Question
4. The initial values seen in the output string above are not quite right. We should start out at the top of inning 1. You
4. The initial values seen in the output string above are not quite right. We should start out at the top of inning 1. You might start with the methods getInning() and isTop() , which implies defining an instance variable to count innings, and an instance variable to keep track of whether it's the top or bottom of the inning. Once you have these initialized correctly in the constructor, the test above should give you the output: ooo Inning:1 (T) Score:0-0 Balls:0 Strikes:0 Outs:0
5. Next you could think about counting the balls, strikes, and outs. You'll need three instance variables for these three values, and their values are returned by the corresponding accessor methods getBalls(), getStrikes(), and getOuts(). Remember that accessor methods never modify instance variables, they only observe them and return information.
6. The values you have defined so far are updated in the method pitch(). Before writing this method, write a few more test cases to think about the behavior of pitch(): CS227Baseball game = new CS227Baseball(3); game.pitch(CS227Baseball.STRIKE); System.out.println(game); // should be 1 strike game.pitch(CS227Baseball.BALL); System.out.println(game); // 1 ball, 1 strike game.pitch(CS227Baseball.STRIKE); System.out.println(game); // 1 ball, 2 strikes game.pitch(CS227Baseball.STRIKE); System.out.println(game); // 0 balls, 0 strikes, 1 out game.pitch(CS227Baseball.POP_FLY); System.out.println(game); // 0 balls, 0 strikes, 2 outs (After three strikes, the batter is out, so there is a new batter starting with 0 balls and 0 strikes.) Write enough of the logic of pitch to get this test to work. Tip: to save some typing when you write these tests, at the top of your test class, add the line import static hw2.CS227Baseball.*; then you can simply write, for example, game.pitch(STRIKE);
7. Now, suppose you continue the test case above where we have two outs in the top of the first inning. When there is one more out, the teams switch, which we observe by seeing that isTop now returns false, and team 1 starts with no outs: game.pitch(POP_FLY); System.out.println(game.isTop()); // this should be false now System.out.println(game); // Bottom of 1st inning, 0 outs The final line of output above should be, ooo Inning:1 (B) Score:0-0 Balls:0 Strikes:0 Outs:0 Then, if team 1 gets three outs, the game should transition to the top of inning 2. You should be able to create a short example that transitions to the end of the game. At the end of a threeinning game, getInning() would return 4, and isOver() would return true. This almost completes the pitch method. However, in order to fully take care of the case for WALK, you'll need to implement the logic for advancing runners around the bases. (In fact, according to our rules, a walk has the same effect as hitting a single with no player out, i.e., pitchWithHit(1). So maybe you want to get that method done before coming back to pitch().)
8. The spec defines three accessor methods playerOnFirst(), playerOnSecond(), and playerOnThird() that return true or false to indicate whether there is currently a player on the indicated base. In order to implement these, you'll need instance variables to keep track of whether there is currently a player on each of the three bases. There are lots of ways to do this, but three booleans should certainly work. Then, you can start on advanceRunners(). As always, start with some simple test cases. For example, CS227Baseball game = new CS227Baseball(3); game.advanceRunners(true); System.out.println(game.playerOnFirst()); // expected true System.out.println(game.toDisplayString()); That last line should produce the output below: o - X Inning:1 (T) | | Score:0-0 o - H Balls:0 Strikes:0 Outs:0 (Remember that the getDisplayString() method just calls your accessor methods to determine what values to put in the string, so make sure those methods are working first.) If you now call advanceRunners(false), the player should move to second, without a new guy appearing on first. game.advanceRunners(false); System.out.println(game.playerOnFirst()); // false System.out.println(game.playerOnSecond()); // true System.out.println(game.toDisplayString()); X - o Inning:1 (T) | | Score:0-0 o - H Balls:0 Strikes:0 Outs:0 game.advanceRunners(false); // do it again System.out.println(game.playerOnFirst()); // false System.out.println(game.playerOnSecond()); // false System.out.println(game.playerOnThird()); // true System.out.println(game.toDisplayString()); o - o Inning:1 (T) | | Score:0-0 X - H Balls:0 Strikes:0 Outs:0 game.advanceRunners(false); // and again System.out.println(game.toDisplayString()); o - o Inning:1 (T) | | Score:1-0 o - H Balls:0 Strikes:0 Outs:0
9. Notice that if a runner gets to home, the score should increase by 1. This is not hard to implement with another instance variable.
10. At this point you'll want to write some more interesting tests for advanceRunners() that include multiple players on base. For example, what would be the result of this sequence? game.advanceRunners(true); System.out.println(game.toDisplayString()); game.advanceRunners(true); System.out.println(game.toDisplayString()); game.advanceRunners(true); System.out.println(game.toDisplayString()); game.advanceRunners(false); System.out.println(game.toDisplayString()); This corresponds to two singles followed by a double, and should produce the output, o - X Inning:1 (T) | | Score:1-0 o - H Balls:0 Strikes:0 Outs:0 X - X Inning:1 (T) | | Score:1-0 o - H Balls:0 Strikes:0 Outs:0 X - X Inning:1 (T) | | Score:1-0 X - H Balls:0 Strikes:0 Outs:0 X - o Inning:1 (T) | | Score:2-0 X - H Balls:0 Strikes:0 Outs:0 Tip: advanceRunners() is probably easiest to implement by starting at third and working backward to first and second.
11. Once you are confident about advanceRunners() (feel like you have all the bases covered, hehe), it is straightforward to implement pitchWithHit() using multiple calls to advanceRunners(). Test it.
