Question
12. Design a new type called payrollT that is capable of holding the data for a list of employees, each of which is represented using
12. Design a new type called payrollT that is capable of holding the data for a list of employees, each of which is represented using the employeeT type introduced in the section on Dynamic records at the end of the chapter. The type payrollT should be a pointer type whose underlying value is a record containing the number of employees and a dynamic array of the actual employeeT values, as illustrated by the following data diagram:
After the input values have been entered, the GetPayroll function should return a value of type payrollT that matches the structure shown in the diagram.
13. Write a program that generates the weekly payroll for a company whose employment records are stored using the type payrollT, as defined in the preceding exercise. Each employee is paid the salary given in the employee record, after deducting taxes. Your program should compute taxes as follows:
Deduct $1 from the salary for each withholding exemption. This figure is the adjusted income. (If the result of the calculation is less than 0, use 0 as the adjusted income.)
Multiply the adjusted income by the tax rate, which you should assume is a flat 25 percent. For example, Bob Cratchit has a weekly income of $15. Because he has seven dependents, his adjusted income is $15 (7 x $1), or $8. Twenty-five percent of $8 is $2, so Mr. Cratchits net pay is $15 $2, or $13.
The payroll listing should consist of a series of lines, one per employee, each of which contains the employees name, gross pay, tax, and net pay. The output should be formatted in columns, as shown in the following sample run:
There is some confusion about Assignment 2 and I wanted to ensure that everyone is on the same page. Many of you were under the impression that writing GetPayroll was unnecessary because the function InitEmployeeTable already initialized an employee table of two employees. You still need to write GetPayroll for a user to enter an arbitrary number of employees to a payroll. InitEmployeeTable fills out an example table for demonstration purposes. The output figure at the beginning of page #2 of the assignment shows how this should work. Output is displayed in black and values in blue are entered via the keyboard. You should use the number entered on the first line asking "how many employees" to loop through the input fields of an employee. Remember that there is a limit based on the MaxEmployees macro (100 by default). Use these values to control the loop by which you obtain employee information. This function should return a pointer to a payroll upon termination.
booktypes.h
/* * Macro: New * Usage: p = New(pointer-type);
* The New pseudofunction allocates enough space to hold an * object of the type to which pointer-type points and returns * a pointer to the newly allocated pointer. Note that * "New" is different from the "new" operator used in C++; * the former takes a pointer type and the latter takes the * target type. */ #define New(type) ((type) malloc(sizeof *((type) NULL)))
/* * Macro: NewArray * Usage: p = NewArray(n, element-type); * NewArray allocates enough space to hold an array of n * values of the specified element type. */ #define NewArray(n, type) ((type *) malloc((n) * sizeof (type)))
-------------------------------------
employee.c
/* * File: employee.c * This program tests the functions defined for records of type * employeeRecordT and employeeT. */
#include
/* * Type: string * ------------ * The type string is identical to the type char *, which is * traditionally used in C programs. The main point of defining a * new type is to improve program readability. At the abstraction * levels at which the type string is used, it is usually not * important to take the string apart into its component characters. * Declaring it as a string emphasizes this atomicity. */
typedef char *string;
/* * Constants * --------- * MaxEmployees -- Maximum number of employees */
#define MaxEmployees 100
/* * Type: employeeRecordT * --------------------- * This structure defines a type for an employee record. */
typedef struct { string name; string title; string ssnum; double salary; int withholding; } employeeRecordT;
