Question
1.Cant seem to make code works, when i put in the infix expression like below it goves me an error 2.I cant seem to make
1.Cant seem to make code works, when i put in the infix expression like below it goves me an error
2.I cant seem to make the gui look like the one below
3. Need help on making UML Class diagram
The first programming project involves writing a program that evaluates infix expressions of unsigned integers using two stacks. THE PROGRAM SHOULD CONSIST OF THREE CLASSES The main class should create a GUI that allows the user input an infix expression and displays the result. The GUI should look as follows:
The GUI must be generated by code that you write. You may not use a drag-and-drop GUI generator.
THE SECOND CLASS should contain the code to perform the infix expression evaluation. The pseudocode for performing that evaluation is shown below:
tokenize the string containing the expression
while there are more tokens get the next token
if it is an operand
push it onto the operand stack
else if it is a left parenthesis
push it onto the operator stack
else if it is a right parenthesis
while top of the operator stack not a left parenthesis
pop two operands and an operator
perform the calculation
push the result onto the operand stack
else if it is an operator
while the operator stack is not empty and
the operator at the top of the stack has higher
or the same precedence than the current operator
pop two operands and perform the calculation
push the result onto the operand stack
push the current operator on the operators stack
while the operator stack is not empty
pop two operands and an operator
perform the calculation
push the result onto the operand stack
the final result is a the top of the operand stack
Be sure to add any additional methods needed to eliminate any duplication of code. Your program is only expected to perform correctly on syntactically correct infix expressions that contain integer operands and the four arithmetic operators + - * /. It should not, however, require spaces between tokens. The usual precedence rules apply. The division performed should be integer division. A check should be made for division by zero. Should the expression contain division by zero, a checked exception DivideByZero should be thrown by the method that performs the evaluation and caught in the main class, where a JOptionPane window should be displayed containing an error message.
ExpressionEvaluatorGUI.java:
import java.awt.Container; import java.awt.GridLayout; import java.awt.event.ActionEvent; import java.awt.event.ActionListener;
import javax.swing.JButton; import javax.swing.JFrame; import javax.swing.JLabel; import javax.swing.JTextField;
public class ExpressionEvaluatorGUI extends JFrame {
private ExpressionEvaluator expressionEvaluator;
private JLabel expressionLabel; private JLabel expressionValueLabel;
private JTextField expressionField; private JTextField expressionValueField;
// -----------------------------------------------------------------
// Declare Buttons private JButton calculateButton; private JButton quitButton;
public ExpressionEvaluatorGUI(ExpressionEvaluator expressionEvaluator) {
// Set expression evaluator this.expressionEvaluator = expressionEvaluator;
instantiateGUIComponents(); buildGUI(); addListeners();
// Set default jframe close operation setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
// SetWINDOW size pack(); setSize(450, 325);
// Set window visible setVisible(true);
}
// --------------------------------------------------------------
/** * Instantiates the GUI components */ private void instantiateGUIComponents() {
// Initialize GUI Copmponents
// Initialize Labels expressionLabel = new JLabel("Enter Infix Expression"); expressionValueLabel = new JLabel("Value of Expression");
// Initialize Fields expressionField = new JTextField(10); expressionValueField = new JTextField(10);
// Initialize Buttons calculateButton = new JButton("Calculate"); quitButton = new JButton("Exit");
// Set display fields to be not editable expressionValueField.setEditable(false); }
// --------------------------------------------------------------
/** * Builds the GUI by adding the components to the frame. */ private void buildGUI() {
