Question
63. Change our trivia game playing system so that it keeps track, and reports to the user in an appropriate fashion, how many times the
63. Change our trivia game playing system so that it keeps track, and reports to the user in an appropriate fashion, how many times the user attempts to answer each of the various questions.
public class TriviaQuestion { private String category; // category of question private String question; // the question private StringLogInterface answers; // acceptable answers
public TriviaQuestion(String category, String question, int maxNumAnswers) // Precondition: maxNumAnswers > 0 { this.category = category; this.question = question; answers = new ArrayStringLog("trivia", maxNumAnswers); }
public String getCategory() { return category; }
public String getQuestion() { return question; }
public boolean tryAnswer(String answer) { return answers.contains(answer); }
public void storeAnswer(String answer) // Precondition: answers String Log is not full { answers.insert(answer); } }
............
public class TriviaGame { private String quizName; private int maxNumQuestions; private int numChances; private int remainingChances; private int numCorrect = 0; private int numIncorrect = 0; private TriviaQuestion[] questions; // the set of questions private boolean[] correct; // true if corresponding question answered private int currNumQuestions = 0; // current number of questions private int[] attempt; // how many attempt been made per question
public TriviaGame(String quizName, int maxNumQuestions, int numChances) // Precondition: maxNumQuestions > 0 and numChances > 0 { this.quizName = quizName; this.maxNumQuestions = maxNumQuestions; this.numChances = numChances; remainingChances = numChances; questions = new TriviaQuestion[maxNumQuestions]; correct = new boolean[maxNumQuestions]; attempt = new int[maxNumQuestions]; }
public String getQuizName() { return quizName; }
public int getNumChances() { return numChances; }
public int getRemainingChances() { return remainingChances; }
public int getNumCorrect() { return numCorrect; }
public int getNumIncorrect() { return numIncorrect; }
public int getCurrNumQuestions() { return currNumQuestions; }
public TriviaQuestion getTriviaQuestion(int questionNumber) // Precondition: 0 < questionNumber <= currNumQuestions { return questions[questionNumber - 1]; }
public boolean isAnswered(int questionNumber) // Precondition: 0 < questionNumber <= currNumQuestions { return correct[questionNumber - 1]; }
public boolean isOver() // Returns true if this game is over, false otherwise. { return (numCorrect == currNumQuestions) || (remainingChances <= 0); }
public void insertQuestion(TriviaQuestion question) // Precondition: currNumQuestions < maxNumQuestions // // Adds question to this TriviaGame. { questions[currNumQuestions] = question; correct[currNumQuestions] = false; attempt[currNumQuestions] = 0; currNumQuestions = currNumQuestions + 1; }
public void correctAnswer(int questionNumber) // Preconditions: 0 < questionNumber < maxNumQuestions // // Updates game status to indicate that question number // "questionNumber" was answered correctly. { correct[questionNumber - 1] = true; numCorrect = numCorrect + 1; remainingChances = remainingChances - 1; }
public void incorrectAnswer() // Updates game status to indicate that a question // was answered incorrectly { numIncorrect = numIncorrect + 1; remainingChances = remainingChances - 1; }
public void recordAttempt(int questionNumber) // Precondition: 0 < questionNumber <= currNumQuestions { attempt[questionNumber - 1]++; }
public void displayAttempts() { // Output the number of attempt for each question int i; for(i = 0 ; i < attempt.length ; i++) System.out.println(" You attempted question " + (i + 1) + ", " + attempt[i] + " times."); } }
................
import java.util.*; import java.io.*;
public class GetTriviaGame { public static TriviaGame useTextFile(String textfile)throws IOException // Precondition: The textfile exists and contains a correctly formatted game. { TriviaGame game;
String quizName; int numQuestions; int numChances;
// for a specific trivia question TriviaQuestion tq; String category; String question; String answer; int numAnswers; FileReader fin = new FileReader(textfile); Scanner triviaIn = new Scanner(fin); String skip; // skip end of line after reading integer // Scan in basic trivia quiz information and set variables. quizName = triviaIn.nextLine(); numQuestions = triviaIn.nextInt(); numChances = triviaIn.nextInt(); skip = triviaIn.nextLine();
// Instantiate the Trivia Game. game = new TriviaGame(quizName, numQuestions, numChances);
// Scan in and set up the questions and answers. for (int i = 1; i <= numQuestions; i++) { category = triviaIn.nextLine(); question = triviaIn.nextLine(); numAnswers = triviaIn.nextInt(); skip = triviaIn.nextLine(); tq = new TriviaQuestion(category, question, numAnswers); for (int j = 1; j <= numAnswers; j++) { answer = triviaIn.nextLine(); tq.storeAnswer(answer); } game.insertQuestion(tq); } return game; } }
...............
