Question
21. Which of the following classes handles reading from a file? A. inputstream B. iostream C. ifstream D. ofstream 22. Passing by reference is also
21. Which of the following classes handles reading from a file?
A. inputstream
B. iostream
C. ifstream
D. ofstream
22. Passing by reference is also an effective way to allow a function to return more than one value.
A. True
B. False
23. Consider the following program:
int operate (int a, int b)
{
return (a*b);
}
float operate (float a, float b)
{
return (a/b);
}
int main ()
{
int x=5,y=2;
float n=5.0,m=2.0;
cout << operate (x,y);
cout << " ";
cout << operate (n,m);
cout << " ";
return 0;
}
Which line(s) of code could be used to call this function (select all that apply):
float operate (float a, float b)
A. float n=5.0,m=2.0;
cout << operate (n,m);
B. float n=5.0,m=2.0;
cout << operate (n,n);
C. int n=5,m=2;
cout << operate (n,m);
D. int n=5,m=2;
cout << operate (n,n);
24. Consider the following prototype:
void duplicate (int& a, int& b, int& c)
What do the ampersands (&) signify?
A. The ampersands signify that their corresponding arguments are to be passed by value instead of by reference.
B. The ampersands signify that their corresponding arguments are to be passed by reference instead of by value.
C. None of these. Ampersands are not allowed in a function prototype.
D. The ampersands signify that their corresponding arguments are to be passed as a constant (read-only) value.
25. What is missing from this function?
int divide (int a, int b=2)
{
int r;
r=a/b;
}
A. &r;
B. cout << r;
C. Nothing is missing
D. return r;
26. Consider the following:
int BinarySearch(int A[], int key, int low, int high)
{
while (low <= high)
{
int m = (low + high) / 2;
if (key < A[m])
{
high = m - 1;
}
else if (key > A[m])
{
low = m + 1;
}
else
{
return m;
}
}
return -1;
}
What is the purpose of iteration in the above code?
A. To search between the low and high index numbers as long as there are index numbers between low and high to search.
B. To walk through each element of the array from the first element until the end of the array is reached.
C. To set the index point in the middle so the right hand side of the array can be searched before the left hand side is searched.
D. To test if the original array has a length greater than zero before attempting any other statements.
27. Consider the following recursive binary search function:
int search::rBinarySearch(int sortedArray[], int first, int last, int key)
{
if (first <= last) {
int mid = (first + last) / 2; // compute mid point.
if (key == sortedArray[mid])
return mid; // found it.
else if (key < sortedArray[mid])
// Calls itself for the lower part of the array
return rBinarySearch(sortedArray, first, mid-1, key);
else
// Calls itself for the upper part of the array
return rBinarySearch(sortedArray, mid+1, last, key);
}
return -1;
}
Which of the following might be described as the base case(s) for this method to end the recursion?
A. When the midpoint value is greater than the target (when this statement is true)
else if (key > sortedArray[mid])
B. when there are no elements in the specified range of indices (when this statement is false):
if (first <= last)
C. When the midpoint value is less than the target
D. when the value is found in the middle of the range (when this statement is true):
if (key == sortedArray[mid])
28. Consider the following two approaches to the binary search algorithm using the STL vector:
Approach A
int BinarySearch(vector
{
int l = 0;
int r = A.size()-1;
while (l <= r)
{
int m = (l + r) / 2;
if (key < A[m])
{
r = m - 1;
}
else if (key > A[m])
{
l = m + 1;
}
else
{
return count;
}
}
return -1;
}
Approach B
int search::BinarySearch(vector
{
if (first <= last) {
int mid = (first + last) / 2;
if (key == sortedArray[mid])
return mid;
else if (key < sortedArray[mid])
return BinarySearch(sortedArray, first, mid-1, key);
else
return BinarySearch(sortedArray, mid+1, last, key);
}
return -1;
}
Which approach uses recursion? Explain why Approach B has more parameters than Approach A.
