Question
28. Consider the code snippet below: class Question { public: virtual void display() const; virtual void display(ostream& out) const; . . . }; class ChoiceQuestion
28. Consider the code snippet below:
class Question { public: virtual void display() const; virtual void display(ostream& out) const; . . . }; class ChoiceQuestion : public Question { public: void display(); . . . };
What change should be made to allow ChoiceQuestion::display() to override Question::display()?
Group of answer choices
ChoiceQuestion::display() needs to be explicitly declared public.
ChoiceQuestion::display() needs to be const.
ChoiceQuestion::display() needs to be explicitly declared virtual.
No change needed, as it already overrides Question::display().
33. What does the following code do?
istream& operator>>(istream& in, Time& a) { int hours, minutes; char separator; in >> hours; in.get(separator); // Read : character in >> minutes; a = Time(hours, minutes); return in; }
Group of answer choices
Creates a Time object from the user (or file) input
All of these
Allows reading multiple Time objects in one statement such as cin >> Time1 >> Time2;
Redefines the > > operator
34. Which of the following non-member function headers best fits the post-increment operator for an object, such as
Time t(1,30); Time x = t++;
Group of answer choices
Time operator++(Time& a, int i)
void operator++(Time a)
Time operator++(Time a)
Time operator++(Time& a)
35.Which of the following class member function headers best fits the pre-increment operator for an object, such as
Time t(1,30); Time x = ++t;
Group of answer choices
Time Time::operator++(Time& a)
Time Time::operator++(int i)
Time Time::operator++()
Time Time::operator++(Time& a, int i)
37.Which of the following function headers best fits a type conversion operator to convert an object of the Fraction class to type double, so that one can use implicit type conversions such as
double root = sqrt( Fraction f(1,2));
Group of answer choices
double operator double(Fraction f) const
Fraction::operator double() const
double convert(Fraction f) const
double Fraction::operator() const
38. What if anything is wrong with the following class definition?
class Fraction //represents fractions of the type 1/2, 3/4, 17/16 { public: Fraction(int n, int d); Fraction(int n); Fraction(); double operator+(Fraction other) const; double operator-(Fraction other) const; double operator*(Fraction other) const; bool operator<(Fraction other) const; void print(ostream& out) const; // other member functions exist but not shown private: int numerator; int denominator; };
Group of answer choices
Nothing is wrong
The operator functions should return type Fraction
Not all the constructors are needed
It is missing a private double data member to represent the decimal value of the fraction
Step by Step Solution
There are 3 Steps involved in it
Step: 1
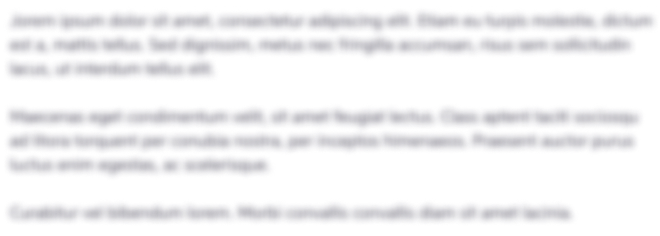
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started