Question
2- Write a Queue class. This class should use your LinkedList class. Your queue should hold integers. It must contain the following methods: Queue() Default
2- Write a Queue class. This class should use your LinkedList class.
Your queue should hold integers. It must contain the following methods:
Queue() Default constructor
enqueue(valueToAdd) Adds a new element to back of the queue
dequeue() Removes the element from the front of the queue and returns its value.
getLength() Returns the length of the
queue. display() Displays the queue and its contents.
It should display the first element in the queue first. See output below for an example.
I already have the linked list that is this one:
This linked list implementation should be in its own class named LinkedList. Your linked list should hold integers. It must contain the following methods:
Default constructor Creates a new node and adds it to the front of the linked list.
Removes the front node from the linked list and returns the value it contained. Creates a new node and adds it to the back of the linked list.
Removes the last node from the linked list and returns the value it contained. Returns the length of the linked list
Displays the linked list and its contents.
############ LinkedList.java ############
class NodeList {
int data;
NodeList next;
public NodeList(int data) {
this.data = data;
next = null;
}
}
public class LinkedList {
private NodeList head;
private int size;
public LinkedList() {
head = null;
size = 0;
}
public void addFront(int data) {
NodeList newNode = new NodeList(data);
newNode.next = head;
head = newNode;
size = size+1;
}
public int removeFront(){
if(head == null){
System.out.println("List is empty");
return -999;
}
int value = head.data;
head = head.next;
size = size-1;
return value;
}
public void addBack(int value){
if(head == null){
head = new NodeList(value);
}else{
NodeList temp = head;
while(temp.next != null)
temp = temp.next;
temp.next = new NodeList(value);
}
size = size+1;
}
public int removeBack(){
// list is empty
if(head == null){
System.out.println("List is empty");
return -999;
}
// only one node
if(head.next == null){
int value = head.data;
head = null;
return value;
}
NodeList temp = head;
while(temp.next.next != null)
temp = temp.next;
int value = temp.next.data;
temp.next = null;
size = size-1;
return value;
}
public int getLength(){
return size;
}
public void display(){
NodeList temp = head;
while(temp != null){
if(temp.next!=null)
System.out.print(temp.data+"-->");
else
System.out.println(temp.data);
temp = temp.next;
}
System.out.println();
}
}
############# LinkedListTest.java ################
public class LinkedListTest {
public static void main(String[] args) {
LinkedList list = new LinkedList();
System.out.println("Adding elements to the front......");
list.addFront(8);
list.display();
list.addFront(33);
list.display();
list.addFront(58);
list.display();
System.out.println("Length of linked list: "+list.getLength());
System.out.println();
System.out.println("Adding elements to the back......");
list.addBack(1);
list.display();
list.addBack(34);
list.display();
list.addBack(20);
list.display();
System.out.println("Length of linked list: "+list.getLength());
System.out.println();
System.out.println("Removing elements from the front.....");
list.removeFront();
list.display();
list.removeFront();
list.display();
System.out.println("Length of linked list: "+list.getLength());
System.out.println();
System.out.println("Removing elements from the back.....");
list.removeBack();
list.display();
list.removeBack();
list.display();
System.out.println("Length of linked list: "+list.getLength());
System.out.println();
}
}
/*
Sample run:
Adding elements to the front......
8
33-->8
58-->33-->8
Length of linked list: 3
Adding elements to the back......
58-->33-->8-->1
58-->33-->8-->1-->34
58-->33-->8-->1-->34-->20
Length of linked list: 6
Removing elements from the front.....
33-->8-->1-->34-->20
8-->1-->34-->20
Length of linked list: 4
Removing elements from the back.....
8-->1-->34
8-->1
Length of linked list: 2
*/
Linked List Adding elements to the front. 33 8 58 >33 8 Length of linked list: 3 Adding elements to the back. 58 >33 58 >33- 8-- 1-- 34 58 >33 Length of linked list: 6 Removing elements from the front... 33 8- 1 -->34 ->20 Length of linked list: 4 Removing elements from the back. >34 >1 >1 Length of linked list: 2 Stack Pushing elements 33 8 58- 33- 8 Height of stack: 3 Popping elements Popped: 58 33 >8 Popped: 33 Popped: 8 Queue 17 >17 102-- 17- 2 Length of queue: 3 De queued: 2 102- 17 De queued: 17 Dequeued: 102
Step by Step Solution
There are 3 Steps involved in it
Step: 1
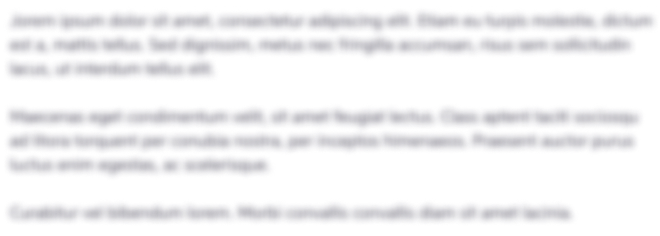
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started