Question
4.5 Roster Stores the student information in an ArrayList list. Read the comments and implement the pseudocode. Please complete the code. /** * The Roster
4.5 Roster
Stores the student information in an ArrayList list. Read the comments and implement the pseudocode.
Please complete the code.
/**
* The Roster class encapsulates an ArrayList which stores the information for each student in the gradebook.
*/
public class Roster {
// Declare mStudentList
???
/**
* Roster()
*
* PSEUDOCODE:
* Create mStudentList.
*/
???
/**
* addStudent()
*
* PSEUDOCODE:
* Add (append) pStudent to mStudentList.
*/
???
/**
* getStudent()
* Searches mStudentList for a Student with pLastName.
*
* PSEUDOCODE:
* index = Call Searcher.search(getStudentList(), pLastName)
* If index == -1 Then Return null
* Else return the Student object in mStudentList at index
*/
???
/**
* getStudentList()
* Accessor method for mStudentList.
*/
public ArrayList getStudentList() {
return mStudentList;
}
/**
* setStudentList()
* Mutator method for mStudentList.
*/
public void setStudentList(ArrayList pStudentList) {
mStudentList = pStudentList;
}
/**
* sortRoster()
* Called to sort the roster by last name.
*
* PSEUDOCODE:
* Call Sorter.sort() passing the list of students
*/
???
/**
* Returns a String representation of this Roster. Handy for debugging.
*/
@Override
public String toString() {
String result = "";
for (Student student : getStudentList()) result += student + " ";
return result;
}
}
Here is the rest of the code,
Main.java
import java.io.File; import java.io.FileNotFoundException; import javax.swing.JFrame;
/** * The Main class containing the main() and run() methods. */ public class Main {
// The Roster of students that is read from "gradebook.txt". private Roster mRoster;
// A reference to the View object. private View mView;
/** * This is where execution starts. Instantiate a Main object and then call * run(). */ public static void main(String[] pArgs) { Main main = new Main(); main.run(); }
public void exit() { try { GradebookWriter writer = new GradebookWriter("gradebook.txt"); writer.writeGradebook(getRoster()); System.exit(0); } catch (FileNotFoundException pExcept) { getView().messageBox("Could not open gradebook.txt for writing. " + "Exiting without saving."); System.exit(-1); } }
/** * Accessor method for mRoster. */ public Roster getRoster() { return mRoster; }
/** * Accessor method for mView. */ public View getView() { return mView; } private void run() { JFrame.setDefaultLookAndFeelDecorated(true);
setView(new View(this));
try { GradebookReader reader = new GradebookReader("gradebook.txt"); setRoster(reader.readGradebook());
} catch (FileNotFoundException pExcept) { getView().messageBox("Could not open gradebook.txt for reading. Exiting."); System.exit(-1); }
}
/** * search() is called when the Search button is clicked on the View. The input parameter is the non-empty last name * of the Student to locate. Call getStudent(pLastName) on the Roster object to get a reference to the Student with * that last name. If the student is not located, getStudent() returns null. */ public Student search(String pLastName) { return getRoster().getStudent(pLastName); }
/** * Mutator method for mRoster. */ public void setRoster(Roster pRoster) { mRoster = pRoster; }
/** * Mutator method for mView. */ public void setView(View pView) { mView = pView; } }
Student.java
import java.util.ArrayList;
/** * The Student class stores the grade information for one Student. */ public class Student implements Comparable
// Declare the instance variables. private String mFirstName; private String mLastName; private ArrayList
/** * Student() * * PSEUDOCODE: * Save pFirstName and pLastName. * Create mexam1List * Create mHomeworkList */ public Student(String pFirstName, String pLastName) { mFirstName = pFirstName; mLastName = pLastName; mexam1List = new ArrayList<>(); mHomeworkList = new ArrayList<>(); }
/** * addexam1() * * PSEUDOCODE: * Call add(pScore) on getexam1List() to add a new exam1 score to the list of exam1 scores. */ public void addexam1(int pScore) { getexam1List().add(pScore); }
/** * addHomework() * * PSEUDOCODE: * Call add(pScore) on getHomeworkList() to add a new homework score to the list of homework scores. */ public void addHomework(int pScore) { getHomeworkList().add(pScore); }
