Question
3. Write a test program (i.e. main) to do the following: Step 1: Create a cargo terminal object o Contains a loading dock (array) with
3. Write a test program (i.e. main) to do the following: Step 1: Create a cargo terminal object o Contains a loading dock (array) with the number of docks read from the trucks file o Contains a tarmac (array) with the number of stands read from the planes file.
Step 2: Load cargo terminal with all semi-trucks and cargo planes in the files o For each semi-truck in the truck file: o Read details for the semi-truck from the file. o Create a semi-truck object using the details. o Add semi-truck to cargo terminals loading dock in location specified in file o Use the addSemiTruck method in the CargoTerminal class o For each cargo plane in the plane file: o Read details for the cargo plane from the file. o Create a cargo plane object using the details. o Add cargo plane to cargo terminals tarmac in location specified in file o Use the addCargoPlane method in the CargoTerminal class
Step 4: Display the cargo terminal. o Display a nicely formatted version of the cargo terminal o Use the displayTrucksAndPlanes() method in the CargoTerminal class Step 3: Print a status report for the tarmac. Write a method to print the status: public static void printTarmacStatus (CargoTerminal terminal)
This method must: Be placed inside your Assignment4 class after main Print the details for each plane on the tarmac o For each cargo plane create one PlaneReport o Place all PlaneReports into an ArrayList o Sort all PlaneReports in the ArrayList using Collections.sort() o Print all PlaneReports in the ArrayList (now sorted by capacity) i. Call print() method on PlaneReport ii. See the Tarmac Status output example below
4. Test file information a. Run your code on the 2 provided files FedExTrucks.txt and FedExPlanes.txt. The files are examples so DO NOT assume that your code should only work for a loading dock with 4 docks and a tarmac with 6 stands and/or these semi-trucks and cargo planes in this specific order. The grader files will be different.
FedExTrucks.txt 4 Number of docks the loading dock contains 1 688 Memphis 3 820 Denver Details for each semi-truck 4 728 Dallas
Note: - Dock 0 is NOT used - Dock 2 is empty!
b. 1 line is the number of docks (i.e. slots) that the loading dock contains c. Remaining lines contain details for each semi-truck. The format is as follows: Dock# Truck# Destination City 1 688 Memphis
FedExPlanes.txt 6 Number of stands tarmac contains 1 7654 Boeing767 120000 London 3 1234 MD11 180000 Barcelona Details for each cargo plane 4 517 Boeing777 230000 Frankfurt 6 112 Boeing757 60000 Jackson Note: - Slot 0 is NOT used - Slots 2 and 5 are empty!
a. 1 line is the number of stands (i.e. slots) that the tarmac contains b. Remaining lines contain details for each cargo plane. The format is as follows: Stand # Flight# Type of Plane Capacity Destination
1 7654 Boeing767 120000 London Interfaces and Classes Printable Interface Description o This interface represents an objects ability to print details about itself
Public Methods o public String print() - returns string with the details an object wants to display, in a nice format
CargoTerminal Class Description o Represents a cargo terminal and its loading dock and tarmac. o The cargo terminal contains one loading dock that is modeled by an array. o The cargo terminal contains one tarmac that is modeled by an array. Slot 0 is not used in either array The 0 slot is still part of the array, but we wont be placing semi-trucks or cargo planes into that location. In the test file, the loading dock has 5 docks (0-4) and the tarmac has 7 stands (0-6). BUT the loading dock is represented ONLY by docks 1-4 and the tarmac by slots 1-6 as shown (empty slots are represented with - - - - - -)
Dock # 1 Dock # 2 Dock # 3 Dock # 4 688 ------ 820 728 Stand # 1 Stand # 2 Stand # 3 Stand # 4 Stand # 5 Stand # 6 7654 ------ 1234 517 ------ 112
Tip: General One way to return nicely formatted strings in the print methods is to use string format method. o For example: return String.format("%4d\t\t%2d\t\t%-15s\t\t%-10s\t%.2f", flightNumber, stand, destinationCity, type, capacity);
o See chapter 4 in your book for details on format specifiers
Output Your output will look like the following when running against the test files FedExTrucks.txt and FedExPlanes.txt Loading semi-trucks and planes into cargo terminal... Dock #1 Dock #2 Dock #3 Dock #4 688 ------ 820 728 Stand #1 Stand #2 Stand #3 Stand #4 Stand #5 Stand #6 7654 ------ 1234 517 ------ 112
Dashes represent an empty slot in loading dock or tarmac arrays
********************************************************************************** Tarmac Status (Lowest to Highest Capacity) **********************************************************************************
Flight Stand Departing To Type Capacity(lbs)
