Question
1-Assume that the Plant class looks like the following: public abstract class Plant { private int age=0; private int height=0; public int getAge() { return
1-Assume that the Plant class looks like the following:
public abstract class Plant {
private int age=0;
private int height=0;
public int getAge() {
return age;
}
public void addYearToAge() {
age++;
}
public int getHeight() {
return height;
}
public void setHeight(int height) {
this.height = height;
}
abstract public void doSpring();
abstract public void doSummer();
abstract public void doFall();
abstract public void doWinter();
}
2- Assume that the MapleTree class looks like the following:
public class MapleTree extends Plant {
private static final int AMOUNT_TO_
GROW_I_ONE_GROWING_SEASON = 2;
// A tree grows upwards a certain number of feet a year.A tree does not die down to ground level during the winter. //
private void grow() {
int currentHeight = getHeight();
setHeight(currentHeight + AMOUNT_TO_GROW_IN_ONE_GROWING_SEASON);
}
public void doSpring() {
grow();
addYearToAge();
System.out.println("Spring: The maple tree is starting to grow " + "leaves and new branches");
System.out.println("\t Current Age: " + getAge() + " " +"Current Height: " + getHeight());
}
public void doSummer() {
grow();
System.out.println("Summer: The maple tree is continuing to grow");
System.out.println("\t Current Age: " + getAge() + " " +"Current Height: " + getHeight());
}
public void doFall() {
System.out.println("Fall: The maple tree has stopped growing and is losing its leaves");
System.out.println("\t Current Age: " + getAge() + " " + "Current Height: " + getHeight());
}
public void doWinter() {
System.out.println("Winter: The maple tree is dormant");
System.out.println("\t Current Age: " + getAge() + " " +"Current Height: " + getHeight());
}
}
3- Assume that the class Tulip looks like:
public class Tulip extends Plant {
// A tulip grows each year to the same height. During the winter they die down to ground level.//
private void grow() {
int currentHeight = getHeight();
setHeight(currentHeight + AMOUNT_TO_GROW_IN_ONE_GROWING_SEASON);
}
private void dieDownForWinter(){
setHeight(0);
}
public void doSpring() {
grow();
addYearToAge();
System.out.println("Spring: The tulip is starting to grow " +"up from the ground");
System.out.println("\t Current Age: " + getAge() + " " +"Current Height: " + getHeight());
}
public void doSummer() {
System.out.println("Summer: The tulip has stopped growing " +"and is flowering");
System.out.println("\t Current Age: " + getAge() + " " + "Current Height: " + getHeight());
}
public void doFall() {
System.out.println("Fall: The tulip begins to wilt");
System.out.println("\t Current Age: " + getAge() + " " +"Current Height: " + getHeight());
}
public void doWinter() {
dieDownForWinter();
System.out.println("Winter: The tulip is dormant underground");
System.out.println("\t Current Age: " + getAge() + " " + "Current Height: " + getHeight());}
}
This exercise will use the previous classes and add new functionality to it.
- Add a new class called Rose that will represent a rose. Use the Plant base class and implement all of the required methods.(20 points)
- Create a class called Simulator that will do the following:
- Instantiate a maple tree object from the class MapleTree(10 points)
- Instantiate a rose object from the class Rose (10 points)
- Instantiate a tulip object from the class Tulip (10 points)
- We want to simulate the life of these trees for 3 years. Use a for loop that will run for 3 years.(50 points)
- We will let the maple tree, rose, and tulip do the spring.
- We will let the maple tree, rose, and tulip do the summer.
- We will let the maple tree, rose, and tulip do the fall.
- We will let the maple tree, rose, and tulip do the winter.
- Copy the plant simulator into the text editor or IDE of your choice.
- Compile and run the example to ensure that the code has been copied correctly.
- Make sure that the output will look like this:
-
Creating a maple tree and tulip and a rose...
Entering a loop to simulate 3 years
Spring: The maple tree is starting to grow leaves and new branches
Current Age: 1 Current Height: 2
Spring: The tulip is starting to grow up from the ground
Current Age: 1 Current Height: 1
Spring: The rose is starting to grow up from the ground
Current Age: 1 Current Height: 1
Summer: The maple tree is continuing to grow
Current Age: 1 Current Height: 4
Summer: The tulip has stopped growing and is flowering
Current Age: 1 Current Height: 1
Summer: The rose has stopped growing and is flowering
Current Age: 1 Current Height: 1
Fall: The maple tree has stopped growing and is losing its leaves
Current Age: 1 Current Height: 4
Fall: The tulip begins to wilt
Current Age: 1 Current Height: 1
Fall: The rose begins to wilt
Current Age: 1 Current Height: 1
Winter: The maple tree is dormant
Current Age: 1 Current Height: 4
Winter: The tulip is dormant underground
Current Age: 1 Current Height: 0
Winter: The rose is dormant underground
Current Age: 1 Current Height: 0
Spring: The maple tree is starting to grow leaves and new branches
Current Age: 2 Current Height: 6
Spring: The tulip is start
ing to grow up from the ground
Current Age: 2 Current Height: 1
Spring: The rose is starting to grow up from the ground
Current Age: 2 Current Height: 1
Summer: The maple tree is continuing to grow
Current Age: 2 Current Height: 8
Summer: The tulip has stopped growing and is flowering
Current Age: 2 Current Height: 1
Summer: The rose has stopped growing and is flowering
Current Age: 2 Current Height: 1
Fall: The maple tree has stopped growing and is losing its leaves
Current Age: 2 Current Height: 8
Fall: The tulip begins to wilt
Current Age: 2 Current Height: 1
Fall: The rose begins to wilt
Current Age: 2 Current Height: 1
Winter: The maple tree is dormant
Current Age: 2 Current Height: 8
Winter: The tulip is dormant underground
Current Age: 2 Current Height: 0
Winter: The rose is dormant underground
Current Age: 2 Current Height: 0
Spring: The maple tree is starting to grow leaves and new branches
Current Age: 3 Current Height: 10
Spring: The tulip is starting to grow up from the ground
Current Age: 3 Current Height: 1
Spring: The rose is starting to grow up from the ground
Current Age: 3 Current Height: 1
Summer: The maple tree is continuing to grow
Current Age: 3 Current Height: 12
Summer: The tulip has stopped growing and is flowering
Current Age: 3 Current Height: 1
Summer: The rose has stopped growing and is flowering
Current Age: 3 Current Height: 1
Fall: The maple tree has stopped growing and is losing its leaves
Current Age: 3 Current Height: 12 Fall: The tulip begins to wilt Current Age: 3 Current Height: 1 Fall: The rose begins to wilt Current Age: 3 Current Height: 1 Winter: The maple tree is dormant Current Age: 3 Current Height: 12 Winter: The tulip is dormant underground Current Age: 3 Current Height: 0 Winter: The rose is dormant underground Current Age: 3 Current Height: 0
Step by Step Solution
There are 3 Steps involved in it
Step: 1
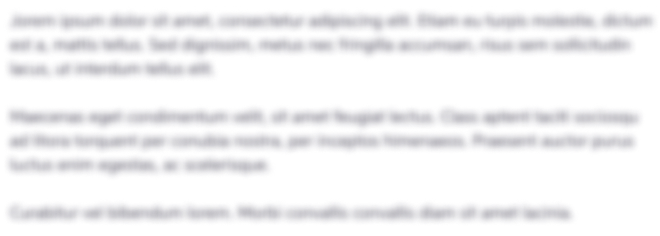
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started