Question
3 files - Matrix.java(completed), Oribit . pde , Orbiter.java. Instructions are given inside the code. Please complete the codes.( Oribit.pde, Orbiter.java ) Matrix.java ( Completed
3 files - Matrix.java(completed), Oribit.pde, Orbiter.java. Instructions are given inside the code. Please complete the codes.( Oribit.pde, Orbiter.java )
Matrix.java ( Completed )
public class Matrix {
private int m,n;
private double[][] M;
//constructor
public Matrix(double[][] array) {
M = array;
m = array.length;
n = array[0].length;
}
//get number of columns
public int nCols() {
return n;
}
//get number of rows
public int nRows() {
return m;
}
//get ith jth entry of this matrix
public double entry(int i, int j) {
return M[i][j];
}
//compute dot product of two matrix
public Matrix dot(Matrix that) throws UndefinedMatrixOpException {
int m1 = this.nRows();
int n1 = this.nCols();
int m2 = that.nRows();
int n2 = that.nCols();
if(n1 != m2) {
throw new UndefinedMatrixOpException("Dimensions are not equal");
}
double[][] c = new double[m1][n2];
for (int i = 0; i < m1; i++)
for (int j = 0; j < n2; j++)
for (int k = 0; k < n1; k++)
c[i][j] += this.M[i][k] * that.M[k][j];
Matrix t = new Matrix(c);
return t;
}
//compute addition of two matrices
public Matrix plus(Matrix that) throws UndefinedMatrixOpException {
int m1 = this.nRows();
int n1 = this.nCols();
int m2 = that.nRows();
int n2 = that.nCols();
if(m1 != m2 || n1 != n2) {
throw new UndefinedMatrixOpException("Dimensions are not equal");
}
double[][] c = new double[m1][n1];
for (int i = 0; i < m1; i++)
for (int j = 0; j < n1; j++)
c[i][j] = this.M[i][j] + that.M[i][j];
Matrix t = new Matrix(c);
return t;
}
//return rotational matrix
public static Matrix rotationH2D(double theta) {
double[][] R = {{Math.cos(theta), -Math.sin(theta), 0},
{Math.sin(theta), Math.cos(theta), 0},
{0,0,1}};
return new Matrix(R);
}
//return translational matrix
public static Matrix translationH2D(double tx, double ty) {
double[][] R = {{1, 0 , 0},
{0, 1, 0},
{tx, ty, 1}};
return new Matrix(R);
}
//return scaling matrix
public static Matrix vectorH2D(double x, double y) {
double[][] R = {{x, 0 , 0},
{0, y, 0},
{0, 0, 1}};
return new Matrix(R);
}
//return identity matrix
public static Matrix identity(int n) {
double[][] R = new double[n][n];
for (int i = 0; i < n; i++)
R[i][i] = 1;
return new Matrix(R);
}
public String toString() {
StringBuilder sb = new StringBuilder();
for (int i = 0; i < m; i++) {
for (int j = 0; j < n; j++) {
sb.append(M[i][j]);
sb.append('\t');
}
sb.append(' ');
}
return sb.toString();
}
}
Orbit.pde (Not complete) - Has to follow instructions in the code
import java.util.*; import java.awt.*;
Orbiter sun; // The root orbiter int lastMillis; double[][] originM = {{0},{0},{1}}; Matrix origin = new Matrix(originM);
// A few fun parameters boolean clearBackground = true; double speedModifier = 1.0; // Set in some of the scenes to change the speed.
// One default scene (selected in the setup() function void setupScene1() { sun = new Orbiter(null, 0, 0, 0, Orbiter.Type.CIRCLE, Color.yellow); Orbiter earth = new Orbiter(sun, 50, 0, 1, Orbiter.Type.CIRCLE, Color.blue); Orbiter moon = new Orbiter(earth, 30, 0, 1, Orbiter.Type.CIRCLE, Color.gray); Orbiter moonSatellite = new Orbiter(moon, 20, 0, 1, Orbiter.Type.CIRCLE, Color.gray); Orbiter jupiter = new Orbiter(sun, 200, 0, 0.5, Orbiter.Type.CIRCLE, Color.red); Orbiter jupiterMoon = new Orbiter(jupiter, 75, 0, 2, Orbiter.Type.CIRCLE, Color.green); Orbiter jupiterExplorer = new Orbiter(jupiterMoon, 40, 0, -1, Orbiter.Type.SQUARE, Color.orange); Orbiter jupiterExplorerRobot = new Orbiter(jupiterExplorer, 20, 0, -3, Orbiter.Type.TRIANGLE, Color.magenta); } // A second default scene void setupScene2() { speedModifier = 0.25; sun = new Orbiter(null, 0, 0, 0, Orbiter.Type.TRIANGLE, Color.yellow); Orbiter earth = new Orbiter(sun, 50, 0, 10, Orbiter.Type.TRIANGLE, Color.blue); Orbiter moon = new Orbiter(earth, 30, 0, 20, Orbiter.Type.TRIANGLE, Color.gray); Orbiter moonSatellite = new Orbiter(moon, 20, 0, 30, Orbiter.Type.TRIANGLE, Color.gray); Orbiter jupiter = new Orbiter(sun, 200, 0, 5, Orbiter.Type.TRIANGLE, Color.red); Orbiter jupiterMoon = new Orbiter(jupiter, 75, 0, 2, Orbiter.Type.TRIANGLE, Color.green); Orbiter jupiterExplorer = new Orbiter(jupiterMoon, 40, 0, 8, Orbiter.Type.TRIANGLE, Color.orange); Orbiter jupiterExplorerRobot = new Orbiter(jupiterExplorer, 20, 0, -6, Orbiter.Type.TRIANGLE, Color.magenta); }
