Question
6.22 Lab Lesson 9 (Part 1 of 2) Part of lab lesson 9 There are two parts to lab lesson 9. The entire lab will
6.22 Lab Lesson 9 (Part 1 of 2)
Part of lab lesson 9
There are two parts to lab lesson 9. The entire lab will be worth 100 points.
Bonus points for lab lesson 9
There are also 10 bonus points. To earn the bonus points you have to complete the Participation Activities and Challenge Activities for zyBooks/zyLabs unit 6. These have to be completed by the due date for lab lesson 9. For example, if you complete 89% of the activities you will get 8 points (there is no rounding).
Lab lesson 9 part 1 is worth 50 points
For part 1 you will have 40 points if you enter the program and successfully run the program tests. An additional 10 points will be based on the style and formatting of your C++ code.
Style points
The 10 points for coding style will be based on the following guidelines:
Comments at the start of your programming with a brief description of the purpose of the program.
Comments throughout your program
Proper formatting of your code (follow the guidelines in the Gaddis text book, or those used by your CS 1336 professor)
If you have any variables they must have meaningful names.
Development in your IDE
For lab lesson 9 (both parts) you will be developing your solutions using an Integrated Development Environment (IDE) such as Visual Studio, Code::Blocks or Eclipse. You should use whatever IDE you are using for your CS 1336 class. Once you have created and tested your solutions you will be uploading the files to zyBooks/zyLabs. Your uploaded file must match the name specified in the directions for the lab lesson. You will be using an IDE and uploading the appropriate files for this and all future lab lessons.
You will need to develop and test the program in your IDE. Once you are satisfied that it is correct you will need to upload the source file to zyBooks/zyLabs, and submit it for the Submit mode tests. If your program does not pass all of the tests you need to go back to the IDE, and update your program to fix the problems you have with the tests. You must then upload the program from the IDE to zyBooks/zylabs again. You can then run the tests again in Submit mode.
When running your program in Submit mode it is very important that you look at the output from all of the tests. You should then try and fix all of the problems in your IDE and then upload the updated code to zyBooks/zyLabs.
C++ requirements
The number of spools ordered, the number of spools currently in stock both are of type int.
The shipping and handling charge is of type double.
The calculations for subtotal, shipping and handling charges, and the total need to be done using type double.
You must have at least three functions. One must be the read function, one is your main function and one is your display function. Your main function will be calling the read and display functions as needed. The read function must NOT call the display function. The display function must NOT call the read function.
You must use function prototypes for all of the functions except main.
The values passed to the read function must be passed by reference so they can be updated by the read function.
The use of non const global variables is not permitted and will result in a loss of, at least, 25 points.
Failure to follow the C++ requirements could reduce the points received from passing the tests.
General overview
This lab lesson you are writing a program for the hypothetical Acme Wholesale Copper Wire Company. The Acme company sells spools of copper wiring for $100 each. Write a program that displays the status of an order.
This program will be reading in from cin and writing to cout.
In this program you will need at least three functions, including main as one of the three.
You must use function prototypes for all of the functions (except main).
Read function
You need to have a function that reads in the following data:
The number of spools ordered
The number of spools currently in stock
Any special shipping and handling charges (see description below).
Your program needs to prompt for these values. The prompts are below under Sample with special shipping and handling.
Do not accept a number less than 1 for the number of spools ordered.
Do not accept a number less than 0 for the number of spools in stock.
Do not accept a shipping and handling charge of less than 0.
For each of these cases you will have to have a loop that issues an error message, prompts for the input value again, and checks the data for validity.
The normal shipping and handling is $11.88 per spool. You will have to ask if there is special shipping and handling. If there is you will need to read in the special shipping and handling charge.
Here are a couple of sample runs. One with the default shipping and handling and one with special shipping and handling.
Sample with default shipping:
Assume the following input from cin:
10 100 n
Here are the prompts to read in the spools to be ordered, spools in stock and shipping and handling:
Spools to be ordered Spools in stock Special shipping and handling (y or n)
Sample with special shipping and handling:
Assume the following input from cin:
10 100 y 9.99
Here is the output or the prompts to cout:
Spools to be ordered Spools in stock Special shipping and handling (y or n) Shipping and handling amount
Note that the Shipping and handling amount prompt is only written out if the input from cin was y from the Special shipping and handling (y or n) prompt.
If the input from the Shipping and handling amount is any value other than y (lower case y) you can assume the default shipping and handling amount. There is not validity checking for this value other than y and any other value (meaning no special shipping and handling).
The read function needs to return three values:
spools to be ordered
spools in stock
shipping and handling (either the default value or one specified by the user).
Since a function can only return one value we cannot return the values via the return statement. Instead you need to pass three variables to the read function and pass them by reference. That way the read function can update the actual variables passed to the read function. You not NOT use any global variables.
error messages
Here are the error messages that the read function may generate:
Spools order must be 1 or more Spools in stock must be 0 or more The spool shipping and handling charge must be 0.0 or more
The above processing is all done in the read function.