// Get Pane Container contentPane = getContentPane();
// Set layout contentPane.setLayout(new GridLayout(3, 2, 10, 10));
// Add components contentPane.add(expressionLabel); contentPane.add(expressionField);
contentPane.add(expressionValueLabel); contentPane.add(expressionValueField);
contentPane.add(calculateButton); contentPane.add(quitButton);
}
// --------------------------------------------------------------
/** * Adds listeners to the GUI buttons */ private void addListeners() {
// Add listener to search button calculateButton.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) {
// Set Error message String expressionValueStr = "Error, try again";
// Get expression Expression infixExpression = new Expression(expressionField .getText());
// Evaluate Expression int expressionValue = expressionEvaluator .evaluate(infixExpression);
// Check if something went wrong if (expressionValue != Integer.MIN_VALUE) { expressionValueStr = Integer.toString(expressionValue); }
// Set expression value field expressionValueField.setText(expressionValueStr);
}
});
// Add listener to quit button quitButton.addActionListener(new ActionListener() { public void actionPerformed(ActionEvent e) { System.exit(0); } }); }
public static void main(String[] args) { // Instantiate an ExpressionEvaluator ExpressionEvaluator expressionEvaluator = new ExpressionEvaluator();
// Create instance of expression evaluator gui new ExpressionEvaluatorGUI(expressionEvaluator); }
}
ArrayListStack.java:
import java.util.ArrayList;
public class ArrayListStack
public ArrayListStack() { stackBody = new ArrayList
public boolean isEmpty() { return (stackBody.size() == 0); }
public void push(T item) { stackBody.add(item); }
public T pop() { if (isEmpty()) { System.out.println("Error in ArrayStack.pop() Stack Empty "); return null; } else { T topElement = stackBody.get(stackBody.size() - 1); stackBody.remove(stackBody.size() - 1); return topElement; } }
/** * *Here we need to mention the return type as 'T' * */ public T TTOP () { if (isEmpty()) { System.out.println("Error in ArrayStackTOP ()Stack Empty "); return null; } else { T topElement = stackBody.get(stackBody.size() - 1); return topElement; } }
}
Expression.java:
import java.util.ArrayList; import java.util.StringTokenizer;
public class Expression { private ArrayList
StringTokenizer strTok = new StringTokenizer(exp, " ");
// Scan the input while (strTok.hasMoreElements()) { Token tok = new Token((String) strTok.nextElement()); expression.add(tok); } } public Expression() { // Create the array that represents the body of the Expression expression = new ArrayList
public int size() { return expression.size(); }
public void add(Token newElement) { expression.add(newElement); } public String toString() { String ret = ""; for (int i = 0; i
public ArrayList
ExpressionEvaluator.java:
public class ExpressionEvaluator {
public int evaluate(Expression infix) { // InfixToPostfixConverter InfixToPostfixConverter itop = new InfixToPostfixConverter(infix);
// Convert infix to postfix itop.convertToPostfix(); Expression postfix = itop.getPostfix();
// Print the postfix System.out.println("Postfix Expression: " + postfix.toString());
// Instantiate a PostfixEvaluator PostfixEvaluator peval = new PostfixEvaluator(postfix);
// Evaluate the postfix expression int value = peval.eval();
// calculated value return value;
} }
InfixToPostfixConverter.java:
import java.util.ArrayList;
public class InfixToPostfixConverter { private Expression infixExpression;
private Expression postfixExpression;
public InfixToPostfixConverter(Expression infix) { infixExpression = infix; }
public void convertToPostfix() { // Create an empty postfix expression postfixExpression = new Expression();
// create an empty operator stack ArrayListStack
// Temporary local variables Token nextToken = null; Token topOfStack = null;
