Question
1.2 Step 2: Write Student class declaration with C++ A class in C++ is a user defined data type that has data and functions (also
1.2 Step 2: Write Student class declaration with C++
A class in C++ is a user defined data type that has data and functions (also called methods) as its members and whose access is governed by the three visibility modifiers - private, public, protected. We will seperate the class declaration with its definition here. Similar to a function's declaration and definition. For a class declaration, we will only specify each member functions' header without providing the body. All codes for Student class declaration need to be written between #define STUDENT_H_INCLUDED and #endif. The general structure of a class declaration should be similar to the following.
#include
class Student { private: //instance variables declaration public: //member function declaration };
We include the
class is a reserved word in C++, immediately follow it should be your class's name.
For encapsulation purpose, all instance variables should be declared with private visibility modifier and all member function should be declared with public visibility modifier. For this course, we will not use protected.
Class declaration must end with ; (the semi-colon outside the close brace)
Inside the Student class, declare some instance variables as above UML diagram shows: an integer variable called studentID, a string variable firstName, a string variable lastName, a string variable called major, an int called gradePoints and an int called totalCredits. Note: for encapsulation purpose, they should all be declared under private visibility modifier. See the following, //---- is where you need to write your own codes to declare those variables. // declare an int variable called studentID //----
// declare a string variable called firstName // declare a string variable called lastName //----
// declare a string variable called major //----
// declare an int variable called gradePoints //----
// declare an int variable called totalCredits //----
Next under the public visibility modifier, we should declare or provide the header of those member functions shown in above UML diagam. For example, for constructor, we should declare it as:
Student(int id, string fName, string lName, string major, int points, int credits);
or without providing any local varialbes inside the formal parameter list:
Student(int , string , string , string , int , int );
Note: for function declaration:
we only provide the header of the function, without function body;
function header must end with ;
According to above UML diagram, declare each of the following function one by one.
//function getId() is the accessor for instance variable studentID //---- //function getFullName() is the accessor for both firstName and lastName //you will need to use string concatenation to return the student full name //---- //function getMajor() is the accessor for instance variable major //---- //function getGradepoints() is the accessor for instance variable gradePoints //---- //function getCredits() is the accessor for instance variable totalCredits //---- //function changeMajor(string ) is the mutator for instance variable major //the function declaration is given as below
void changeMajor(string newMajor); //function changeMajor(string, int, int) is an overloadded mutator for major //the function declaration is given as below
void changeMajor(string newMajor, int newPoints, int newCredits);
//function toString() is used to print out all instance variables value
string toString();
When you finish writing each of above function's declaration, save the Student.h file.
1.3 Step 3: Create a new file Student.cpp and defining each of the function in Student.h inside
Inside CodeBlocks, from the left hand side control panel, click on CSE100Lab9 project. Next, similar as what we did in step 1.1, from the top menu, pick "File" => "New" => "File...", and pick "C/C++ Source". Next inside the text field "File name", enter Student (don't enter Student.cpp), you will notice that a file called Student.cpp is created under file folder "sources". You will need to write each of the functions you declared above inside this file.
Next, in order to link this file with the header file we created in step #2 - Student.h, we need to add the following directive inside
#include "Student.h"
You can include any other necessary libraries, such as
For each function's definition inisde the class Student.cpp, we need to put the class name and a double colon (::) immediately in front of the function name. The :: symbol is called the scope resolution operator. It is needed to indicate that these are class member functions and to tell the compiler which class they belong to. We will define the constructor first. Remember the constructor with arguments assigns input data to the instance variables.
Student::Student(int id, string fName, string lName, string major, int points, int credits) { studentID = id; // write the segment of codes that assign input parameters to each of the instance variables //---- }
1.4 Step 4: Finishing defining other functions inside the Student.cpp
As above UML diagram indicates, you need to supply the following accessors/getter functions:
getId() to get the ID of the Student object.
getFullName() to get the full name of the Student.
getMajor() to get the major of the Student object.
getGradepoints() to get the number of points of the Student object.
getCredits() to get the total credits of the Student object.