public interface StringLogInterface { void insert(String element); // Precondition: This StringLog is not full. // // Places element into this StringLog.
boolean isFull(); // Returns true if this StringLog is full, otherwise returns false.
int size(); // Returns the number of Strings in this StringLog.
boolean contains(String element); // Returns true if element is in this StringLog, // otherwise returns false. // Ignores case differences when doing string comparison.
void clear(); // Makes this StringLog empty.
String getName(); // Returns the name of this StringLog.
String toString(); // Returns a nicely formatted string representing this StringLog. }
.............
public class ArrayStringLog implements StringLogInterface { protected String name; // name of this StringLog protected String[] log; // array that holds strings protected int lastIndex = -1; // index of last string in array
public ArrayStringLog(String name, int maxSize) // Precondition: maxSize > 0 // // Instantiates and returns a reference to an empty ArrayStringLog // object with name "name" and room for maxSize strings. { log = new String[maxSize]; this.name = name; }
public ArrayStringLog(String name) // Instantiates and returns a reference to an empty ArrayStringLog // object with name "name" and room for 100 strings. { log = new String[100]; this.name = name; }
public void insert(String element) // Precondition: This StringLog is not full. // // Places element into this StringLog. { lastIndex++; log[lastIndex] = element; }
public boolean isFull() // Returns true if this StringLog is full, otherwise returns false. { if (lastIndex == (log.length - 1)) return true; else return false; }
public int size() // Returns the number of Strings in this StringLog. { return (lastIndex + 1); }
public boolean contains(String element) // Returns true if element is in this StringLog, // otherwise returns false. // Ignores case differences when doing string comparison. { int location = 0; while (location <= lastIndex) { if (element.equalsIgnoreCase(log[location])) // if they match return true; else location++; } return false; }
public void clear() // Makes this StringLog empty. { for (int i = 0; i <= lastIndex; i++) log[i] = null; lastIndex = -1; }
public String getName() // Returns the name of this StringLog. { return name; }
public String toString() // Returns a nicely formatted string representing this StringLog. { String logString = "Log: " + name + " "; for (int i = 0; i <= lastIndex; i++) logString = logString + (i+1) + ". " + log[i] + " "; return logString; } }
............
import java.io.*; import java.util.Scanner;
public class Exercise2 { public static void main(String[] args) throws IOException { Scanner conIn = new Scanner(System.in); TriviaGame game; // the trivia game int questNum; // current question number TriviaQuestion tq; // current question String answer; // answer provided by user
// Initialze the game game = GetTriviaGame.useTextFile("game.txt"); // Greet the user. System.out.println("Welcome to the " + game.getQuizName() + " trivia quiz."); System.out.print("You will have " + game.getNumChances() + " chances "); System.out.println("to answer " + game.getCurrNumQuestions() + " questions. ");
questNum = 0; while (!game.isOver()) { // Get number of next unanswered question. do { if (questNum == game.getCurrNumQuestions()) questNum = 1; else questNum = questNum + 1; } while (game.isAnswered(questNum)); // Ask question and handle user's response. tq = game.getTriviaQuestion(questNum); System.out.println(tq.getCategory() + ": " + tq.getQuestion()); answer = conIn.nextLine(); if (tq.tryAnswer(answer)) { System.out.println("Correct! "); game.correctAnswer(questNum); } else { System.out.println("Incorrect "); game.incorrectAnswer(); } game.recordAttempt(questNum); }
System.out.println(" Game Over"); System.out.println(" Results:"); System.out.print(" Chances used: " + (game.getNumChances() - game.getRemainingChances())); System.out.println(" Number Correct: " + game.getNumCorrect()); System.out.println(" Thank you. "); } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
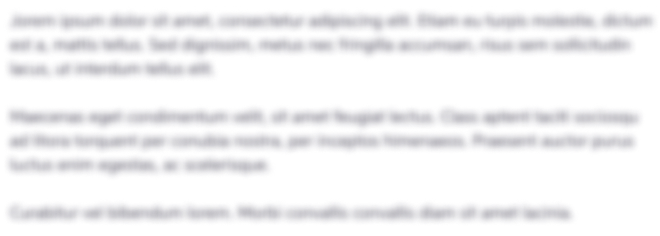
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started