// Main loop ArrayList // If it is an operand append it to postfix if (nextToken.isOperand()) postfixExpression.add(nextToken); // If it is an open parenthesis push it into stack else if (nextToken.isOpenParen()) operatorStack.push(nextToken); // If next token is a closed parenthesis else if (nextToken.isClosedParen()) { // Keep pulling operators out of stack and appending // them to postfix, untilTOP of stack is an open paren topOfStack = operatorStack.TTOP(); while (!topOfStack.isOpenParen()) { postfixExpression.add(topOfStack); operatorStack.pop(); topOfStack = operatorStack.TTOP(); } // and then discard the open paren operatorStack.pop(); } // If it is an operator ... else if (nextToken.isOperator()) { // get the precedence of this token int tokenPrecedence = nextToken.getPrecedence(); // If stack is empty, push nextToken into stack if (operatorStack.isEmpty()) operatorStack.push(nextToken); else { // Get the precedence of theTOP of the stack /** * * Here we have to call 'TTOP' method using operatorStack object * */ topOfStack = operatorStack.TTOP(); // If the top of stack is an open parenthesis push nextToken if (topOfStack.isOpenParen()) operatorStack.push(nextToken); else { // Get the precedence of the top of stack int stackPrecedence = topOfStack.getPrecedence(); // if nextToken's precedence is > that ofTOP of stack's // push next token into stack if (tokenPrecedence > stackPrecedence) operatorStack.push(nextToken); else { // Keep pulling operators out of stack and appending // them to postfix, as long all all these conditions // are true while ((tokenPrecedence // At the end push nextToken into Stack operatorStack.push(nextToken); } } } } else { System.out.println("Illegal String in InfixToPostfixConverter"); break; } } // At the end of the infix expression: pull all the operators // out of the stack and append them to postfix while (!operatorStack.isEmpty()) { topOfStack = operatorStack.pop(); postfixExpression.add(topOfStack); } } //----------------------------------------------------------------- /** * Returns the current postfix expression * @return postfix expression */ public Expression getPostfix() { return postfixExpression; } } IStack.java: public interface IStack PostfixEvaluator.java: import java.util.ArrayList; public class PostfixEvaluator { // ----------------------------------------------------------------- // Input postfix expression private Expression postfixExpression; // ----------------------------------------------------------------- // Value of expression private int valueOfExpression; // ----------------------------------------------------------------- /** * Constructs evaluator from postfix expression */ public PostfixEvaluator(Expression postfix) { postfixExpression = postfix; } // ----------------------------------------------------------------- /** * Evaluates the postfixExpression * * @return value */ public int eval() { // Starts with an empty operand stack ArrayListStack // Temp variable Token nextToken; ArrayList // If it is an operand, push it into stack if (nextToken.isOperand()) { operandStack.push(nextToken); // System.out.println(operandStack); } // If it is an operator, else if (nextToken.isOperator()) { // Get two operands out of the stack if (operandStack.isEmpty()) { System.out.println("Error in PostfixEvaluator.eval() " + "-- Input expression was probably wrong"); return Integer.MIN_VALUE; } Token operand2 = operandStack.pop(); if (operandStack.isEmpty()) { System.out.println("Error in PostfixEvaluator.eval() " + "-- Input expression was probably wrong"); return Integer.MIN_VALUE; } Token operand1 = operandStack.pop(); // Perform the operation Token result = calculate(nextToken, operand1, operand2); // Push the result back into the stack operandStack.push(result); // System.out.println(operandStack); } } // At the end, if only one element is left in the stack if (operandStack.isEmpty()) { System.out.println("Error in PostfixEvaluator.eval() " + "-- Input expression was probably wrong"); return Integer.MIN_VALUE; } // Get the operand out of the stack, and convert it into // an integer Token topToken = operandStack.pop(); valueOfExpression = Integer.parseInt(topToken.getBody()); if (operandStack.isEmpty()) return valueOfExpression; else { System.out.println("Error in PostfixEvaluator.eval() " + "-- Input expression was probably wrong"); return Integer.MIN_VALUE; } } // ----------------------------------------------------------------- /** * Performs an arithmetic operation * * @param operator * @param operand1 * @param operand2 * @return */ private Token calculate(Token operatorToken, Token