A. Both Approach A and Approach B use recursion. The additional parameters are not necessary and should be removed to make the method implementation more readable.
B. Approach B uses recursion. Approach B has first and last parameters because they are not defined locally in the method itself. Either defining these values in the parameters or locally in the method is a matter of preference and has no bearing on the outcome. These values are needed to define the search area, either by increasing the value of first or decreasing the value of last.
C. Approach B uses recursion. Approach B includes the additional first and last parameters because they are required in the process of recursion to reduce the search area, either by increasing the value of first or decreasing the value of last when a recursive call is made.
D. Approach A uses recursion. Approach B requires the first and last parameters because they are needed in the process of iteration to reduce the search area, either by increasing the value of first or decreasing the value of last in the argument list when the iteration is repeated.
29. Consider the following code. Assume that the program includes iostream and uses namespace std.
Why would you get identifier not found errors?
int main ()
{
int i;
do {
cout << "Type a number (0 to exit): ";
cin >> i;
odd (i);
} while (i!=0);
return 0;
}
void odd (int a)
{
if ((a%2)!=0) cout << "Number is odd. ";
else even (a);
}
void even (int a)
{
if ((a%2)==0) cout << "Number is even. ";
else odd (a);
}
A. Because the functions even and odd are not declared prior to the function calls. Their prototypes are needed above main:
void odd (int a);
void even (int a);
B. Because the function calls do not match the function definitions.
C. Because the variable i is not initialized to a valid integer value before it is used.
D. Because variable a is not defined in the body of the even and odd functions.
30. You have an array of structs that is defined as follows.
struct pairT {
std::string key;
int val;
};
Which of the following could be used to add a new struct to the array called entries in position 0?
A.
pairT p;
p.key = "Bob";
p.val = 12345;
entries[0] =p;
B.
pairT p;
p = "Bob";
p = 12345;
entries[0] =p;
C.
pairT p;
entries[0] = {key = "Bob"; val = 12345};
D.
pairT p;
p.key = "Bob" && p.val = 12345;
entries.0 =p;
31. Consider the following:
struct database {
int id_number;
int age;
float salary;
};
How would you set the age variable equal to 22?
A.
database.age = 22;
B.
database.age(22);
C.
database employee;
employee.age = 22;
D.
age = 22;
32. What is wrong with this function?
void swapShellsFirstInArray(int &basket[5])
{
int temp = basket[0];
basket[0] = basket[1];
basket[1] = temp;
}
A. The code will only work with a vector, not an array
B. The return type should not be void
C. The array size is too large in the parameter list
D. You cannot pass an array by reference
33. I have declared the following function:
int findfirst(int a[][7])
{
return a[0][0];
}
Here is my main function:
int main()
{
int myarray[1][1] = {1};
int first = findfirst(myarray);
}
What is wrong?
A. The first variable in main stores the return value of findfirst, which is an integer array, not an integer.
B. The array is too small for the findfirst function. It must have 7 columns.
C. The array is empty when the findfirst function is called.
D. The array in main is not declared properly. It should be named a, not myarray.
E. The array is too small for the findfirst function. It must have 7 rows..
34. The std::binary_search function returns the index number of the val if it is found. Otherwise, it returns -1.
A. True
B. False
Step by Step Solution
There are 3 Steps involved in it
Step: 1
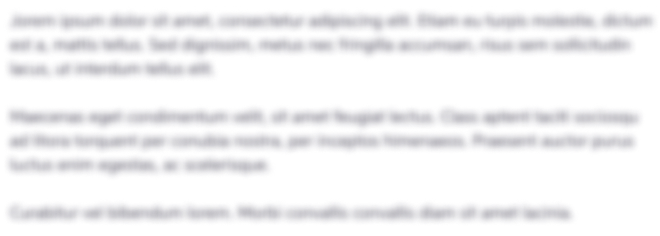
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started