/** * compareTo() * * PSEUDOCODE: * Return: -1 if the last name of this Student is < the last name of pStudent * Return: 0 if the last name of this Student is = the last name of pStudent * Return: 1 if the last name of this Student is > the last name of pStudent * Hint: the last names are Strings. */ @Override public int compareTo(Student pStudent) { int result = this.getLastName().compareTo(pStudent.getLastName());
if (result > 0) { result = 1; } else if (result < 0) { result = -1; } return result; }
/** * getexam1() * * Accessor method to retreive an exam1 score from the list of exam1s. */ public int getexam1(int pNum) { return getexam1List().get(pNum); }
/** * getexam1List() * * Accessor method for mexam1List. */ protected ArrayList
/** * getFirstName() * * Accessor method for mFirstName. */ public String getFirstName() { return mFirstName; }
/** * getHomework() * * Accessor method to retrieve a homework score from the list of homeworks. */ public int getHomework(int pNum) { return getHomeworkList().get(pNum); }
/** * getHomeworkList() * * Accessor method for mHomeworkList. */ protected ArrayList
/** * getLastname() * * Accessor method for mLastName. */ public String getLastName() { return mLastName; }
/** * setexam1() * * Mutator method to store an exam1 score into the list of exam1 scores. */ public void setexam1(int pNum, int pScore) { getexam1List().set(pNum, pScore); }
/** * setexam1List() * * Mutator method for mexam1List. */ protected void setexam1List(ArrayList
/** * setFirstName() * * Mutator method for mFirstName. */ public void setFirstName(String pFirstName) { mFirstName = pFirstName; }
/** * setHomework() * * Mutator method to store a homework score into the list of homework * scores. */ public void setHomework(int pNum, int pScore) { getHomeworkList().set(pNum, pScore); }
/** * setHomeworkList() * * Mutator method for mHomeworkList. */ protected void setHomeworkList(ArrayList
/** * setLastname() * * Mutator method for mLastName. */ public void setLastName(String pLastName) { mLastName = pLastName; }
/** * toString() * * Returns a String representation of this Student. The format of the * returned string shall be such that the Student information can be printed * to the output file, i.e: * * lastname firstname hw1 hw2 hw3 hw4 exam11 exam12 */ @Override public String toString() { String result = getLastName() + " " + getFirstName() + " "; for (Integer grade : getHomeworkList()) { result = result + grade.toString() + " "; } for (Integer grade : getexam1List()) { result = result + grade.toString() + " "; } result = result.substring(0, result.length() - 1);
return result; } }
View.java
import java.awt.event.ActionEvent; import java.awt.event.ActionListener; import javax.swing.BoxLayout; import javax.swing.JButton; import javax.swing.JFrame; import javax.swing.JLabel; import javax.swing.JOptionPane; import javax.swing.JPanel; import javax.swing.JTextField;
/** * The View class implements the GUI. */ public class View extends JFrame implements ActionListener {
public static final int FRAME_WIDTH = 500; public static final int FRAME_HEIGHT = 250;
// Declare instance variables private JButton mClearButton; private JTextField[] mexam1Text = new JTextField[CourseConstants.NUM_exam1S]; private JButton mExitButton; private JTextField[] mHomeworkText = new JTextField[CourseConstants.NUM_HOMEWORKS]; private Main mMain; private JButton mSaveButton; private JButton mSearchButton; private JTextField mSearchText; private Student mStudent;
/** * View() * * The View constructor creates the GUI interface and makes the frame * visible at the end. */ public View(Main pMain) {
// Save a reference to the Main object pMain in mMain. mMain = pMain;
JPanel panelSearch = new JPanel(); mSearchText = new JTextField(25); panelSearch.add(mSearchText); mSearchButton = new JButton("Search"); mSearchButton.addActionListener(this); panelSearch.add(mSearchButton);
// PSEUDOCODE: // Create a JPanel named panelHomework which uses the FlowLayout. // Add a JLabel "Homework: " to the panel // Create mHomeworkText which is an array of CourseConstants.NUM_HOMEWORKS JTextFields // For i = 0 to CourseConstants.NUM_HOMEWORKS - 1 Do // Create textfield mHomeworkText[i] displaying 5 cols // Add mHomeworkText[i] to the panel // End For JPanel panelHomework = new JPanel(); panelHomework.add(new JLabel("Homework:")); for (int x = 0; x < CourseConstants.NUM_HOMEWORKS; ++x) { mHomeworkText[x] = new JTextField(5); panelHomework.add(mHomeworkText[x]); }