The values you read into (for the spools ordered, spools in stock and the shipping and handling charge) all need to be passed to the read function by reference. This is needed because the read function will be updating all three variables and it needs access to the variables.
The returned shipping charge will either be the default charge of $11.88 per spool or the special shipping and handling charge (if requested).
Display function
You will also need a display function that takes the spools ordered, spools in stock and the shipping and handling charges. These values will not be changed by the function, so these can all be passed by value.
The display function needs to display:
the number of spools (ordered) that are ready to ship from the current stock
the number of spools on back-order (if the numbered ordered is greater than what is in stock)
the subtotal of the spools ready to ship (the number ready to ship times $100)
the total shipping and handling charges for the spools ready to ship
the total of the order ready to ship
The display function will output these values to cout.
Assume the number of spools ordered is 9 and there are 5 spools in stock. Assume the shipping cost is $10. The output would be as follows. All of the amounts should have two digits to the right of the decimal point and a leading $ character. The total width of the number (not including the $ character) is 10 characters.
Spools to be ordered Spools in stock Special shipping and handling (y or n) Shipping and handling amount Spools ready to ship: 5 Spools on back-order: 4 Subtotal ready to ship: $ 500.00 Shipping and handling: $ 50.00 Total shipping charges: $ 550.00
The main function
The main function is fairly simple. You need to call the read function and then pass the updated values to the display function.
Here are some sample runs:
Sample run # 1 (valid input with special handling):
Assume the following is read in from cin
9 5 y 10.0
The contents of cout:
Spools to be ordered Spools in stock Special shipping and handling (y or n) Shipping and handling amount Spools ready to ship: 5 Spools on back-order: 4 Subtotal ready to ship: $ 500.00 Shipping and handling: $ 50.00 Total shipping charges: $ 550.00
Sample run # 2 (valid input, no special handling)
The following is read in from cin
35 35 n
The following would be written to cout:
Spools to be ordered Spools in stock Special shipping and handling (y or n) Spools ready to ship: 35 Spools on back-order: 0 Subtotal ready to ship: $ 3500.00 Shipping and handling: $ 415.80 Total shipping charges: $ 3915.80
Sample run # 3 (invalid input)
Assume the following is read in from cin
0 200 -1 12 y -0.01 5.99
The contents of cout after the run:
Spools to be ordered Spools order must be 1 or more Spools to be ordered Spools in stock Spools in stock must be 0 or more Spools in stock Special shipping and handling (y or n) Shipping and handling amount The spool shipping and handling charge must be 0.0 or more Shipping and handling amount Spools ready to ship: 12 Spools on back-order: 188 Subtotal ready to ship: $ 1200.00 Shipping and handling: $ 71.88 Total shipping charges: $ 1271.88
Failure to follow the requirements for lab lessons can result in deductions to your points, even if you pass the validation tests. Logic errors, where you are not actually implementing the correct behavior, can result in reductions even if the test cases happen to return valid answers. This will be true for this and all future lab lessons.
Expected output
There are six tests. Each test will have a new set of input data. You must match, exactly, the expected output.
You will get yellow highlighted text when you run the tests if your output is not what is expected. This can be because you are not getting the correct result. It could also be because your formatting does not match what is required. The checking that zyBooks does is very exacting and you must match it exactly. More information about what the yellow highlighting means can be found in course "How to use zyBooks" - especially section "1.4 zyLab basics".
Finally, do not include a system("pause"); statement in your program. This will cause your verification steps to fail.
Note: that the system("pause"); command runs the pause command on the computer where the program is running. The pausecommand is a Windows command. Your program will be run on a server in the cloud. The cloud server may be running a different operating system (such as Linux).
Error message "Could not find main function"
Now that we are using functions some of the tests are unit tests. In the unit tests the zyBooks environment will call one or more of your functions directly.
To do this it has to find your main function.
Right now zyBooks has a problem with this when your int main() statement has a comment on it.
For example:
If your main looks as follows:
int main() // main function
You will get an error message:
Could not find main function
You need to change your code to:
// main function int main()
If you do not make this change you will continue to fail the unit tests.
g++ lesson9part1.cpp -Wall -Wextra -Wuninitialized -pedantic-errors -Wconversion -o a.out
We will use this command to compile your code
Step by Step Solution
There are 3 Steps involved in it
Step: 1
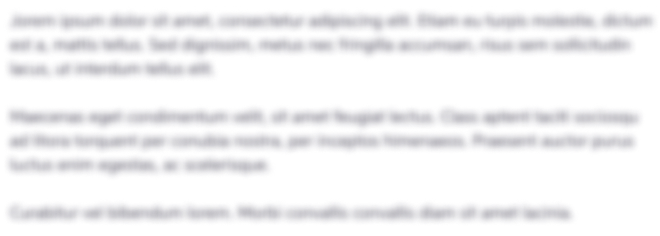
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started