Hint: These functions contains a line of code that return the desired instance variable value(studentID, major, etc.)
int Student::getId() { // write a line of code that returns the studentID } string Student::getFullName() { // write a line of code that returns the full name of the student, includes both first and last name. //---- } string Student::getMajor() { // write a line of code that returns the student's major } int Student::getGradepoints() { // write a line of code that returns the student grade points } int Student::getCredits() { // write a line of code that returns the student total credits }
Also, according to above UML diagram, you should have two changeMajor() functions, they can be viewed as mutator for major variable. The function is overloaded. That is, the changeMajor() functionality is actually two functions, both with the same name that accept different number of input arguments. The first option will take only a string and will change the Students major to the new input as follows:
void Student::changeMajor(string newMajor) { // Change the value of the Students major variable to the new inputs value. //---- }
The second option, however, will be slightly more complex. In the second version of the changeMajor() function, we will accept a new major string, as before, and two integers, a new value for the students number of grade points and credits. Just change the major if your input variables i.e. points and credits are less than or equal to the current values before we assign the new values to their appropriate data members; otherwise print an "Invalid attempt" message on screen.
void Student::changeMajor(string newMajor, int newPoints, int newCredits) { // If newPoints and newCredits are less than or equal to their respective instance variable, update // the students major variable to its new major. Otherwise, print an error message 'Invalid attempt' //---- }
You also need to provide a toString() function. This function will return a string of the following format:
=================================== Student ID: 1234 Student Name: John Smith Major: Computer_Science Num. of Points: 345 Total credits: 100
Check the sample code Circle.cpp to see how we used sstream class to design the toString() function. Once you finish designing the Student.cpp, remember to save it.
1.5 Step 5: Calling the constructor of Student class from the main() method
In order to use/test on Student class variables and functions, we need to have a driver's program, the main() function will serve this purpose. The class main.cpp will have the main() function. Again, in order to use the Student class, you will need to add the following directive inside
#include "Student.h"
You can include any other necessary libraries, such as
variables and methods, you need to use the constructor to create an object first. int main() { //declare variables where you will store inputs from user //---- //prompt the user for inputs studentID, firstName, lastName, major //gradePoints and totalCredits. //---- //store the input in the declared variables //---- //use the constructor with arguments to create a brand-new Student object //called student1 using the variable values provided by the user //---- //more codes ......(continue on step #6) }
1.6 Step 6: Calling Student class fuctions and display the output
Put the following lines of code inside the main() function (i.e. continue on above step #5)
//call the getFullName() function to get the full name of the student. cout << " Student Name:\t" << //----student1.getFullName() + " "); //call the getId() method to get the ID of the student cout <<" Student ID:\t" << //----student1.getId() + " "); //call the toString() method to get every info. of the student //show it on screen cout << student1.toString() << endl; //Attempt to change the major to 'International Affairs' with 10 points and 500 credits //by calling changeMajor(String newMajor, int newPoints, int newCredits) function //This should not succeed. It should print the 'Invalid attempt" message. //---- //call getMajor() method and store the student's old major //into a variable oldMajor string oldMajor = //----;
//Change just the students major to //'International Affairs' by calling changeMajor(String newMajor) function //----
// Print out the following message on screen //
2. Sample Run of the Program
Below is an example of what your output should roughly look like when this lab is completed. Note: user input is bold
Enter first name: John Enter last name: Smith Enter student major: Computer_Science Enter student ID: 1234 Enter # of Points: 345 Enter # of credits: 100 Student Name: John Smith Student ID: 1234 ============================ Student ID: 1234 Student Name: John Smith Major: Computer_Science Num. of Points: 345 Total credits: 100 Invalid attempt John Smith has changed major from Computer_Science to International Affairs ============================ Student ID: 1234 Student Name: John Smith Major: International Affairs Num. of Points: 345 Total credits: 100
Step by Step Solution
There are 3 Steps involved in it
Step: 1
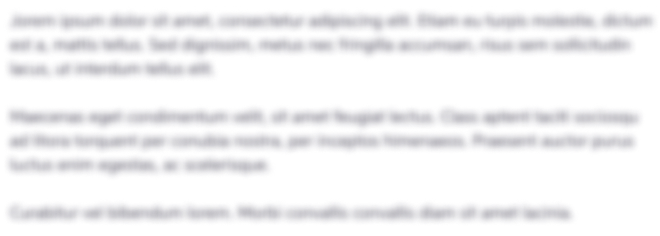
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started