// Create the exam1 panel which contains the "exam1: " label and the two exam1 text fields. The pseudocode is omitted // because this code is very similar to the code that creates the panelHomework panel. JPanel panelexam1 = new JPanel(); panelexam1.add(new JLabel("exam1:")); for (int x = 0; x < CourseConstants.NUM_exam1S; ++x) { mexam1Text[x] = new JTextField(5); panelexam1.add(mexam1Text[x]); }
JPanel panelButtons = new JPanel(); mClearButton = new JButton("Clear"); mClearButton.addActionListener(this); panelButtons.add(mClearButton); mSaveButton = new JButton("Save"); mSaveButton.addActionListener(this); panelButtons.add(mSaveButton); mExitButton = new JButton("Exit"); mExitButton.addActionListener(this); panelButtons.add(mExitButton);
// PSEUDOCODE: // Create a JPanel named panelMain using a vertical BoxLayout. // Add panelSearch to panelMain. // Add panelHomework to panelMain. // Add panelexam1 to panelMain. // Add panelButtons to panelMain. JPanel panelMain = new JPanel(); panelMain.setLayout(new BoxLayout(panelMain, BoxLayout.Y_AXIS)); panelMain.add(panelSearch); panelMain.add(panelHomework); panelMain.add(panelexam1); panelMain.add(panelButtons);
// Initialize the remainder of the frame, add the main panel to the frame, and make the frame visible. setTitle("Gradebook Viewer"); setSize(FRAME_WIDTH, FRAME_HEIGHT); setResizable(false); setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); add(panelMain); setVisible(true); }
@Override public void actionPerformed(ActionEvent pEvent) {
switch (pEvent.getActionCommand()) { case "Search": String lastName = mSearchText.getText(); if (lastName.isEmpty()) { this.messageBox("Please enter the student's last name."); } else { Student student = mMain.search(lastName); if (student == null) { this.messageBox("Student not found. Try again."); } else { mStudent = student; displayStudent(student); } } break; case "Save": if (mStudent != null) { saveStudent(mStudent); } break; case "Clear": clear(); break; case "Exit": if (mStudent != null) { saveStudent(mStudent); } mMain.exit(); break; } }
/** * clear() * * Called when the Clear button is clicked. Clears all of the text fields by setting the contents to the empty string. * After clear() returns, no student information is being edited or displayed. * * PSEUDOCODE: * Set the mSearchText text field to "" * Set each of the homework text fields to "" * Set each of the exam1 text fields to "" * Set the mStudent reference to null */ private void clear() { mSearchText.setText(""); for (int x = 0; x < CourseConstants.NUM_HOMEWORKS; ++x) { mHomeworkText[x].setText(""); } for (int x = 0; x < CourseConstants.NUM_exam1S; ++x) { mexam1Text[x].setText(""); } mStudent = null; }
/** * displayStudent() * * Displays the homework and exam1 scores for a student in the mHomeworkText and mexam1Text text fields. * * PSEUDOCODE: * For i = 0 to CourseConstants.NUM_HOMEWORKS - 1 Do * int hw = pStudent.getHomework(i) * String hwstr = convert hw to a String (Hint: Integer.toString()) * mHomeworkText[i].setText(hwstr) * End For * Write another for loop similar to the one above to place the exam1s scores into the text fields */ private void displayStudent(Student pStudent) { for (int x = 0; x < CourseConstants.NUM_HOMEWORKS; ++x) { Integer hw = pStudent.getHomework(x); String hwStr = hw.toString(); mHomeworkText[x].setText(hwStr); } for (int x = 0; x < CourseConstants.NUM_exam1S; ++x) { Integer exam1 = pStudent.getexam1(x); String exam1Str = exam1.toString(); mexam1Text[x].setText(exam1Str); } }
/** * messageBox() * * Displays a message box containing some text. */ public void messageBox(String pMessage) { JOptionPane.showMessageDialog(this, pMessage, "Message", JOptionPane.PLAIN_MESSAGE); }
/** * saveStudent() * * Retrieves the homework and exam1 scores for pStudent from the text fields and writes the results to the Student record * in the Roster. * * PSEUDOCODE: * For i = 0 to CourseConstants.NUM_HOMEWORKS - 1 Do * String hwstr = mHomeworkText[i].getText() * int hw = convert hwstr to an int (Hint: Integer.parseInt()) * Call pStudent.setHomework(i, hw) * End For * Write another for loop similar to the one above to save the exam1 scores */ private void saveStudent(Student pStudent) {
for (int x = 0; x < CourseConstants.NUM_HOMEWORKS; ++x) { String hwStr = mHomeworkText[x].getText(); Integer hw = Integer.parseInt(hwStr); pStudent.setHomework(x, hw); } for (int x = 0; x < CourseConstants.NUM_exam1S; ++x) { String exam1Str = mexam1Text[x].getText(); Integer exam1 = Integer.parseInt(exam1Str); pStudent.setexam1(x, exam1); }
int studentIdx = Searcher.search(mMain.getRoster().getStudentList(), pStudent.getLastName());