// The setup. You don't need to edit this other than to switch scenes by commenting out // the setupScene1() and uncommenting setupScene2(). void setup() { size(800, 800); background(0); setupScene1(); //setupScene2(); // Run this one with clearBackground set to false lastMillis = millis(); }
// The draw function // DO NOT EDIT void draw() { if (clearBackground) background(0); // Make the background black. int currentMillis = millis(); // Get the current number of milliseconds int elapsedMillis = currentMillis - lastMillis; // Get the number of milliseconds elapsed since last call double timeDelta = elapsedMillis / 1000.0; updateOrbiters(timeDelta * speedModifier); pushMatrix(); scale(1, -1); translate(width / 2, - height / 2); drawOrbiters(); popMatrix(); lastMillis = currentMillis; }
void updateOrbiters(double timeDelta) { // TODO // This code should traverse the orbiters (in BFS or DFS, but I used BFS) // order using a stack or a queue (your choice), and call updateRotation // on each one using the timeDelta parameter. // // Recall that Java has a Queue
void drawOrbiters() { // TODO // This code should traverse the orbiters (in BFS or DFS order, i used BFS) // and call drawOrbiter on each orbiter. }
// The code for drawing an orbiter. This is called from your drawOrbiters() method // but you should not have to edit it. void drawOrbiter(Orbiter orbiter) { try { Matrix position = orbiter.getMatrix().dot(origin); int px = (int) Math.round(position.entry(0,0) / position.entry(2,0)); int py = (int) Math.round(position.entry(1,0) / position.entry(2,0)); // Draw the orbiter noStroke(); fill(orbiter.getFillColor().getRed(), orbiter.getFillColor().getGreen(), orbiter.getFillColor().getBlue()); switch (orbiter.getType()) { case CIRCLE: ellipse(px, py, 16, 16); break; case SQUARE: rect(px-4, py-4, 8, 8); break; case TRIANGLE: triangle(px, py+3, px-2, py-1, px+2, py-1); break; } noFill(); // Draw the orbit path if (clearBackground) { stroke(60); for (Orbiter child : orbiter.getChildren()) { int radius = (int) (2*child.getOrbitRadius()); ellipse(px, py, radius, radius); } } } catch (UndefinedMatrixOpException umoe) { } }
Orbiter.java (Not complete) - Has to follow instructions in the code
import java.awt.Color;
import java.util.*;
/**
* An Orbiter is an object that orbits some other object, called its
parent.
* The center of an orbital system is an Orbiter with no parent.
* Each Orbiter may have child Oribters that orbit it.
*
* An Orbiter stores its orbital radius and current orbit angle.
*/
public class Orbiter {
public enum Type {
CIRCLE, SQUARE, TRIANGLE
}
private final double orbitRadius;
private final Type type;
private final Color fillColor;
private double orbitAngle;
private double orbitSpeed;
private final List
private final Orbiter parent;
public Orbiter(Orbiter parent, double orbitRadius, double orbitAngle,
double orbitSpeed, Type type, Color fillColor) {
this.orbitRadius = orbitRadius;
this.orbitAngle = orbitAngle;
this.type = type;
this.fillColor = fillColor;
this.parent = parent;
this.orbitSpeed = orbitSpeed;
if (parent != null) parent.children.add(this);
}
public double getOrbitRadius() { return orbitRadius; }
public double getOrbitAngle() { return orbitAngle; }
public Color getFillColor() { return fillColor; }
public Type getType() { return type; }
public Orbiter getParent() { return parent; }
public List
/**
* Updates the rotation of this orbiter by the amount specified in the
deltaAngle parameter.
* @param deltaAngle The amount of rotation angle to add the to the
current rotation.
*/
public void updateRotation(double timeDelta) {
orbitAngle += (timeDelta * orbitSpeed);
}
public Matrix getMatrix() throws UndefinedMatrixOpException {
// TODO
// If this is the root node, then return the 3x3 identity matrix
// If this is not the root node, should return the transformation
// matrix for this orbiter (see the writeup for an idea of how to
// do this). Make sure you've coded the Matrix class first.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
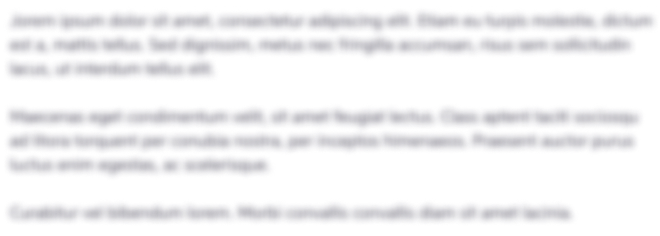
Get Instant Access with AI-Powered Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started