Question
17.2 PA#2 Bicycle Race Results Problem description Write a C++ program that will allow a user to access the results from a time-trial bike race.
17.2 PA#2 Bicycle Race Results
Problem description
Write a C++ program that will allow a user to access the results from a time-trial bike race. The race consists of laps around a 6.1 mile course, and the racers try to complete as many laps as they can within 6 hours. Only complete laps count. Your program will read in the race results from a file and display the data in the format requested.
Your program will be provided with a dataset in a file containing the following information for each racer:
Bib number - a positive integer
Racers name - a string (may include spaces)
Distance covered by the racer (equal to the number of laps completed times 6.1)
Recorded time - a string in 05:23:32 format The number of laps is not provided and does not need to be computed.
Your main function should read from user input the filename in which the racer's results are stored. It will not contain more than 100 racer's results. A sample of this file named dataset.txt is available under Downloadable files below. When your main function starts, it should try to open the file using the filename it read from the input.
Then, it should offer the user a menu with the following options:
Display Results sorted by bib number
Display Results sorted by distance, then time
Lookup a bib number given a name
Lookup a result by bib number
Quit the Program
The program should perform the selected operation and then re-display the menu. Display an error message if the user enters an inappropriate number.
For options 1 and 2, display the results for each racer on a single, separate line. The results should include the Bib number, the name, the distance, and the time. The values should line up in columns (see "Tips" section below for more details).
For option 3, output the bib number and for option 4, output the results for the racer on a single line. For options 3 and 4, if the racer is not found display an appropriate message.
See sample_execution.txt in the Downloadable files section. In order to pass the test cases, your output will need to match exactly.
Additional Requirements:
This program should be completed in a Linux or Unix environment, using a command line compiler like g++.
You must submit a program for grading by 11:59pm Friday Feb 10 (worth 4pts). It must be a reasonable attempt (try passing just the first test case).
Your program should contain a structure called Result with the following 4 fields:
bibNumber - integer number
name - a string
distance - a double
time - a string
Important: Don't change field names/data types or their order. You'll not be able to pass all tests otherwise.
Use a partially filled array of structures to store the results. Use a counter variable to count the number of results that are read in from the file, and use this value as the size of the array for the search and sort functions.
Aside from main your program should have at least these 6 functions:
void readDataset(ifstream& in, Result results[], int &size); void displayDataset(Result results[], int size); int linearSearchByName(Result results[], int size, string targetName); int binarySearchByNumber(Result results[], int size, int targetNumber); void sortByNumber(Result results[], int size); void sortByDistanceTime(Result results[], int size);
readDataset. Should read results into results array from the "in" file stream (opened in main). Updates size.
displayDataset. Should nicely print results array (see "Tips" section)
linearSearchByName. Should find the index of a racer's result by their name using a linear search. If no such racer exist return -1.
binarySearchByNumber. Should find the index of a racer's result by their bib number using a binary search. If no such racer exist return -1.
sortByNumber. Should sort results by bibNumber field in ascending order. Must use bubble sort or selection sort algorithm.
sortByDistanceTime. Should sort results by distance (descending) and if two racers have the same distance they should be ordered by time in ascending order. Must use bubble sort or selection sort algorithm.
Important: Don't change function names, parameter/return data types or you'll not be able to pass all tests.
Do not use a library sort function or comparators to sort the data. Modify the code covered in class (included in Downloadable files below).
Tips
You can use the following function to print the menu:
void displayMenu(){ cout << " Menu "; cout << "1. Display Results sorted by bib number "; cout << "2. Display Results sorted by distance, then time "; cout << "3. Lookup a bib number given a name "; cout << "4. Lookup a result by bib number "; cout << "5. Quit the Program "; cout << "Enter your choice: "; }
You can use the following function to display a header for the results table. Use a modified version of the cout statement to output each line of the results.
void displayHeader(){ cout << left << setw(7) << "BibNum" << setw(18) << "Name" << right << setw(8) << "Distance" << setw(10) << "Time " << endl; }
In addition to normal tests, this lab uses unit tests - test that check correctness of one individual function at a time. You may see compilation errors when running these tests. It may mean that you haven't defined the required function yet (or your name/arguments/return value doesn't match).
Step by Step Solution
There are 3 Steps involved in it
Step: 1
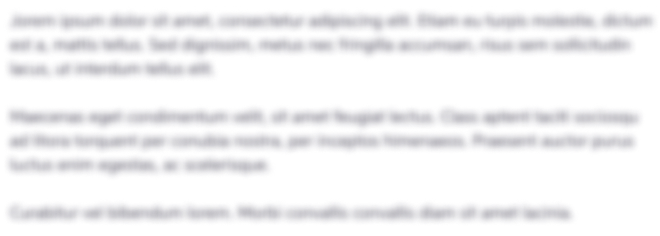
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started