Question
3. In the program figurespointers we have a base class location and various derived classes: circle, triangle, rectangle. Complete the implementation of the derived classes.
3. In the program figurespointers we have a base class location and various derived classes:
circle, triangle, rectangle. Complete the implementation of the derived classes.
For marking purposes, run the program entering: circle(1,2,3), triangle(3,4,1,2,1) and rectangle(5,6,3,4). Explain why this program does not work properly.
Change the classes (not main) so that the program runs properly and run the program with the same data as before.
Declaration of Classes FigurePointers
This is the copy paste code for this code: Declaration of Classes Figure Pointers:
/* File: figurepointers.h
*/
#ifndef FIGUREPOINTERS_H
#define FIGUREPOINTERS_H
#include
#include
#include
#include
using namespace std;
class location {
private:
float x; // position of the figure
float y;
public:
void read(istream & in) ;
void write(ostream & out) const;
virtual float area(void) const;
};
class circle : public location {
private:
float radius;
public:
void read(istream & in);
void write(ostream & out) const;
float area(void) const; // area of the figure;
};
class rectangle : public location {
private:
float side1, side2;
public:
void read(istream & in);
void write(ostream & out) const;
float area(void) const; // area of the figure;
};
class triangle : public rectangle {
private:
float angle;
public:
void read(istream & in);
void write(ostream & out) const;
float area(void) const; // area of the figure;
};
#endif /* FIGUREPOINTERS_H */
Implementation of Class FigurePointers:
/* File: figurepointers.cpp
Implementation of class figurepointers */
#include "figurepointers.h"
////////////////// implementation of location /////// ///////////
void location::read(istream & in)
{
cout
in >> x;
cout
in >> y;
}
float location::area(void) const
{
return 0.0;
}
void location::write(ostream & out) const
{
out
out
out
}
////////////////// implementation of circle //////////////////
////////////////// implementation of triangle //////////////////
////////////////// implementation of rectangle //////////////////
Main Program Using Classes FigurePointers:
/* File: testfigurepointers.cpp
Application program using classes figurepointers
Programmer: your name Date: */
#include "figurepointers.h"
int main(void)
{
string type; // figure type
ofstream fout ("testfigurepointersout.txt");
location * p;
while(true) { // loop until break occurs
cout > type;
if(type == "circle") {
p = new circle;
p->read(cin);
fout
p->write(fout);
delete p;
} else if (type == "triangle") {
p = new triangle;
p->read(cin);
fout
p->write(fout);
delete p;
} else if (type == "rectangle") {
p = new rectangle;
p->read(cin);
fout
p->write(fout);
} else break; // we are done entering data
}
fout.close();
return 0;
}
Declaration of Classes FigurePointers File: figurepointers.h #ifnd ef FIGUREPOINTERS.H #define FIGUREPOINTERS.H #include
Step by Step Solution
There are 3 Steps involved in it
Step: 1
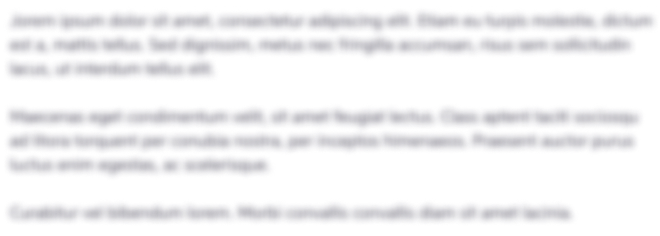
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started