Code has been started (JavaDoc)
package hw2;
/**
* Simplified model of American baseball.
*
* THIS CODE WILL NOT COMPILE UNTIL YOU HAVE ADDED STUBS FOR ALL METHODS
* SPECIFIED IN THE JAVADOC
*
* @author
*/
public class CS227Baseball {
/**
* Constant indicating that a pitch results in a ball.
*/
public static final int BALL = 0;
/**
* Constant indicating that a pitch results in a strike.
*/
public static final int STRIKE = 1;
/**
* Constant indicating that a pitch results in an out from a fly ball.
*/
public static final int POP_FLY = 2;
/**
* Number of strikes causing a player to be out.
*/
public static final int MAX_STRIKES = 3;
/**
* Number of balls causing a player to walk.
*/
public static final int MAX_BALLS = 4;
/**
* Number of outs before the teams switch.
*/
public static final int MAX_OUTS = 3;
/**
* Constructs a game that has the given number of innings and starts at the top
* of inning 1.
*
* @param givenNumInnings
*/
public CS227Baseball(int givenNumInnings) {
}
/**
* Advances all players on base by one base, updating the score if there is
* currently a player on third. If the argument newPlayerOnFirst is true, a
* player (i.e. the batter) is placed on first base.
*
* @param newPlayerOnFirst
*/
public void advanceRunners(boolean newPlayerOnFirst) {
}
/**
* Returns the number of balls for the current batter.
*
* @return
*/
public int getBalls() {
return 0;
}
/**
* Returns a three-character string representing the players on base, in the
* order first, second, and third, where 'X' indicates a player is present and
* 'o' indicates no player. For example, the string "XXo" means that there are
* players on first and second but not on third.
*
* @return
*/
public java.lang.String getBases() {
return null;
}
/**
* Returns the current inning. Innings are numbered starting at 1. When the game
* is over, this method returns the games total number of innings, plus one.
*
* @return
*/
public int getInning() {
return 0;
}
/**
* Returns the number of outs for the current batter.
*
* @return
*/
public int getOuts() {
return 0;
}
/**
* Returns the score of the indicated team, where an argument of true indicates
* team 0 (batting in the top of the inning) and an argument of false indicates
* team 1 (batting in the bottom of the inning). If game is a CS227Baseball
* object, the call game.getScore(game.isTop()) always returns the score for the
* team that is currently at bat.
*
* @param team0
* @return
*/
public int getScore(boolean team0) {
return 0;
}
/**
* Returns the number of strikes for the current batter.
*
* @return
*/
public int getStrikes() {
return 0;
}
/**
* Returns true if the game is over, false otherwise.
*
* @return
*/
public boolean isOver() {
return false;
}
/**
* Returns true if it's the first half of the inning (team 0 is at bat).
*
* @return
*/
public boolean isTop() {
return false;
}
/**
* Pitch not resulting in a hit. The argument for outcome should be one of the
* three predefined constants BALL, STRIKE, or POP_FLY. A detailed description
* of these values is described in the section entitled "Balls, strikes, and
* outs" in the assignment pdf. This method may update the count of balls,
* strikes, and/or outs, and may cause existing players on base to advance in
* the case of a walk, and causes the teams to switch if the number of outs
* reaches MAX_OUTS. This method does nothing if the game is over or if the
* argument is not one of the values BALL, STRIKE, or POP_FLY.
*
* @param outcome
*/
public void pitch(int outcome) {
}
/**
* Pitch resulting in a hit where no player is out. The argument for numBases
* should be 1, 2, 3, or 4, indicating a single, double, triple, or home run.
* The batter and all players currently on base must advance the given number of
* bases (possibly resulting in one or more runs). This method does nothing if
* the game is over or if the argument is not one of the values 1, 2, 3, or 4.
*
* @param numBases
*/
public void pitchWithHit(int numBases) {
}
/**
* Pitch resulting in a hit and a possible out.
*/
public void pitchWithHitAndOut(int numBases, int whichBaseFielded) {
}
/**
* Returns whether there is a player on first base.
*
* @return
*/
public boolean playerOnFirst() {
return true;
}
/**
* Returns whether there is a player on second base.
*
* @return
*/
public boolean playerOnSecond() {
return true;
}
/**
* Returns whether there is a player on third base.
*
* @return
*/
public boolean playerOnThird() {
return true;
}
/**
* Returns a multi-line string representation of the current game state. The
* format is: o - o Inning:1 (T) | | Score:0-0 o - H Balls:0 Strikes:0 Outs:0
*
* The 'T' after the inning number indicates it's the top of the inning, and a
* 'B' would indicate the bottom.
*
* @return
*/
public java.lang.String toDisplayString() {
return null;
}
/**
* Returns a one-line string representation of the current game state. The
* format is: ooo Inning:1 (T) Score:0-0 Balls:0 Strikes:0 Outs:0
*
* The first three characters represent the players on base as returned by the
* getBases() method. The 'T' after the inning number indicates it's the top of
* the inning, and a 'B' would indicate the bottom.
*/
public java.lang.String toString() {
return null;
}
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
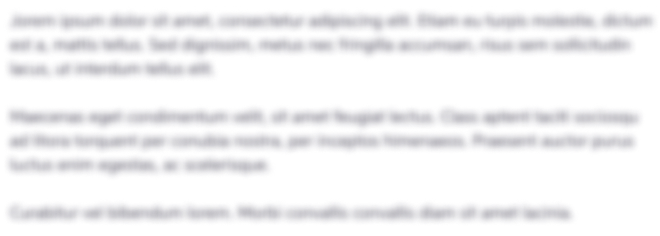
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started