Answered step by step
Verified Expert Solution
Question
1 Approved Answer
A class, PizzaPrice, is provided on the Netbeans project in the package vut.data.Q1. The class stores the name of a pizza and 3 prices,






A class, PizzaPrice, is provided on the Netbeans project in the package vut.data.Q1. The class stores the name of a pizza and 3 prices, the price of a small pizza, the price of a medium sized pizza and the price of a large pizza. The class has a constructor that passes as parameters the name and the price of a small pizza only. The price of a medium pizza is 60% more than the price of a small pizza and the price of a large pizza is 90% more than the price of a small pizza. Code all the mutator methods to only accept/assign a price when it is greater than zero. Code the setMediumPPrice method to calculate and assign the price of a medium pizza accordingly. Code the setLarge PPrice method to calculate and assign the price of a large pizza accordingly. The setMediumPPrice and setLarge PPrice must round off the price to 2 decimal places before assigning it to the instance variables (use the Math.round() method to round off the price. 2 Use the JUnit testing Framework, to verify if your setMediumPPrice and setLargePPrice methods are functioning correctly. Code the following methods: Code a test method testSetMediumPPrice 1750 that create an object using the parameter values Four Seasons and 17.50. Call the set Medium PPrice() mutator code the assertEquals() method to check if the prize of a medium pizza is calculated and assigned to the instance variable correctly. Code a test method testSet Medium PPrice Neg1750 that create an object using parameter values Four Seasons and 17.50. Call the setMediumPPrice () mutator with negative small pizza price o code the assertEquals() method to check if the price of a medium pizza will be calculated and assigned to the instance variable. Code a test method testSet Large PPrice 1750 that create an object using the parameter values Four Seasons and 17.50). Call the setLargePPrice () mutator code the assertEquals() method to check if the price of a large pizza is calculated and assigned to the instance variable correctly. O 3 A 3-tier design application with Problem domain, Data Access and GUI classes is required that allows the user to view and manipulate information about Aloe Vera products stored in a MySQL database by clicking on various buttons. Follow the following steps to create the application: 1. Create tblProducts in the database Test1ProductsDB using the product script provided in the NetBeans Project. The table should look like the one shown in Figure 1 ProdCode | ProdName 1001 *Aloe Vera Gel 1002 1003 1004 1005 Forever Garcinia Plus *Aloe Berry Nectar Forever Beta Care Forever Bee Pollen 1006 Forever Bee Propolis 1007 | *Aloe Blossom 1008 | Forever Lite Vanilla ProdCategory | No_In_Stock | Price | WEIGHT MANAGEMENT | WEIGHT MANAGEMENT | NUTRITIONAL_SUPPLEMENT | NUTRITIONAL_SUPPLEMENT | NUTRITIONAL_SUPPLEMENT | NUTRITIONAL_SUPPLEMENT | NUTRITIONAL_SUPPLEMENT WEIGHT_MANAGEMENT 0 19 21 | 33 2 32 6 86 388 226 360 300 | 326 96 426 Figure 1 2. Coding the ProductPD class The class declares the name, code, price, quantity and the category of the product as attributes. The category is stored as an enum type. The class is partly done and is stored in the vut.data package. Check if all the attributes, getters and setters have been declared correctly and if not, code the missing parts of the dass to completion. Also code the toString method to display as in Figure 4. Include two overloaded constructors: o The first constructor should pass as parameters the product name, category, quantity, and price in that order. This constructor will be used to add user input to the database. o The second constructor should pass the product code, product name, category, quantity, and price in that order. This constructor will be used to store data from the database. 4 LO 5 3. Coding the ProductDA class Code the following methods in the DA class: initialise()- the method will be used to connect to the java application to the database. viewAll()- the method will be used to access and return all the rows of tblProducts. viewOutofStockItems() - the method will be used to access and return all the columns of the products that are depleted. replenish (quantity, product code)- the method passes as parameters the quantity and the product code. The method will be used to replenish any product of a given code by a given quantity. addProduct (prod Obj)- the methodpasses as a parameter an object of the productPD class. It will be used to add a new product to tblProducts. Be sure to call these methods in the PD class. 