Question
4.15 Project 3 Project Outcomes: Read user input and validate the value uses branch control structure uses loop control structure follows standard acceptable programming practices.
4.15 Project 3
Project Outcomes:
Read user input and validate the value
uses branch control structure
uses loop control structure
follows standard acceptable programming practices.
uses javadoc to for the class
Prep Readings:
Chapter 1 - 4
Project Requirements:
Write a Java program named InterestCalculator.java that calculates the value of a bank account based on starting principle, interest rate and time.
The program prompt the user for
Number of quarters to display (int) that is greater than zero and less or equal to 10.
Starting balance (double) that is greater than zero.
Interest rate (double) that is greater than zero and less than or equal to 20%
Display the information the user entered and ask if it is correct. If so continue, if not ask for all the information again (loop and half example in Addition Iteration examples).
Calculate the ending balance for each quarter and display the results in a table similar to below, the formula for quarterly interest is balance * interestRate / 100 * .25 where balance is balance from last quarter (current balance) and interestRate is annual interest rate from user input. The new balance will be the old balance plus the calculated quarterly interest.
If the user enters a value outside the boundaries listed above, prompt them with an error message and ask for the value again. Please find the corresponding error messages in sample run 2.
Sample run 1: input/output with all correct inputs
Enter number of quarters from 1 to 10: 8 Enter the beginning principal balance greater than 0: 1000 Enter the interest rate percentage without the percent sign greater than 0% and less than/equal to 20%: 5.25 You entered a principal balance of $1000.00 for 8 quarters at 5.25% interest. Is this correct? (y/n) y Quarter Beginning Interest Ending Number Balance Earned Balance 1 $1000.00 $13.13 $1013.13 2 $1013.13 $13.30 $1026.42 3 $1026.42 $13.47 $1039.89 4 $1039.89 $13.65 $1053.54 5 $1053.54 $13.83 $1067.37 6 $1067.37 $14.01 $1081.38 7 $1081.38 $14.19 $1095.57 8 $1095.57 $14.38 $1109.95
Sample run 2: input/output with some bad inputs
Enter number of quarters from 1 to 10: 12 Number of quarters must be between 1 and 10 inclusive. Enter number of quarters from 1 to 10: 8 Enter the beginning principal balance greater than 0: -200 Beginning balance must be greater than zero. Enter the beginning principal balance greater than 0: 1000 Enter the interest rate percentage without the percent sign greater than 0% and less than/equal to 20%: 25 Interest rate must be between 1 and 20 inclusive. Enter the interest rate percentage without the percent sign greater than 0% and less than/equal to 20%: 5.25 You entered a principal balance of $1000.00 for 8 quarters at 5.25% interest. Is this correct? (y/n) n Enter number of quarters from 1 to 10: 8 Enter the beginning principal balance greater than 0: 10000 Enter the interest rate percentage without the percent sign greater than 0% and less than/equal to 20%: 5.25 You entered a principal balance of $10000.00 for 8 quarters at 5.25% interest. Is this correct? (y/n) Y Quarter Beginning Interest Ending Number Balance Earned Balance 1 $10000.00 $131.25 $10131.25 2 $10131.25 $132.97 $10264.22 3 $10264.22 $134.72 $10398.94 4 $10398.94 $136.49 $10535.43 5 $10535.43 $138.28 $10673.70 6 $10673.70 $140.09 $10813.80 7 $10813.80 $141.93 $10955.73 8 $10955.73 $143.79 $11099.52
Both samples are from either jGrasp or command line execution of the program. You will not see user input when you run this code in zybook.
Other instruction
Copy the user prompt and error message from the sample run to avoid output format mismatch.
Use printf to print table rows with data. Display to the hundreds for all money amount.
Develop this program on your PC and upload the InterestCalculator.java file to the project 3 dropbox on elearning.
Your program should be compilable and giving generally correct input/output on your PC before you test run your code on this page.
You will get 80% if you pass all tests on this page. If you can not pass all tests here, there will be an automatic 20% off before your code is manually graded on the .java file uploaded to elearning. There will be another 20% off if your code is not compilable.
The rest of the grade (20%) will be graded on elearning on your coding style and javadoc.
Information for running on your code on this page
input to be used in develop mode for sample run 1:
8 1000 5.25 y
Output you will see in develop mode with above input:
Enter number of quarters from 1 to 10: Enter the beginning principal balance greater than 0: Enter the interest rate percentage without the percent sign greater than 0% and less than/equal to 20%: You entered a principal balance of $1000.00 for 8 quarters at 5.25% interest. Is this correct? (y/n) Quarter Beginning Interest Ending Number Balance Earned Balance 1 $1000.00 $13.13 $1013.13 2 $1013.13 $13.30 $1026.42 3 $1026.42 $13.47 $1039.89 4 $1039.89 $13.65 $1053.54 5 $1053.54 $13.83 $1067.37 6 $1067.37 $14.01 $1081.38 7 $1081.38 $14.19 $1095.57 8 $1095.57 $14.38 $1109.95
input to be used in develop mode for sample run 2:
12 8 -200 1000 25 5.25 n 8 10000 5.25 Y
Output you will see in develop mode with above input:
Enter number of quarters from 1 to 10: Number of quarters must be between 1 and 10 inclusive. Enter number of quarters from 1 to 10: Enter the beginning principal balance greater than 0: Beginning balance must be greater than zero. Enter the beginning principal balance greater than 0: Enter the interest rate percentage without the percent sign greater than 0% and less than/equal to 20%: Interest rate must be between 1 and 20 inclusive. Enter the interest rate percentage without the percent sign greater than 0% and less than/equal to 20%: You entered a principal balance of $1000.00 for 8 quarters at 5.25% interest. Is this correct? (y/n) Enter number of quarters from 1 to 10: Enter the beginning principal balance greater than 0: Enter the interest rate percentage without the percent sign greater than 0% and less than/equal to 20%: You entered a principal balance of $10000.00 for 8 quarters at 5.25% interest. Is this correct? (y/n) Quarter Beginning Interest Ending Number Balance Earned Balance 1 $10000.00 $131.25 $10131.25 2 $10131.25 $132.97 $10264.22 3 $10264.22 $134.72 $10398.94 4 $10398.94 $136.49 $10535.43 5 $10535.43 $138.28 $10673.70 6 $10673.70 $140.09 $10813.80 7 $10813.80 $141.93 $10955.73 8 $10955.73 $143.79 $11099.52
Step by Step Solution
There are 3 Steps involved in it
Step: 1
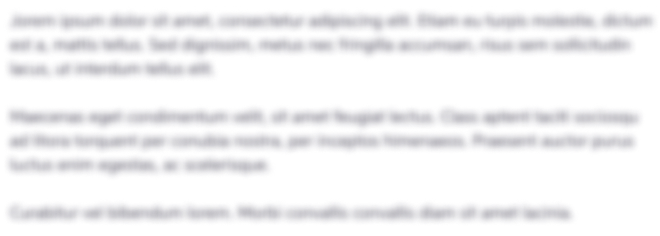
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started