112 6 Jackson Boeing757 60000.00 7654 1 London Boeing767 120000.00 1234 3 Barcelona MD11 180000.00 517 4 Frankfurt Boeing777 230000.00
FedExTrucks.txt
5 1 688 Memphis 3 820 Denver 4 728 Dallas
FedExPlanes.txt
7 1 7654 Boeing767 120000 London 3 1234 MD11 180000 Barcelona 4 517 Boeing777 230000 Frankfurt 6 112 Boeing757 60000 Jackson
Bellow is what I have done so far:
import java.io.BufferedReader;
import java.io.File;
import java.io.FileNotFoundException;
import java.io.FileReader;
import java.io.IOException;
import java.util.Scanner;
public class NgocHuanAssignment4 {
public interface Printable {
String print();
}
public class CargoTerminal{
private SemiTruck [] loadingDock;
private CargoPlane [] tarmac;
private int numberDocks;
private int numberStands;
public CargoTerminal( int numberDocks, int numberStands) {
this.numberDocks = numberDocks;
this.numberStands = numberStands;
loadingDock = new SemiTruck[numberDocks];
tarmac = new CargoPlane[numberStands];
}// end constructor
public int getNumberDocks() {
return numberDocks;
}// end getter
public int getNumberStands() {
return numberStands;
}// end getter
public void addSemiTruck(int dock, SemiTruck semiTruck) {
// place incoming semitruck object in loading dock array
loadingDock[dock] = semiTruck;
System.out.println("Semi-truck parked at dock " + dock);
} //end method addSemiTruck
// Simulates parking a semitruck at a specific loading dock at cargo terminal
public void addCargoPlane(int stand, CargoPlane plane) {
// place incoming cargo plane object in loading stand array
tarmac[stand] = plane;
System.out.println("Cargo plane park at stand" + stand);
}// end method addCargoPlane
public SemiTruck getSemiTruck(int dock) {
return loadingDock[dock];
}// end Semitruck
public CargoPlane getPlane(int stand) {
return tarmac[stand];
}// end cargoPlane
public void displayTruckAndPlane() {
System.out.println("Loading Dock:");
for(int i=1; i SemiTruck semiTruck = loadingDock[i]; if (semiTruck !=null) { System.out.println("Dock #" + i + ":" + semiTruck.getTruckNumber()); }//end if statement }// end for loop // Pint stand # and flight number System.out.println(" Tarmac:"); for(int i =1; i CargoPlane plane = tarmac[i]; if(plane != null) { System.out.println("Stand # "+ i +":" + plane.getFlightNumber()); }// end if statement }// end for loop }// end display method }// end CargoTerminal Class // start class SemiTruck public class SemiTruck { private int truckNumber; private String destinationCity; // start constructor public SemiTruck(int truckNumber, String destinationCity) { this.truckNumber = truckNumber; this.destinationCity = destinationCity; }// end constructor // getter for each data field public int getTruckNumber() { return truckNumber; }// end getter trucknumber public String getDestinationCity() { return destinationCity; }// end getter Destination }// end class SemiTruck //Start CargoPlane class public class CargoPlane{ private int flightNumber; private String type; private double capacity; private String destinationCity; // start constructor public CargoPlane(int flightNumber, String type, double capacity, String destinationCity) { this.flightNumber = flightNumber; this.type = type; this.capacity = capacity; this.destinationCity = destinationCity; }// end constructor // getter for each data public int getFlightNumber() { return flightNumber; }// end getter flight number public String getType() { return type; }//end getter type public double getCapacity() { return capacity; }// end getter capacity public String getDestinationCity() { return destinationCity; }// end getter destination }// end class Cargoplane class // start class Planereport public class PlaneReport implements Printable, Comparable private int stand; private int flightNumber; private String type; private double capacity; private String destinationCity; // start public method constructor public PlaneReport(int stand, int flightNumber, String type, double capacity, String destinationCity) { this.stand = stand; this.flightNumber = flightNumber; this.type = type; this.capacity = capacity; this.destinationCity = destinationCity; }// end constructor // Override Printable method @Override public String print() { return String.format("%4d\t\t%2d\t\t%-15s\t\t%-10s\t%.2f", flightNumber, stand, destinationCity, type, capacity); }// end override //Override Comparable @Override public int compareTo(PlaneReport otherReport) { if(this.capacity < otherReport.capacity) { return -1; } else if(this.capacity > otherReport.capacity) { return 1; } else { return 0; } // end if else statement }// end override }// end class PlaneReport public static void main(String[] args) throws FileNotFoundException { } } // end java class PLEASE HELP WITH THIS ISSUE
Step by Step Solution
There are 3 Steps involved in it
Step: 1
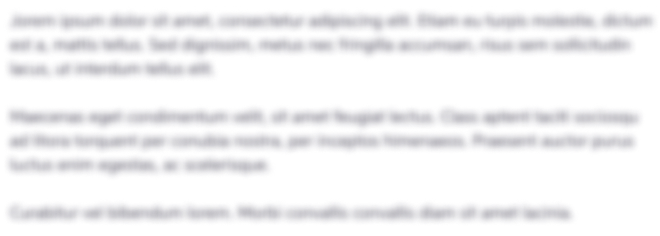
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started