4. GUI - Product Management Form Class The application consists of the GUI shown in Figure 2 Product Sale Application Prod Code: Prod Name: Prod Price: Quantity: Stock Value: Add Product Product Category WEIGHT MANAGEMENT NUTRITIONAL SUPPLEMENT Previous Next Replenish Out of Stock Add Product Clear Form Process 0 Figure 2 5 6 Some class level variables have been declared: The class level array list items will be used to store objects returned by the viewAll method of the DA class. The integer k will be used as an index to access the objects of the items array list. 5. Code the Product ManagementForm class as follows: Be sure the radio buttons are grouped. Hide the AddProduct panel when this form is first loaded The connection to the database should be established when the form first loads. The displayOnTextFields() method will be used to display in the text fields, the products returned by the viewAll as in Figure 3. Code it as follows: . O Assign the data returned by the viewAll method onto the items arraylist Make use of the class level integer k to display the data in the text fields as shown in Figure 3 The first product in the array list must be displayed in the text fields when the form first loads, so call the display On TextFields method in the constructor of the form. Prod Code: Prod Name: Prod Price: Quantity: Stock Value: . O 1002 Forever Garcinia Plus $388.00 19 $7,372.00 Previous Next Replenish Out of Stock Add Product Figure 3 Button Next- when clicked, the button increases the value of the integer k by 1 if this increment will not go beyond the maximum index of the array list. So it checks, increases and displays the next product of the items array list in the text fields. Otherwise it displays the message that the end of the array list has been reached. Button Previous- The button displays the products in the array list going backwards. That is, if the position of the product currently displayed is 4, when it is clicked again it will display position 3. So when clicked, the 6 7 . button decreases the value of the integer k by 1 if this decrement will not go beyond the lowest index of the array list. So it checks, decreases and displays the previous product of the items array list in the text fields. Otherwise it displays the message that the beginning of the array list has been reached. Button Replenish- the button prompts the user to enter the product code of the product to be replenished and the number of items or quantity to increase the product by and then calls the replenish method. Use input boxes for prompting the user to enter the product number and the quantity. Button Out of Stock - the button calls the viewOutofStockItems() method to display on the text area all the products that are depleted. Use the toString method of the PD dass to display as in Figure 4. Button Add Product - when clicked, the button displays the addProduct panel, clears the controls, and makes the product code and stock value text fields uneditable. Button Process the button will be used to add a new product to the database. When clicked, it checks if any of the radio buttons is selected or not, if none of the radio buttons is selected it indicates that to the user using a message box (see Figure 4) and stops processing immediately. If a radio button is selected, it assigns the text property of the radio button to a string variable prodCategory and then, Get the other input values from the controls 0 Create a product object o Call the add Product method to add the object to the database, dear the controls and Call the displayOn Textboxes method. 0 0 0 1001#*Aloe Vera Gel#86.0#0#WEIGHT_MANAGEMENT 1004#Forever Beta Care#360.0#0#NUTRITIONAL_SUPPLEMENT Button Clear- clears the controls Figure 4 ERROR indicate the category as wel X OK Figure 5 7
Step by Step Solution
There are 3 Steps involved in it
Step: 1
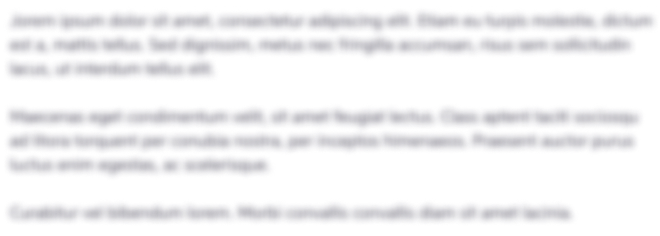
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started