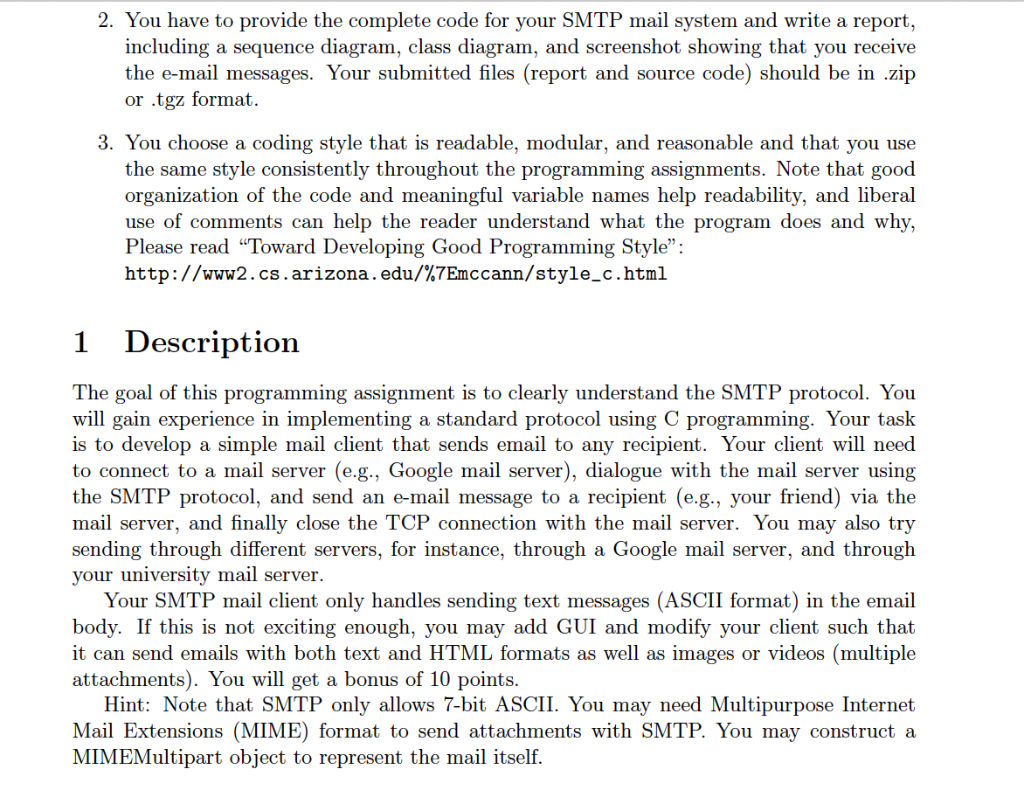
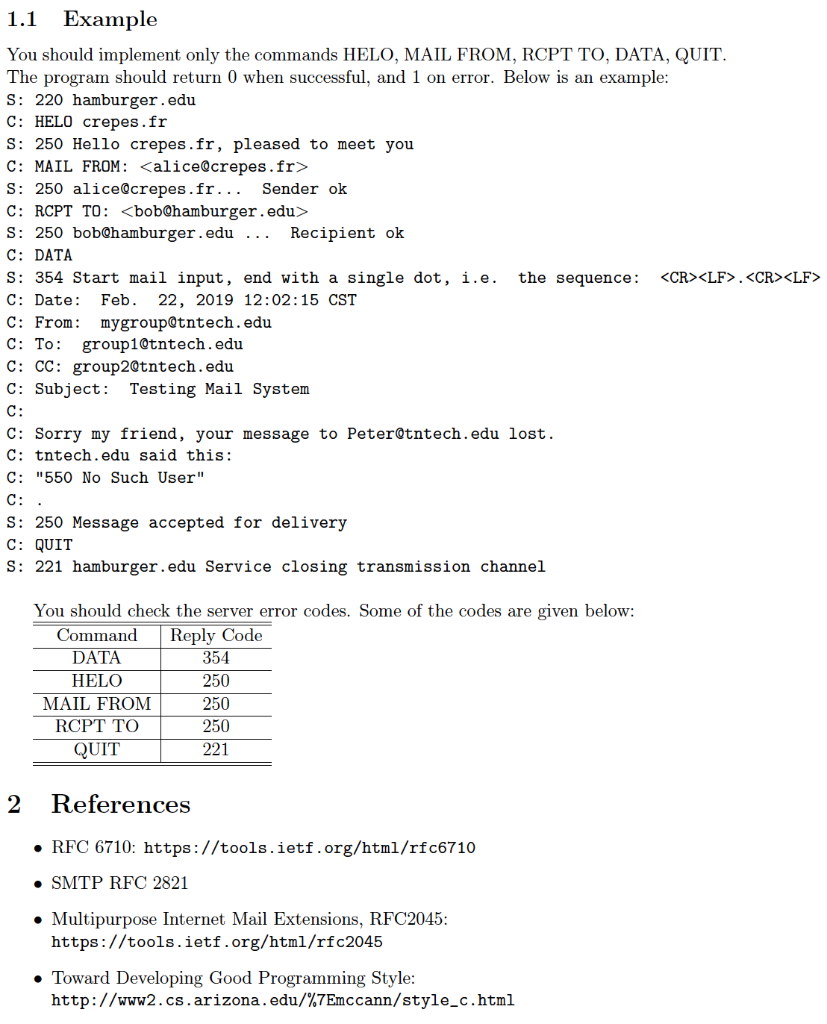
2. You have to provide the complete code for your SMTP mail system and write a report including a sequence diagram, class diagram, and screenshot showing that you receive the e-mail messages. Your submitted files (report and source code) should be in .zip or .tgz format. 3. You choose a coding style that is readable, modular, and reasonable and that you use the same style consistently throughout the programming assignments. Note that good organization of the code and meaningful variable names help readability, and liberal use of comments can help the reader understand what the program does and why, Please read "Toward Developing Good Programming Style": http://www2.cs.arizona.edu/i,7Emccann/style_c.html Description The goal of this programming assignment is to clearly understand the SMTP protocol. You will gain experience in implementing a standard protocol using C programming. Your task is to develop a simple mail client that sends email to any recipient. Your client will need to connect to a mail server (e.g., Google mail server), dialogue with the mail server using the SMTP protocol, and send an e-mail message to a recipient (e.g., your friend) via the mail server, and finally close the TCP connection with the mail server. You may also try sending through different servers, for instance, through a Google mail server, and through your university mail server. Your SMTP mail client only handles sending text messages (ASCII format) in the email body. If this is not exciting enough, you may add GUI and modify your client such that it can send emails with both text and HTML formats as well as images or videos (multiple attachments). You will get a bonus of 10 points Hint: Note that SMTP only allows 7-bit ASCII. You may need Multipurpose Internet Mail Extensions (MIME) format to send attachments with SMTP. You may construct a MIMEMultipart object to represent the mail itself 1.1 Example You should implement only the commands HELO, MAIL FROM, RCPT TO, DATA, QUIT. The program should return 0 when successful, and 1 on error. Below is an example S: 220 hamburger.edu C HELO crepes.fr S: 250 Hello crepes.fr, pleased to meet you C: MAIL FROM:
S: 250 aliceQcrepes.fr. .. Sender ok C: RCPT TO: S: 250 bobOhamburger. edu .. . Recipient ok C: DATA S: 354 Start mail input, end with a single dot, i.e. the sequence: " C: Date Feb. 22, 2019 12:02:15 CST C: From: mygroup@tntech.edu C: To: group1Otntech.edu C: CC: group2Qtntech.edu C: Subject: Testing Mail System C: C: Sorry my friend, your message to Petertntech.edu lost C: tntech.edu said this: C: "550 No Such User" S: 250 Message accepted for delivery C: QUIT S: 221 hamburger.edu Service closing transmission channel You should check the server error codes. Some of the codes are given below: Command Reply Code DATA HELO MAIL FROM RCPT TO QUIT 354 250 250 250 221 2 References . RFC 6710: https://tools.ietf.org/html/rfc6710 SMTP RFC 2821 Multipurpose Internet Mail Extensions, RFC2045 https://tools.ietf.org/html/rfc2045 Toward Developing Good Programming Style http://www2.cs.arizona.edu/%7Emccann/style_c.html 2. You have to provide the complete code for your SMTP mail system and write a report including a sequence diagram, class diagram, and screenshot showing that you receive the e-mail messages. Your submitted files (report and source code) should be in .zip or .tgz format. 