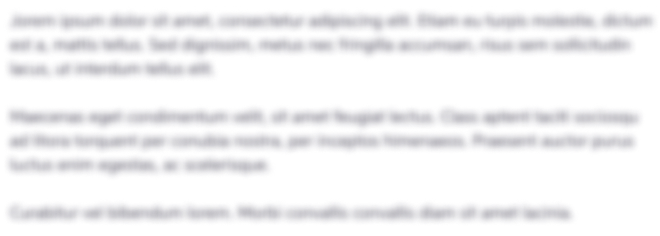
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started