Question
3.1 The interface for the student class discussed in class is expanded with additional member and non-member functions and the code is provided below: class
3.1 The interface for the student class discussed in class is expanded with additional member and non-member functions and the code is provided below:
class student
{
public:
student();
// default constructor
// Postcondition: The newly declared object is initialized according to the code of the constructor.
student(string nm, int nc, double gpa);
// 2nd constructor
// Postcondition: The data members of the newly declared object are initialized with the values passed to the function
string getName();
// An accessor function
// Postcondition: to be filled in by you
double getGPA();
// Another accessor function
// Postcondition: to be filled in by you
void inputOneStuRec();
// The function prompts the user to enter name, total credits, and GPA for a student
// Postcondition: date members of the calling object are loaded with values entered by the user
void setStuRec(string nm, int nc, double gpa);
// Postcondition: to be filled in by you
void displayOneStuRec();
// Postcondition: to be filled in by you
private:
string name;
int tCredits;
double GPA;
};
int fillArray(student s[], int size)
{
int n; // used to store the actual number of student records entered by the user
cout
cin >> n;
cout
for (int i = 0; i
{
cout
s[i].inputOneStuRec();
}
cout
return n;
}
// ******************************
// Prototype of non-member function
int fillArray(student s[], int size);
// An external function, i.e., it is not a member function of the student class!
// Postcondition: 1) returns n, which is the actual number of student record entered by the user
// 2) array is loaded with n sets of values entered the user
void displayStuRecs(student s[], int n);
// A non-member external function. It calls a public member function displayOneStuRec() to get the job done
// Postcondition: The first n student records/objects stored in s array passed to the function are displayed
void sortStuRecsByName(student s[], int n);
// A non-member external function.
// Postcondition: The first n student records/objects stored in the s array are sorted by name alphabetically
void sortStuRecsByGPA(student s[], int n);
// Postcondition: The first n student records/objects stored in the s array are sorted by GPA in descending order
void swap(student &s1, student &s2);
// s1 and s2 must be declared as reference parameters. When the function gets called, s1 and s2 refer back to the two student objects or
// records declared and defined in the calling function
// Postcondition: Two student objects (declared and defined in the calling function) that are referenced by s1 and s2 are swapped
// *******************************
You are asked to
1) Implement all member and non-member functions
2) Write a main() function that makes use of the student class above to generate an output similar to the following sample display.
You may enter up to 30 student records. How many? 3 s[0] Enter student's first name, total credits, and GPA: John 22 3.15 s[1] Enter student s first name, total credits, and GPA: Jane 45 3.68 s[2]: Enter student's first name, total credits, and GPA: Adam 78 3.47 List of 3 students before sorting Name: John GPA: 3.15 Name: Jane GPA: 3.68 Name: Adam GPA: 3.47 Credits: 22 Credits: 45 Credits: 78 We are now sorting the student list by name After sorting, the list becomes: Name: Adam GPA: 3.47 Name: Jane GPA: 3.68 Name: John GPA: 3.15 Credits: 78 Credits: 45 Credits: 22 We are now sorting the student list by GPA After sorting, the list becomes Name: Jane GPA: 3.68 Name: Adam GPA: 3.47 Name: John GPA: 3.15 Credits: 45 Credits: 78 Credits: 22
Step by Step Solution
There are 3 Steps involved in it
Step: 1
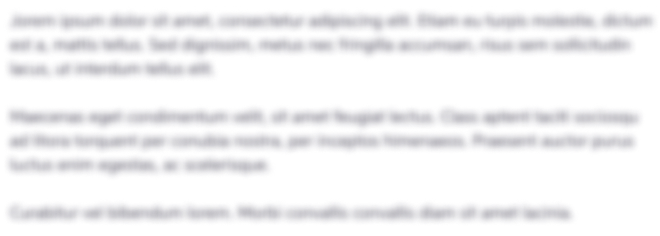
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started