3. You choose a coding style that is readable, modular, and reasonable and that you use the same style consistently throughout the programming assignments. Note that good organization of the code and meaningful variable names help readability, and liberal use of comments can help the reader understand what the program does and why, Please read "Toward Developing Good Programming Style": http://www2.cs.arizona.edu/i,7Emccann/style_c.html Description The goal of this programming assignment is to clearly understand the SMTP protocol. You will gain experience in implementing a standard protocol using C programming. Your task is to develop a simple mail client that sends email to any recipient. Your client will need to connect to a mail server (e.g., Google mail server), dialogue with the mail server using the SMTP protocol, and send an e-mail message to a recipient (e.g., your friend) via the mail server, and finally close the TCP connection with the mail server. You may also try sending through different servers, for instance, through a Google mail server, and through your university mail server. Your SMTP mail client only handles sending text messages (ASCII format) in the email body. If this is not exciting enough, you may add GUI and modify your client such that it can send emails with both text and HTML formats as well as images or videos (multiple attachments). You will get a bonus of 10 points Hint: Note that SMTP only allows 7-bit ASCII. You may need Multipurpose Internet Mail Extensions (MIME) format to send attachments with SMTP. You may construct a MIMEMultipart object to represent the mail itself 1.1 Example You should implement only the commands HELO, MAIL FROM, RCPT TO, DATA, QUIT. The program should return 0 when successful, and 1 on error. Below is an example S: 220 hamburger.edu C HELO crepes.fr S: 250 Hello crepes.fr, pleased to meet you C: MAIL FROM: S: 250 aliceQcrepes.fr. .. Sender ok C: RCPT TO: S: 250 bobOhamburger. edu .. . Recipient ok C: DATA S: 354 Start mail input, end with a single dot, i.e. the sequence: " C: Date Feb. 22, 2019 12:02:15 CST C: From: mygroup@tntech.edu C: To: group1Otntech.edu C: CC: group2Qtntech.edu C: Subject: Testing Mail System C: C: Sorry my friend, your message to Petertntech.edu lost C: tntech.edu said this: C: "550 No Such User" S: 250 Message accepted for delivery C: QUIT S: 221 hamburger.edu Service closing transmission channel You should check the server error codes. Some of the codes are given below: Command Reply Code DATA HELO MAIL FROM RCPT TO QUIT 354 250 250 250 221 2 References . RFC 6710: https://tools.ietf.org/html/rfc6710 SMTP RFC 2821 Multipurpose Internet Mail Extensions, RFC2045 https://tools.ietf.org/html/rfc2045 Toward Developing Good Programming Style http://www2.cs.arizona.edu/%7Emccann/style_c.html