Question
13.1 Bank Account (Individual Assignment) Required Skills Inventory Write an abstract base class in Java according to Interface specifications given in UML. Problem Description and
13.1 Bank Account (Individual Assignment)
Required Skills Inventory
Write an abstract base class in Java according to Interface specifications given in UML.
Problem Description and Given Info
You must write a public abstract class named BankAccount with fields and methods as defined below.
UML CLass Diagram: BankAccount
Structure of the Fields
As described by the UML Class Diagram above, your BankAccount class must have the following fields:
a protected field named accountID of type String, initialized to "0000-0000-0000-0000"
a protected field named interestRate of type double, initialized to 0.0
a protected field named balance of type int, initialized to 0
Structure of the Methods
As described by the UML Class Diagram above, your BankAccount class must have the following methods:
a public method named credit that takes an int argument and returns a boolean
a public abstract method named debit that takes an int argument and returns a boolean
a public method named getBalance that takes no arguments and returns an int
a public method named getAccountID that takes no arguments and returns an String
a public method named setAccountID that takes a String argument and returns nothing
a public method named getInterestRate that takes no arguments and returns a double
a public method named setInterestRate that takes a double argument and returns nothing
a public abstract method named applyInterest that takes no arguments and returns nothing
a public abstract method named accountInfo that takes no arguments and returns a String
Behavior of the Methods
The credit method should add the argument amount to the balance
The debit method is abstract so there will be no behavior or method body
The getBalance method should return the balance
The getAccountID method should return the accountID
The setAccountID method should store the argument value in the accountID field
The getBalance method should return the balance
The getInterestRate method should return the interestRate
The setInterestRate method should store the argument amount in the interestRate field
The applyInterest method is abstract so there will be no behavior or method body
The accountInfo method is abstract so there will be no behavior or method body
Additional Information
Since this is an abstract class, you will not be able to instantiate any object from it.
You are given a TestAccount class that inherits\extends the BankAccount class. you may use this to help with testing your BankAccount class.
You are also given a Main class with a main method where you can write code to test your BankAccount class.
All Bank Accounts
All accounts have balance, credit and debit amounts, and fees stored and passed as a number of pennies (int).
All debit amounts will be subtracted from the balance, and all credit amounts will be added to the balance.
All bank accounts have a non-negative interest rate (0.02 would be a 2% interest rate).
The credit method will always return true.
public abstract class BankAccount {
protected String accountID = "0000-0000-0000-0000";
protected double interestRate = 0;
protected int currentBalance = 0;
public boolean credit(int pennies) { currentBalance = currentBalance + pennies; return true; }
public abstract boolean debit(int pennies);
public int getBalance() { return currentBalance; }
public String getAccountID() { return accountID; }
public void setAccountID(String accountID) { this.accountID = accountID; }
public double getInterestRate() { return interestRate; }
public void setInterestRate(double interestRate) { this.interestRate = interestRate; }
public abstract void applyInterest();
public abstract String accountInfo(); }
public class TestAccount extends BankAccount {
public boolean debit(int pennies) { this.balance -= pennies; return true; } public void applyInterest() { this.balance += (this.interestRate * this.balance); } public String accountInfo() { return "TestAccount with Balance = " + String.format("$%.2f ", (this.balance / 100.0)); } }
TestAccount.java:8: error: cannot find symbol this.balance -= pennies; ^ symbol: variable balance TestAccount.java:13: error: cannot find symbol this.balance += (this.interestRate * this.balance); ^ symbol: variable balance TestAccount.java:13: error: cannot find symbol this.balance += (this.interestRate * this.balance); ^ symbol: variable balance TestAccount.java:17: error: cannot find symbol return "TestAccount with Balance = " + String.format("$%.2f ", (this.balance / 100.0)); ^ symbol: variable balance
By edit bankaccount.java to eliminate these errors pls!!!
Step by Step Solution
There are 3 Steps involved in it
Step: 1
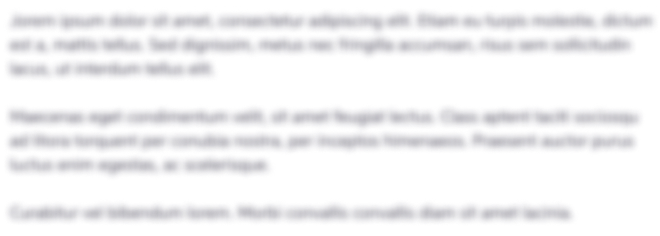
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started