operand1Token, Token operand2Token) { // Get the operator from the token String operator = operatorToken.getBody(); // Get the two operands by converting from String to int int operand1 = Integer.parseInt(operand1Token.getBody()); int operand2 = Integer.parseInt(operand2Token.getBody()); // Default return value, in case an error occurs int result = Integer.MAX_VALUE; // Perform the operation, and set a value for result if (operator.equals("")) { if (operand1 > operand2) result = 1; else result = 0; } else if (operator.equals(">=")) { if (operand1 >= operand2) result = 1; else result = 0; } else if (operator.equals("==")) { if (operand1 == operand2) result = 1; else result = 0; } else if (operator.equals("!=")) { if (operand1 != operand2) result = 1; else result = 0; } else if (operator.equals("||")) { if (operand1 != 0 || operand2 != 0) result = 1; else result = 0; } else if (operator.equals("&&")) { if (operand1 != 0 && operand2 != 0) result = 1; else result = 0; } else if (operator.equals("+")) { result = operand1 + operand2; } else if (operator.equals("-")) { result = operand1 - operand2; } else if (operator.equals("*")) { result = operand1 * operand2; } else if (operator.equals("/")) { if (operand2 != 0) result = operand1 / operand2; else System.out.println("Division by zero error in" + " PostfixEvaluator.calculate()."); } else if (operator.equals("%")) { if (operand2 != 0) result = operand1 % operand2; else System.out.println("Division by zero error in" + " PostfixEvaluator.calculate()."); } else { System.out.println("Illegal Operator in " + "PostfixEvaluator.calculate()"); } // Convert res into a Token and return it. return new Token("" + result); } } Token.java: public class Token { // ------------------------------------------------------------- // It classifies the following as "OPERATORS". private final static String[] validOperators = { "", ">=", "==", "!=", "||", "&&", "+", "-", "*", "/", "%" }; // ------------------------------------------------------------- private final static char OPENPAREN = '('; private final static char CLOSEDPAREN = ')'; // ------------------------------------------------------------- // Holds contents of token private String body; public Token(String body) { this.body = body; } public String getBody() { return body; } public boolean isOperator() { for (int i = 0; i public boolean isOpenParen() { char ch = body.charAt(0); return (ch == OPENPAREN); } public boolean isClosedParen() { char ch = body.charAt(0); return (ch == CLOSEDPAREN); } public boolean isOperand() { return (!((isOperator() || isOpenParen() || isClosedParen()))); } public int getPrecedence() { if (body.equals("") || body.equals(">=")) return 1; else if (body.equals("==") || body.equals("!=")) return 2; else if (body.equals("||")) return 3; else if (body.equals("&&")) return 4; else if (body.equals("+") || body.equals("-")) return 5; else if (body.equals("*") || body.equals("/") || body.equals("%")) return 6; return -1; } public String toString() { return body; } } ExpressionEvaluatorTester.java: public class ExpressionEvaluatorTester { public static void main(String[] args) { // Create an array of Test Data Expression[] testExpressions = { new Expression("1 2"), new Expression("1 >= 2"), new Expression("1 3"), new Expression("1 3"), new Expression("1 > 2 || 2 > 3"), new Expression("1 3"), new Expression("1 5 ) * 3 ) % 6"), new Expression("( 25 2 )"), new Expression("25 + 45 "), new Expression("10 * ( 5 + 3 )"), new Expression("20"), new Expression("10 * 5 + 3"), new Expression("10 * ( 7 + ( 12 - 9 ) ) / 10"), new Expression("100 % ( ( 3 + 2 ) + 3 ) / 4"), new Expression("102 % ( ( 3 + 2 * 10 ) - 10 - 5 ) / 3"), new Expression("( 25 + ( 10 > 5 ) * 3 ) % 6") }; // Expected values of test data int[] expectedExpressionValues = { 1, 0, 1, 0, 0, 1, 0, 1, 1, 1, 1, 0, 1, 0, 1, 0, 0, 1, 0, 1, 6, 1, 9, 5, 4, 2, 70, 80, 20, 53, 10, 1, 2, 4 }; // Instantiate an ExpressionEvaluator ExpressionEvaluator expressionEvaluator = new ExpressionEvaluator(); // All Tests Passed Boolean boolean allTestsPassed = true; for (int i = 0; i }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
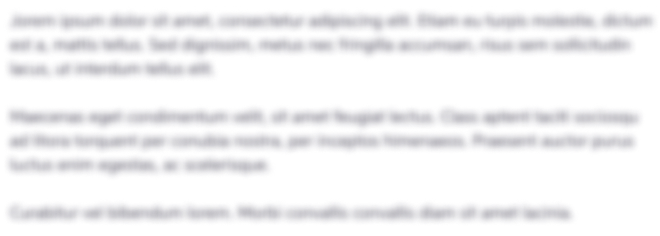
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started