mMain.getRoster().getStudentList().set(studentIdx, pStudent);
}
}
CourseConstants.java public class CourseConstants {
public static final int NUM_exam1S = 2; public static final int NUM_HOMEWORKS = 4;
}
GradebookReader.java
import java.io.File; import java.io.FileNotFoundException; import java.util.Scanner;
/** * GradebookReader reads the gradebook info from the file name passed to the ctor. */ public class GradebookReader {
private Scanner mIn;
/** * Attempts to open the gradebook file for reading. If successful, mIn will be used to read from the file. If the file * cannot be opened, a FileNotFoundException will be thrown. */ public GradebookReader(String pFname) throws FileNotFoundException { mIn = new Scanner(new File(pFname)); }
/** * Reads the exam1 scores for a Student. */ private void readexam1(Student pStudent) { for (int n = 0; n < CourseConstants.NUM_exam1S; ++n) { pStudent.addexam1(mIn.nextInt()); } }
/** * Called to read the gradebook information. Calls readRoster() to read the student records and then sorts the roster * by last name. */ public Roster readGradebook() { Roster roster = readRoster(); roster.sortRoster(); return roster; }
/** * Reads the homework scores for a Student. */ private void readHomework(Student pStudent) { for (int n = 0; n < CourseConstants.NUM_HOMEWORKS; ++n) { pStudent.addHomework(mIn.nextInt()); } }
/** * Reads the student information from the input file adding Student objecs to the roster. */ private Roster readRoster() { Roster roster = new Roster(); while (mIn.hasNext()) { String lastName = mIn.next(); String firstName = mIn.next(); Student student = new Student(firstName, lastName); readHomework(student); readexam1(student); roster.addStudent(student); } return roster; } }
Searcher.java
import java.util.ArrayList;
public class Searcher {
public static int search(ArrayList
// recursive search used to find the student by last name private static int recBinarySearch(ArrayList
int result = pList.get(middle).getLastName().compareTo(pKey);
if (result < 0) { // value is less than key, recurse with pLow = middle + 1 return recBinarySearch(pList, pKey, middle + 1, pHigh); } else if (result > 0) { // value is greater than key, recurse with pHigh = middle - 1 return recBinarySearch(pList, pKey, pLow, middle - 1); } else { // value matches with key; return 'middle' return middle; } }
}
GradebookWriter.java
import java.io.File; import java.io.FileNotFoundException; import java.io.PrintWriter;
/** * GradebookWriter inherits from PrintWriter and writes the gradebook info to the file name passed to the ctor. */ public class GradebookWriter extends PrintWriter {
private PrintWriter mOut;
/** * GradebookWriter() * Call the super class ctor that takes a String. */ public GradebookWriter(String pFname) throws FileNotFoundException { super(new File(pFname)); mOut = this; }
/** * writeGradebook() * Writes the gradebook info to the file, which was opened in the ctor. * * PSEUDOCODE: * EnhancedFor each student in pRoster.getStudentList() Do * Call println(student) * End For * Call close() */ public void writeGradebook(Roster pRoster) {
for (Student student : pRoster.getStudentList()) { mOut.println(student); } mOut.close(); } }
Sorter.java import java.util.ArrayList;
public class Sorter {
// Partition the list into 'two' lists (within the same list) private static int partition(ArrayList
int leftIndex = pFromIdx - 1; int rightIndex = pToIdx + 1;
while (leftIndex < rightIndex) { leftIndex++; while (pList.get(leftIndex).compareTo(pivot) == -1) { leftIndex++; } rightIndex--; while (pList.get(rightIndex).compareTo(pivot) == 1) { rightIndex--; } if (leftIndex < rightIndex) { swap(pList, leftIndex, rightIndex); } } return rightIndex; }
// Implementation of the quickSort method from notes private static void quickSort(ArrayList
// Entry point for the Sorter public static void sort(ArrayList
quickSort(pList, 0, pList.size() - 1);
}
// Method to swap two elements within the list private static void swap(ArrayList
}
gradebook.txt
Simpson Lisa 25 25 25 25 100 100 Flintstone Fred 15 17 22 18 80 60 Jetson George 20 21 22 23 70 83 Explosion Nathan 5 4 3 2 1 0 Muntz Nelson 20 15 10 5 60 70 Terwilliger Robert 23 21 19 17 80 90 Flanders Ned 12 14 17 23 85 95 Bouvier Selma 16 16 16 16 16 16 Spuckler Cletus 1 2 3 4 5 6 Wiggum Clancy 6 5 4 3 2 1 Skinner Seymour 19 23 21 24 78 83
Step by Step Solution
There are 3 Steps involved in it
Step: 1
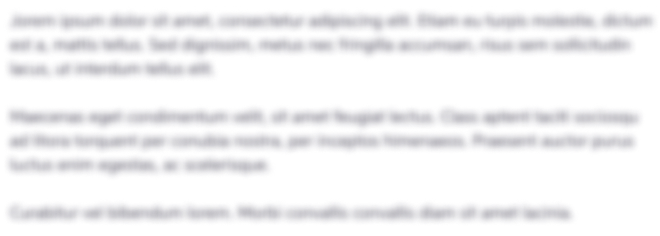
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started