/* * Type: employeeT * --------------- * This type definition introduces an employeeT as a * pointer to the same record type as before. */
typedef struct { string name; string title; string ssnum; double salary; int withholding; } *employeeT;
/* * Type: payrollT * -------------- * This type represents an entire collection of employees. The * type definition uses a dynamic array of employeeT values to * ensure that there is no maximum bound imposed by the type. * The cost of this design is that the programmer must explicitly * allocate the storage for the array using NewArray. */
typedef struct { int nEmployees; employeeT *employees; } *payrollT;
/* * Global variables * ---------------- * staff -- Array of employees * nEmployees -- Number of employees * manager -- Used to produce a figure for the code */
static employeeRecordT staff[MaxEmployees]; static int nEmployees;
static employeeRecordT manager = { "Ebenezer Scrooge", "Partner", "271-82-8183", 250.00, 1 };
/* Private function declarations */
static void InitEmployeeTable(void); static payrollT CreatePayroll(employeeRecordT staff[], int nEmployees); static void ListEmployees(payrollT payroll); static double AverageSalary(payrollT payroll);
/* helper functions from the textbook lib */ string GetLine(void); string ReadLine(FILE *infile);
/* Main program */
main() { payrollT payroll;
InitEmployeeTable(); payroll = CreatePayroll(staff, nEmployees); ListEmployees(payroll); }
static void InitEmployeeTable(void) { employeeRecordT empRec;
empRec.name = "Ebenezer Scrooge"; empRec.title = "Partner"; empRec.ssnum = "271-82-8183"; empRec.salary = 250.00; empRec.withholding = 1; staff[0] = empRec; empRec.name = "Bob Cratchit"; empRec.title = "Clerk"; empRec.ssnum = "314-15-9265"; empRec.salary = 15.00; empRec.withholding = 7; staff[1] = empRec; nEmployees = 2; }
static payrollT CreatePayroll(employeeRecordT staff[], int nEmployees) { payrollT payroll; int i;
payroll = (payrollT) malloc(sizeof *payroll); // New(payrollT); payroll->employees = (employeeT *) malloc(nEmployees*sizeof(employeeT)); // NewArray(nEmployees, employeeT); payroll->nEmployees = nEmployees; for (i = 0; i employees[i] = (employeeT)malloc(sizeof *((employeeT) NULL)); // (New(employeeT); payroll->employees[i]->name = staff[i].name; payroll->employees[i]->title = staff[i].title; payroll->employees[i]->ssnum = staff[i].ssnum; payroll->employees[i]->salary = staff[i].salary; payroll->employees[i]->withholding = staff[i].withholding; } return (payroll); }
static void ListEmployees(payrollT payroll) { int i;
for (i = 0; i nEmployees; i++) { printf("%s (%s) ", payroll->employees[i]->name, payroll->employees[i]->title); } }
static double AverageSalary(payrollT payroll) { double total; int i;
total = 0; for (i = 0; i nEmployees; i++) { total += payroll->employees[i]->salary; } return (total / nEmployees); }
/* * to read strings from user and dynamically allocate memory, * it might be good idea to use the following helper functions * from the book lib * */ string ReadLine(FILE *infile) { string line, nline; int n, ch, size;
n = 0; size = 120; line = (string) malloc(size + 1); if(line==NULL) return NULL; while ((ch = getc(infile)) != ' ' && ch != EOF) { if (n == size) { size *= 2; nline = (string) malloc(size + 1); if(nline==NULL) {free(line); return NULL; } strncpy(nline, line, n); free(line); line = nline; } line[n++] = ch; } if (n == 0 && ch == EOF) { free(line); return (NULL); } line[n] = '\0'; nline = (string) malloc(n + 1); if(nline==NULL) {free(line); return NULL; } strcpy(nline, line); free(line); return (nline); }
string GetLine(void) { return (ReadLine(stdin));
2 nEmployees employees Ebenezer Scrooge Partner 27 1-82-8183 250.0O 1 Bob Cratchit Clerk 314-15-9 265 array employeeT 15. o 7 After writing the types that define this data structure, write a function GetPayroll that reads in a list of employees, as shown in the following sample run: How many employees: 2 Employee #1: Name: Ebenezer Scrooge Title: Partner SSNum: 271-82-8183 Salary: 250.00 Withholding exemptions: 1 Employee #2: Name: Bob Cratchit Title: Clerk SSNum: 314-15-9265 Salary: 15.00 Withholding exemptions: 7Step by Step Solution
There are 3 Steps involved in it
Step: 1
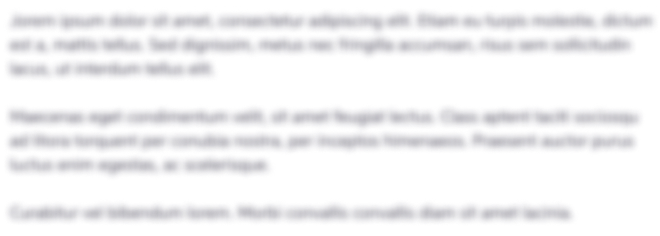
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started